How’s does async stream work
Generated on 7/30/2024
1 search
Async streams in Swift are part of the language's concurrency model, which uses the async
and await
keywords to handle asynchronous operations. Here's a detailed explanation of how async functions and streams work:
-
Task Suspension: When a task is waiting for an asynchronous operation to complete, it suspends to yield the CPU to other tasks. This is modeled in code using the
async
andawait
keywords. A function that may suspend is marked with theasync
keyword, and theawait
keyword is used to indicate that a suspension can occur on that line. This allows the CPU to be used efficiently by other tasks while waiting for the asynchronous operation to complete. (A Swift Tour: Explore Swift’s features and design) -
Memory Allocation: Async functions do not allocate memory out of a large contiguous stack like synchronous functions. Instead, they hold onto one or more slabs of memory. When an async function needs to allocate memory, it asks the task for memory from the current slab. If the slab is full, a new slab is allocated. This method is typically faster than using
malloc
because the allocator is only used by a single task and follows a stack discipline. (Explore Swift performance) -
Partial Functions: Async functions are split into multiple partial functions at runtime, each spanning the gaps between potential suspension points. For example, if there is one
await
in the function, it results in two partial functions. The first partial function runs until theawait
, and the second picks up after theawait
. This ensures that only one partial function is on the C stack at any time, optimizing memory usage and performance. (Explore Swift performance) -
Concurrency and Actors: Swift also introduces actors, which are reference types that encapsulate shared mutable state and automatically protect their state by serializing accesses. Calls to actor methods from outside the actor's context are asynchronous, ensuring safe concurrent access to shared resources. (A Swift Tour: Explore Swift’s features and design)
For a more comprehensive understanding, you can explore the following sessions:
These sessions cover the intricacies of async functions, memory allocation, and concurrency in Swift.
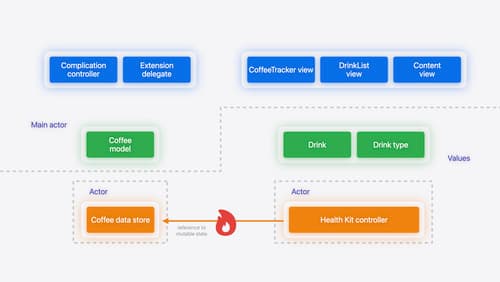
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
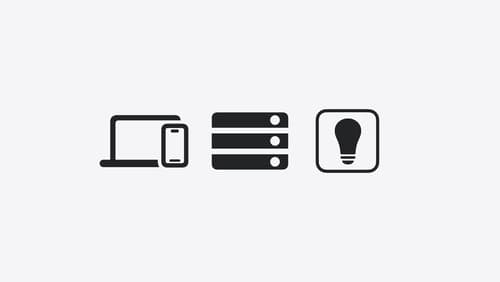
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
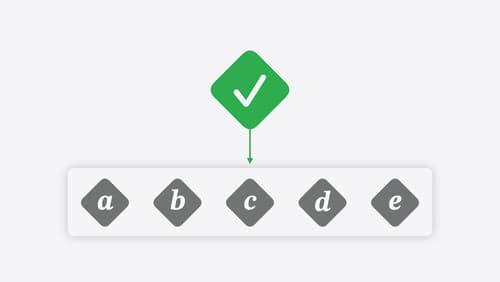
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
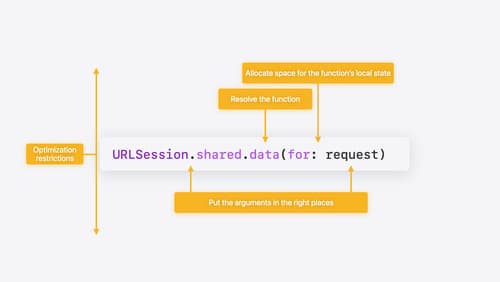
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.