how do I prevent my audio streaming app from pausing audio playback in the background when other audio app is opened?
Asked on 07/31/2024
1 search
To prevent your audio streaming app from pausing audio playback in the background when another audio app is opened, you need to manage audio session interruptions and configure your app's audio session properly. This is not directly covered in the provided WWDC segments, but here are some general steps you can follow:
-
Configure Audio Session Category: Set your app's audio session category to
AVAudioSessionCategoryPlayback
. This category is designed for apps that play audio in the background.do { try AVAudioSession.sharedInstance().setCategory(.playback, mode: .default, options: []) try AVAudioSession.sharedInstance().setActive(true) } catch { print("Failed to set audio session category: \(error)") }
-
Handle Audio Session Interruptions: Implement handlers for audio session interruptions to manage what happens when your app's audio is interrupted by another app.
NotificationCenter.default.addObserver(self, selector: #selector(handleInterruption), name: AVAudioSession.interruptionNotification, object: AVAudioSession.sharedInstance()) @objc func handleInterruption(notification: Notification) { guard let userInfo = notification.userInfo, let typeValue = userInfo[AVAudioSessionInterruptionTypeKey] as? UInt, let type = AVAudioSession.InterruptionType(rawValue: typeValue) else { return } switch type { case .began: // Audio has been interrupted // Pause or stop your audio playback break case .ended: // Interruption ended // Resume your audio playback if needed break default: break } }
-
Background Modes: Ensure that your app has the appropriate background modes enabled in the app's capabilities. Specifically, enable the "Audio, AirPlay, and Picture in Picture" background mode.
-
Mixing with Other Audio: If you want your app to mix its audio with other apps' audio, you can set the
AVAudioSessionCategoryOptionMixWithOthers
option.do { try AVAudioSession.sharedInstance().setCategory(.playback, mode: .default, options: [.mixWithOthers]) try AVAudioSession.sharedInstance().setActive(true) } catch { print("Failed to set audio session category: \(error)") }
For more detailed information on handling audio in your app, you might want to check out sessions related to audio management from WWDC, such as "Enhance your spatial computing app with RealityKit audio" which covers various aspects of audio playback and configuration.
Relevant Sessions
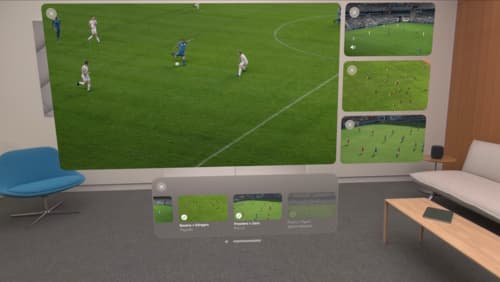
Explore multiview video playback in visionOS
Learn how AVExperienceController can enable playback of multiple videos on Apple Vision Pro. Review best practices for adoption and explore great use cases, like viewing a sports broadcast from different angles or watching multiple games simultaneously. And discover how to design a compelling and intuitive multiview experience in your app.
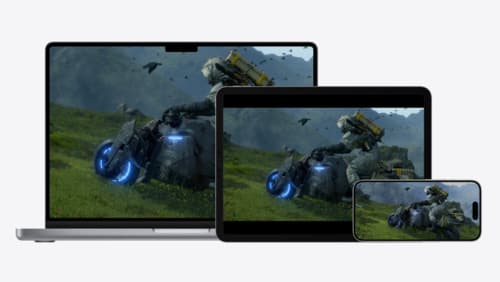
Port advanced games to Apple platforms
Discover how simple it can be to reach players on Apple platforms worldwide. We’ll show you how to evaluate your Windows executable on Apple silicon, start your game port with code samples, convert your shader code to Metal, and bring your game to Mac, iPhone, and iPad. Explore enhanced Metal tools that understand HLSL shaders to validate, debug, and profile your ported shaders on Metal.
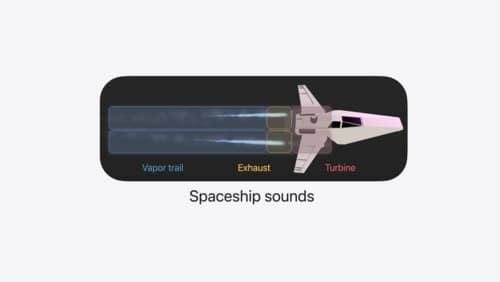
Enhance your spatial computing app with RealityKit audio
Elevate your spatial computing experience using RealityKit audio. Discover how spatial audio can make your 3D immersive experiences come to life. From ambient audio, reverb, to real-time procedural audio that can add character to your 3D content, learn how RealityKit audio APIs can help make your app more engaging.