How to embed a tab bar controller inside a navigation controller?
Asked on 07/31/2024
1 search
To embed a tab bar controller inside a navigation controller, you can follow these steps:
-
Create a
UITabBarController
instance: This will be the main controller that holds your tab bar. -
Create instances of the view controllers you want to include in the tab bar: These view controllers will be the individual tabs.
-
Embed each view controller in a
UINavigationController
: This allows each tab to have its own navigation stack. -
Set the view controllers of the
UITabBarController
: Assign the array of navigation controllers to the tab bar controller.
Here is a sample code snippet in Swift:
import UIKit
class FirstViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
self.view.backgroundColor = .red
self.title = "First"
}
}
class SecondViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
self.view.backgroundColor = .blue
self.title = "Second"
}
}
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
window = UIWindow(frame: UIScreen.main.bounds)
let firstVC = FirstViewController()
let secondVC = SecondViewController()
let firstNavController = UINavigationController(rootViewController: firstVC)
let secondNavController = UINavigationController(rootViewController: secondVC)
let tabBarController = UITabBarController()
tabBarController.viewControllers = [firstNavController, secondNavController]
window?.rootViewController = tabBarController
window?.makeKeyAndVisible()
return true
}
}
In this example:
FirstViewController
andSecondViewController
are the view controllers that will be displayed in the tabs.- Each view controller is embedded in a
UINavigationController
. - These navigation controllers are then assigned to the
viewControllers
property of theUITabBarController
.
For more detailed information on how to enable the sidebar with UITabBarController
in UIKit, you can refer to the session Elevate your tab and sidebar experience in iPadOS.
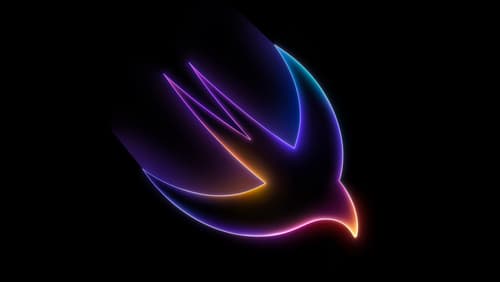
Platforms State of the Union
Discover the newest advancements on Apple platforms.
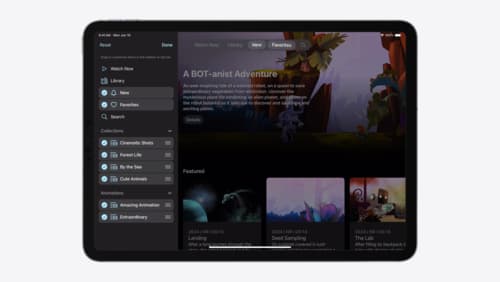
Elevate your tab and sidebar experience in iPadOS
iPadOS 18 introduces a new navigation system that gives people the flexibility to choose between using a tab bar or sidebar. The newly redesigned tab bar provides more space for content and other functionality. Learn how to use SwiftUI and UIKit to enable customization features – like adding, removing and reordering tabs – to enable a more personal touch in your app.