How to write data to swiftdata
Generated on 7/31/2024
1 search
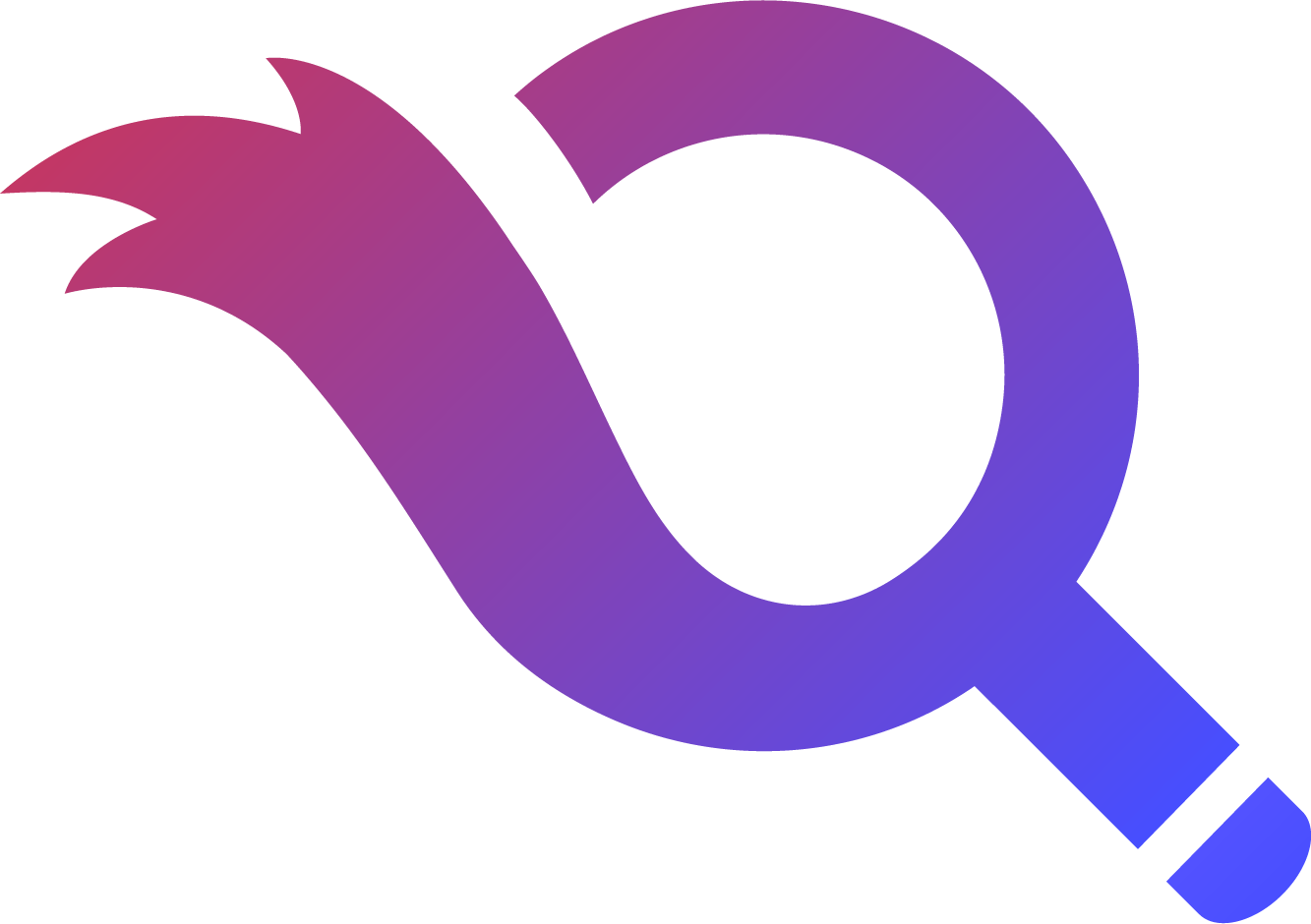
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To write data using SwiftData, you can follow these steps:
-
Import the SwiftData Framework: First, ensure that you have imported the SwiftData framework in your Swift file.
-
Define Your Model: Use the
@Model
macro to define your data model. This macro helps in creating the schema for your data. -
Create a Model Container: Use the
ModelContainer
to manage your data models. You can customize the container to use different storage backends if needed. -
Write Data: Use the model context to write data to your store.
