How to request user location?
Generated on 7/31/2024
1 search
To request user location in your app, you can use the CoreLocation framework. Here are the steps to do this, based on the information from the WWDC session "What’s new in location authorization":
-
Request Authorization:
- Use the
CLLocationManager
to request location authorization. You can request either "when in use" or "always" authorization. - For example, to request "when in use" authorization, you can use:
let locationManager = CLLocationManager() locationManager.requestWhenInUseAuthorization()
- Use the
-
Handle Different Authorization States:
- Users can grant different levels of location access, such as full accuracy or approximate location. You can request temporary full accuracy if needed:
locationManager.requestTemporaryFullAccuracyAuthorization(withPurposeKey: "YourPurposeKey")
- Users can grant different levels of location access, such as full accuracy or approximate location. You can request temporary full accuracy if needed:
-
Check Authorization Status:
- You can check the current authorization status using the
authorizationStatus
property ofCLLocationManager
:let status = CLLocationManager.authorizationStatus() switch status { case .notDetermined: // Request authorization case .restricted, .denied: // Handle denied access case .authorizedWhenInUse, .authorizedAlways: // Access granted @unknown default: // Handle other cases }
- You can check the current authorization status using the
-
Use CL Service Sessions:
- The new
CLServiceSession
provides a declarative way to manage location authorization. It helps to define your authorization goals and handle the session lifecycle:let serviceSession = CLServiceSession() serviceSession.requestAuthorization()
- The new
For more detailed information, you can refer to the session What’s new in location authorization at the timestamp 00:57.
Relevant Sessions
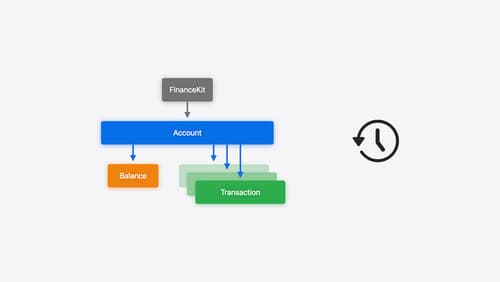
Meet FinanceKit
Learn how FinanceKit lets your financial management apps seamlessly and securely share on-device data from Apple Cash, Apple Card, and more, with user consent and control. Find out how to request one-time and ongoing access to accounts, transactions, and balances — and how to build great experiences for iOS and iPadOS.
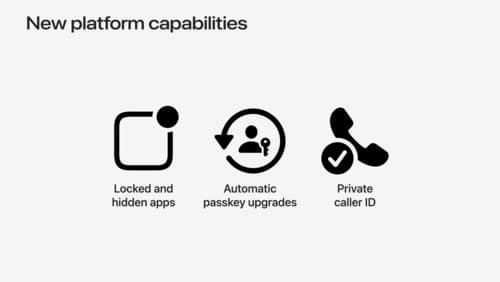
What’s new in privacy
At Apple, we believe privacy is a fundamental human right. Learn about new and improved permission flows and other features that manage data in a privacy-preserving way, so that you can focus on creating great app experiences.
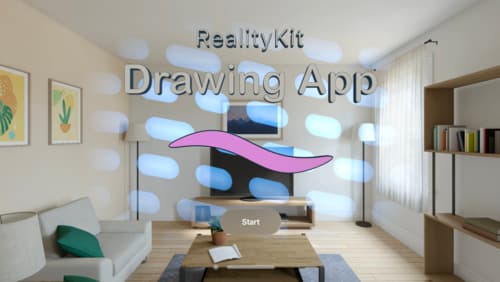
Build a spatial drawing app with RealityKit
Harness the power of RealityKit through the process of building a spatial drawing app. As you create an eye-catching spatial experience that integrates RealityKit with ARKit and SwiftUI, you’ll explore how resources work in RealityKit and how to use features like low-level mesh and texture APIs to achieve fast updates of the users’ brush strokes.
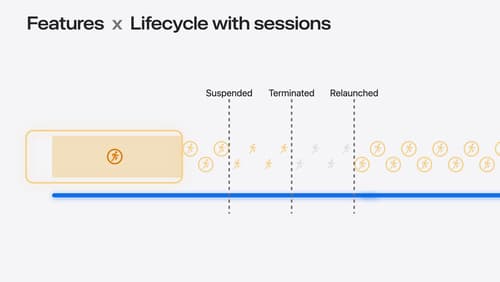
What’s new in location authorization
Location authorization is turning 2.0. Learn about new recommendations and techniques to get the authorization you need, and a new system of diagnostics that can let you know when an authorization goal can’t be met.