How can I create a SwiftUI App?
Asked on 07/31/2024
1 search
To create a SwiftUI app, you can follow these steps:
-
Launch Xcode: Open Xcode, Apple's integrated development environment (IDE).
-
Create a New Project: Select "Create a new Xcode project" and choose the "App" template under the iOS, macOS, watchOS, or tvOS section, depending on your target platform.
-
Set Up Your Project: Enter your project name, organization identifier, and other settings. Make sure to select "SwiftUI" for the User Interface option.
-
Understand SwiftUI Basics: SwiftUI is a declarative framework, meaning you describe what your UI should look like and SwiftUI takes care of rendering it. Views are the basic building blocks in SwiftUI. They are declarative, compositional, and state-driven.
- Declarative: You describe what view you want in your user interface, and SwiftUI produces the result.
- Compositional: You can combine smaller views to create more complex ones.
- State-Driven: Views automatically update when the state changes.
-
Build Your First View: Start by creating a simple view. For example, you can create a
Text
view inside aVStack
(vertical stack) to display text vertically.import SwiftUI struct ContentView: View { var body: some View { VStack { Text("Hello, SwiftUI!") .font(.largeTitle) .padding() Text("Welcome to your first SwiftUI app.") .font(.subheadline) } } } struct ContentView_Previews: PreviewProvider { static var previews: some View { ContentView() } }
-
Run Your App: Click the "Run" button in Xcode to build and run your app on the simulator or a connected device.
For more detailed guidance, you can watch the SwiftUI essentials session from WWDC 2024, which covers the fundamentals of views, built-in capabilities, and how to use SwiftUI across all Apple platforms.
Relevant Sessions
These sessions provide a comprehensive overview of SwiftUI and its capabilities, helping you get started with building your SwiftUI app.
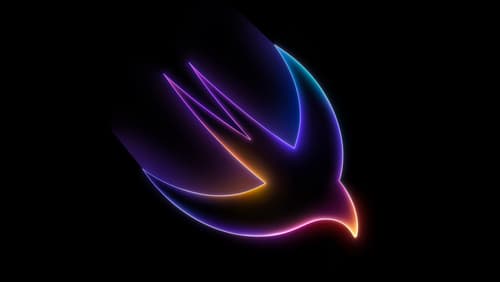
Platforms State of the Union
Discover the newest advancements on Apple platforms.
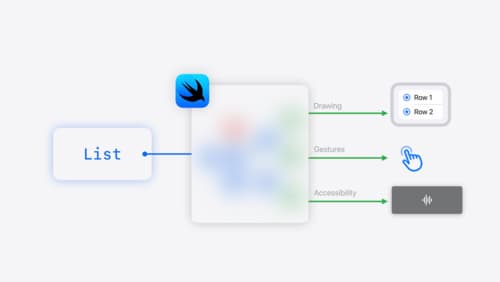
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
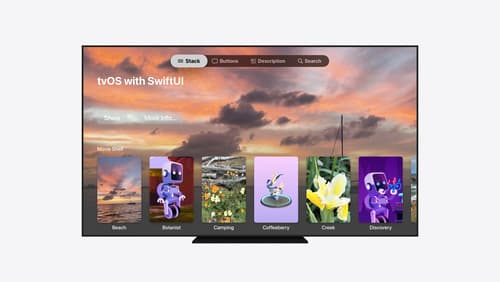
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.