How do I implement picture-in-picture in AVPlayerViewController?
Generated on 7/31/2024
1 search
To implement Picture-in-Picture (PiP) in AVPlayerViewController
, you can leverage the AVKit
framework, which provides built-in support for PiP. Here are the steps to enable and use PiP in your app:
-
Enable PiP in
AVPlayerViewController
: Ensure that theallowsPictureInPicturePlayback
property of yourAVPlayerViewController
instance is set totrue
.let playerViewController = AVPlayerViewController() playerViewController.allowsPictureInPicturePlayback = true
-
Configure the
AVPlayer
: Set up yourAVPlayer
with the desired media content and assign it to theplayer
property of yourAVPlayerViewController
.let player = AVPlayer(url: videoURL) playerViewController.player = player
-
Present the
AVPlayerViewController
: Present theAVPlayerViewController
in your app's view hierarchy.present(playerViewController, animated: true) { player.play() }
-
Handle PiP Delegate Methods (Optional): Implement the
AVPlayerViewControllerDelegate
methods to handle PiP events, such as when PiP starts or stops.class ViewController: UIViewController, AVPlayerViewControllerDelegate { func playerViewControllerWillStartPictureInPicture(_ playerViewController: AVPlayerViewController) { // Handle PiP start } func playerViewControllerDidStopPictureInPicture(_ playerViewController: AVPlayerViewController) { // Handle PiP stop } func playerViewController(_ playerViewController: AVPlayerViewController, failedToStartPictureInPictureWithError error: Error) { // Handle PiP start failure } }
-
Set the Delegate: Assign the delegate to your
AVPlayerViewController
instance.playerViewController.delegate = self
For more detailed information on using AVPlayerViewController
and its capabilities, you can refer to the session Explore multiview video playback in visionOS from WWDC 2024. This session covers various aspects of AVPlayerViewController
, including its integration with system features and enhancements for video viewing capabilities.
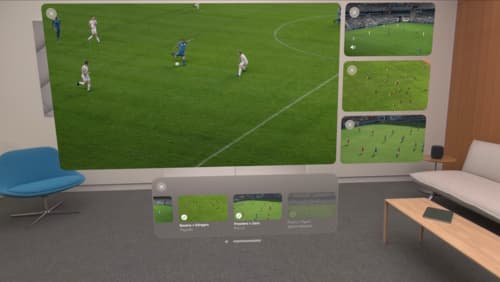
Explore multiview video playback in visionOS
Learn how AVExperienceController can enable playback of multiple videos on Apple Vision Pro. Review best practices for adoption and explore great use cases, like viewing a sports broadcast from different angles or watching multiple games simultaneously. And discover how to design a compelling and intuitive multiview experience in your app.
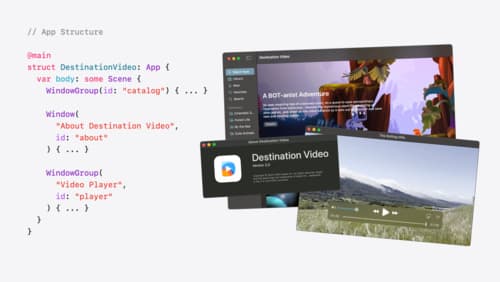
Tailor macOS windows with SwiftUI
Make your windows feel tailor-made for macOS. Fine-tune your app’s windows for focused purposes, ease of use, and to express functionality. Use SwiftUI to style window toolbars and backgrounds. Arrange your windows with precision, and make smart decisions about restoration and minimization.
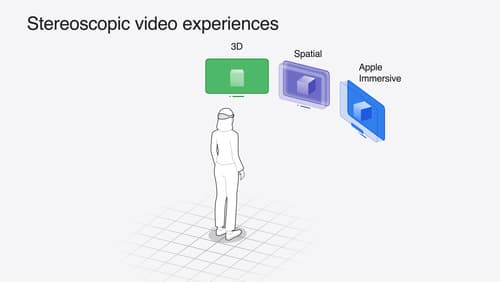
Build compelling spatial photo and video experiences
Learn how to adopt spatial photos and videos in your apps. Explore the different types of stereoscopic media and find out how to capture spatial videos in your iOS app on iPhone 15 Pro. Discover the various ways to detect and present spatial media, including the new QuickLook Preview Application API in visionOS. And take a deep dive into the metadata and stereo concepts that make a photo or video spatial.