How would I create streaming speech to text recognition?
Generated on 7/31/2024
1 search
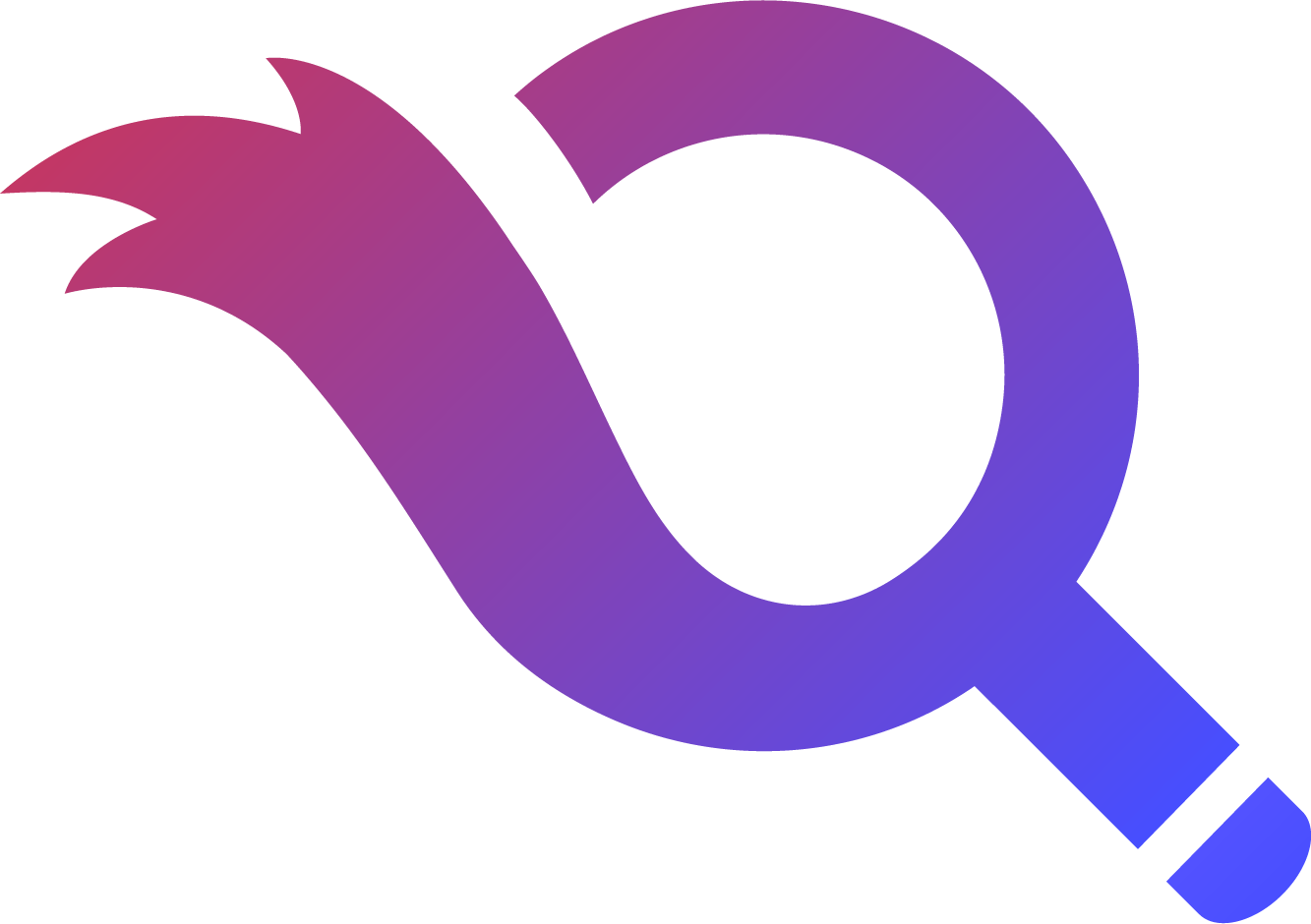
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To create streaming speech-to-text recognition, you can use the Web Speech API, which is a proposed web standard that allows you to respond to voice in real-time through the speech recognition interface. Here's a step-by-step guide based on the content from the session "Optimize for the spatial web":
-
Create a Speech Recognition Object:
const recognition = new webkitSpeechRecognition(); recognition.continuous = true; // For continuous recognition recognition.interimResults = true; // To get interim results
-
Register a Handler to Listen for Result Events:
recognition.onresult = (event) => { const results = event.results; const transcript = results[results.length - 1][0].transcript; console.log(transcript); // Use the transcript as needed };
-
Start the Recognizer on a User Event:
document.querySelector('button').addEventListener('click', () => { recognition.start(); });
-
Handle Permissions: Ensure that the user is aware of why microphone input is needed, as there will be a permission prompt.