Here is a simple example to illustrate these steps:
Step-by-Step Example
-
Import the Framework:
import SwiftData
-
Define Your Model:
@Model class Trip { var destination: String var date: Date init(destination: String, date: Date) { self.destination = destination self.date = date } }
-
Create a Model Container:
let container = ModelContainer([Trip.self])
-
Write Data:
let context = container.viewContext let newTrip = Trip(destination: "Paris", date: Date()) context.insert(newTrip) do { try context.save() } catch { print("Failed to save trip: \(error)") }
Additional Information
-
Custom Data Stores: If you need to use a custom data store, you can configure it using the
Datastore
protocol. This allows you to use different backends like JSON files, SQLite, or even remote web services. For more details, you can refer to the session Create a custom data store with SwiftData. -
Model Container Customization: You can further customize your model container to fit your needs. For example, you can set up a custom URL for your data on disk. For more details, you can refer to the session What’s new in SwiftData.
Relevant Sessions
These sessions provide a comprehensive overview and deeper insights into using SwiftData effectively in your applications.
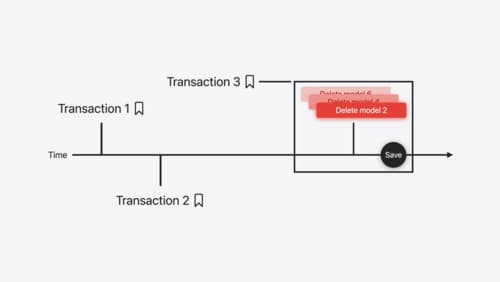
Track model changes with SwiftData history
Reveal the history of your model’s changes with SwiftData! Use the history API to understand when data store changes occurred, and learn how to use this information to build features like remote server sync and out-of-process change handing in your app. We’ll also cover how you can build support for the history API into a custom data store.
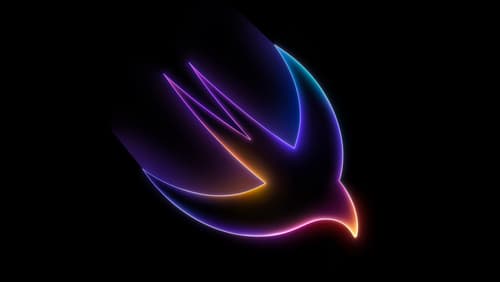
Platforms State of the Union
Discover the newest advancements on Apple platforms.
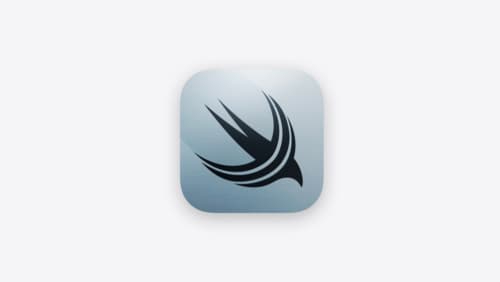
What’s new in SwiftData
SwiftData makes it easy to add persistence to your app with its expressive, declarative API. Learn about refinements to SwiftData, including compound uniqueness constraints, faster queries with #Index, queries in Xcode previews, and rich predicate expressions. Join us to explore how you can use all of these features to express richer models and improve performance in your app. To discover how to build a custom data store or use the history API in SwiftData, watch “Create a custom data store with SwiftData” and “Track model changes with SwiftData history”.
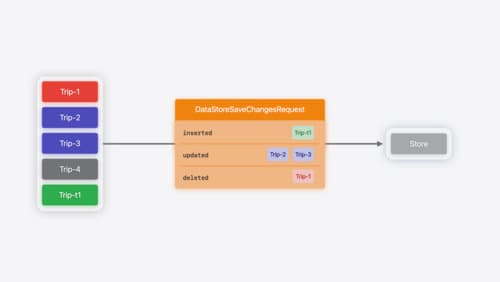
Create a custom data store with SwiftData
Combine the power of SwiftData’s expressive, declarative modeling API with your own persistence backend. Learn how to build a custom data store and explore how to progressively add persistence features in your app. To get the most out of this session, watch “Meet SwiftData” and “Model your schema with SwiftData” from WWDC23.