How do I use async functions?
Asked on 07/31/2024
1 search
To use async functions in Swift, you need to understand a few key concepts and syntax. Here are the main points:
-
Marking Functions as Async: A function that may suspend is marked with the
async
keyword. This indicates that the function can perform asynchronous operations. -
Using Await: When calling an async function, you use the
await
keyword to indicate that a suspension can occur at that line. This allows the function to yield control back to the system while waiting for the asynchronous operation to complete. -
Task Suspension: When an async function is waiting for an operation to complete, it suspends to yield the CPU to other tasks. This is modeled in code using the
async
andawait
keywords. -
Memory Allocation: Async functions allocate their local state on a separate stack from the C stack. They are split into multiple functions at runtime, with each partial function handling a segment of the async function's execution.
-
Partial Functions: An async function is split into partial functions that span the gaps between potential suspension points. Each partial function runs like an ordinary C function until the next suspension point.
Here are some relevant segments from WWDC sessions that explain these concepts in more detail:
-
Explore Swift performance:
- Async functions (24:33)
- Partial functions (26:46)
-
A Swift Tour: Explore Swift’s features and design:
- Concurrency (18:33)
-
Go further with Swift Testing:
- Asynchronous conditions (24:26)
These sessions provide a comprehensive overview of how async functions work in Swift, including their implementation, memory management, and practical usage in code.
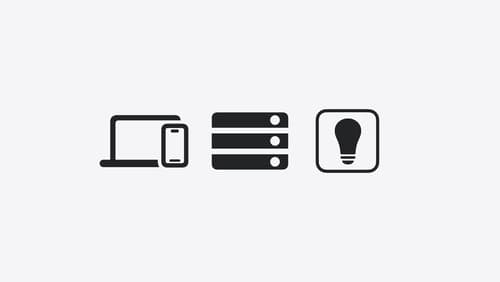
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
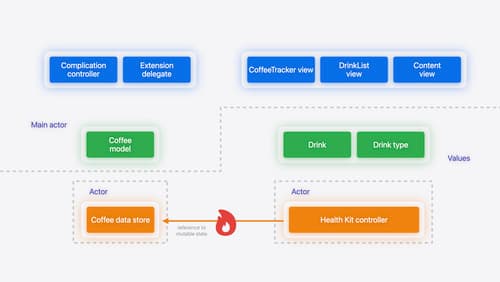
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
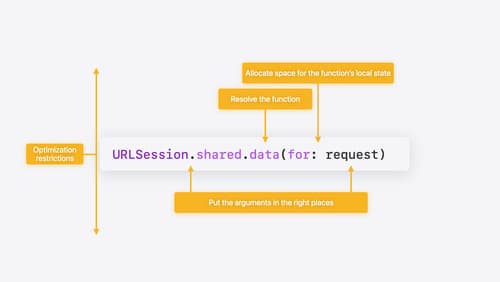
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.