-
Speech Synthesis for Feedback: You can also use the Speech Synthesis API to provide audio feedback:
const utterance = new SpeechSynthesisUtterance('Your final score is...'); speechSynthesis.speak(utterance);
For more detailed information, you can refer to the session Optimize for the spatial web.
Additionally, if you are looking to implement more advanced speech-to-text recognition using machine learning, you can leverage the Metal Performance Shaders (MPS) for fast Fourier transforms and transformer models as described in the session "Accelerate machine learning with Metal". This involves using a short-time Fourier transform (STFT) and a transformer model to extract text from an audio signal. For more details, you can refer to the session Accelerate machine learning with Metal.
Relevant Sessions:
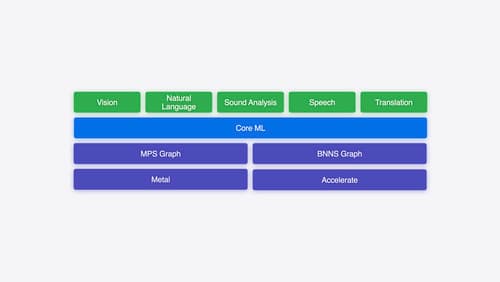
Explore machine learning on Apple platforms
Get started with an overview of machine learning frameworks on Apple platforms. Whether you’re implementing your first ML model, or an ML expert, we’ll offer guidance to help you select the right framework for your app’s needs.
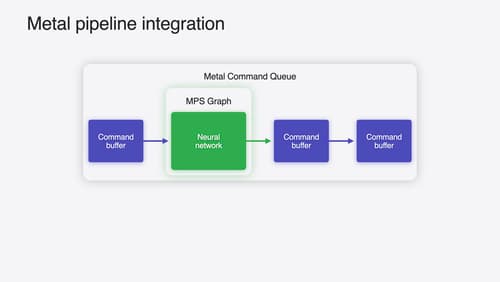
Accelerate machine learning with Metal
Learn how to accelerate your machine learning transformer models with new features in Metal Performance Shaders Graph. We’ll also cover how to improve your model’s compute bandwidth and quality, and visualize it in the all new MPSGraph viewer.
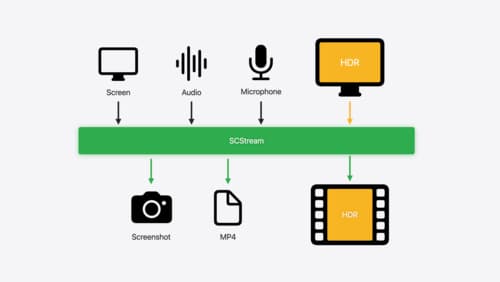
Capture HDR content with ScreenCaptureKit
Learn how to capture high dynamic colors using ScreenCaptureKit, and explore new features like HDR support, microphone capture, and straight-to-file recording.
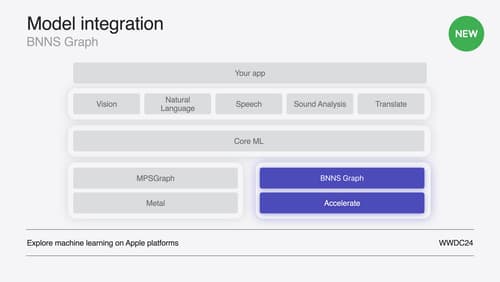
Support real-time ML inference on the CPU
Discover how you can use BNNSGraph to accelerate the execution of your machine learning model on the CPU. We will show you how to use BNNSGraph to compile and execute a machine learning model on the CPU and share how it provides real-time guarantees such as no runtime memory allocation and single-threaded running for audio or signal processing models.
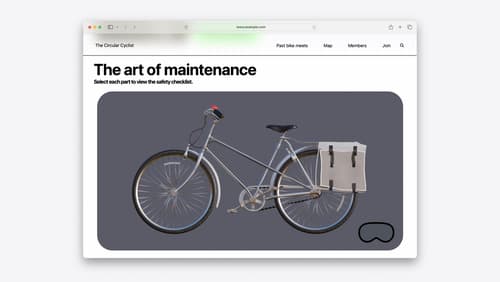
Optimize for the spatial web
Discover how to make the most of visionOS capabilities on the web. Explore recent updates like improvements to selection highlighting, and the ability to present spatial photos and panorama images in fullscreen. Learn to take advantage of existing web standards for dictation and text-to-speech with WebSpeech, spatial soundscapes with WebAudio, and immersive experiences with WebXR.