how do I create a metal rendering context
Generated on 7/31/2024
1 search
To create a Metal rendering context, you can follow these general steps:
-
Set Up Your Project: Ensure your project is configured to use Metal. This involves setting up the necessary frameworks and creating a Metal device.
-
Create a Metal Layer: You can start with a
CAMetalLayer
if it is easier. This layer will be used to display your rendered content. -
Create a Metal Device: Obtain a reference to the Metal device, which represents the GPU.
-
Create a Command Queue: This queue will manage the execution of command buffers.
-
Create a Render Pipeline: Set up a render pipeline descriptor and create a render pipeline state object.
-
Create a Drawable Texture: Create a texture to render into.
-
Render Loop: Set up a render loop to continuously render frames.
Here is a simplified example in code:
import Metal
import QuartzCore
class MetalRenderer {
var device: MTLDevice!
var commandQueue: MTLCommandQueue!
var metalLayer: CAMetalLayer!
init(view: UIView) {
// Create a Metal device
device = MTLCreateSystemDefaultDevice()
// Create a command queue
commandQueue = device.makeCommandQueue()
// Create a Metal layer
metalLayer = CAMetalLayer()
metalLayer.device = device
metalLayer.pixelFormat = .bgra8Unorm
metalLayer.framebufferOnly = true
metalLayer.frame = view.layer.frame
view.layer.addSublayer(metalLayer)
}
func render() {
guard let drawable = metalLayer?.nextDrawable() else { return }
let renderPassDescriptor = MTLRenderPassDescriptor()
renderPassDescriptor.colorAttachments[0].texture = drawable.texture
renderPassDescriptor.colorAttachments[0].loadAction = .clear
renderPassDescriptor.colorAttachments[0].clearColor = MTLClearColor(red: 0, green: 0, blue: 0, alpha: 1)
renderPassDescriptor.colorAttachments[0].storeAction = .store
let commandBuffer = commandQueue.makeCommandBuffer()
let renderEncoder = commandBuffer?.makeRenderCommandEncoder(descriptor: renderPassDescriptor)
renderEncoder?.endEncoding()
commandBuffer?.present(drawable)
commandBuffer?.commit()
}
}
For more detailed information and practical examples, you can refer to the following sessions from WWDC 2024:
- Port advanced games to Apple platforms (Shaders)
- Render Metal with passthrough in visionOS (Introduction)
These sessions provide comprehensive guidelines and code samples to help you get started with Metal rendering.
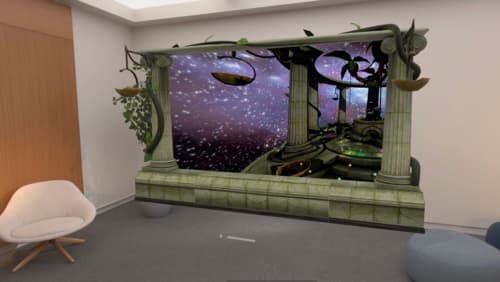
Bring your iOS or iPadOS game to visionOS
Discover how to transform your iOS or iPadOS game into a uniquely visionOS experience. Increase the immersion (and fun factor!) with a 3D frame or an immersive background. And invite players further into your world by adding depth to the window with stereoscopy or head tracking.
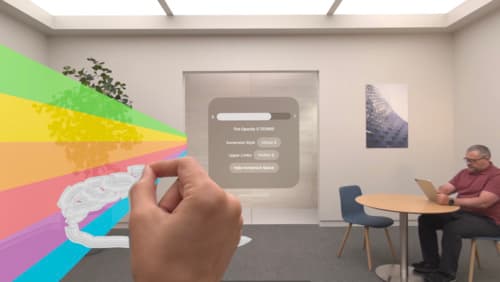
Render Metal with passthrough in visionOS
Get ready to extend your Metal experiences for visionOS. Learn best practices for integrating your rendered content with people’s physical environments with passthrough. Find out how to position rendered content to match the physical world, reduce latency with trackable anchor prediction, and more.
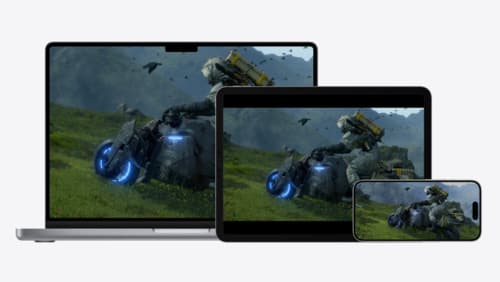
Port advanced games to Apple platforms
Discover how simple it can be to reach players on Apple platforms worldwide. We’ll show you how to evaluate your Windows executable on Apple silicon, start your game port with code samples, convert your shader code to Metal, and bring your game to Mac, iPhone, and iPad. Explore enhanced Metal tools that understand HLSL shaders to validate, debug, and profile your ported shaders on Metal.