is better to use structs or classes in swift
Generated on 7/31/2024
1 search
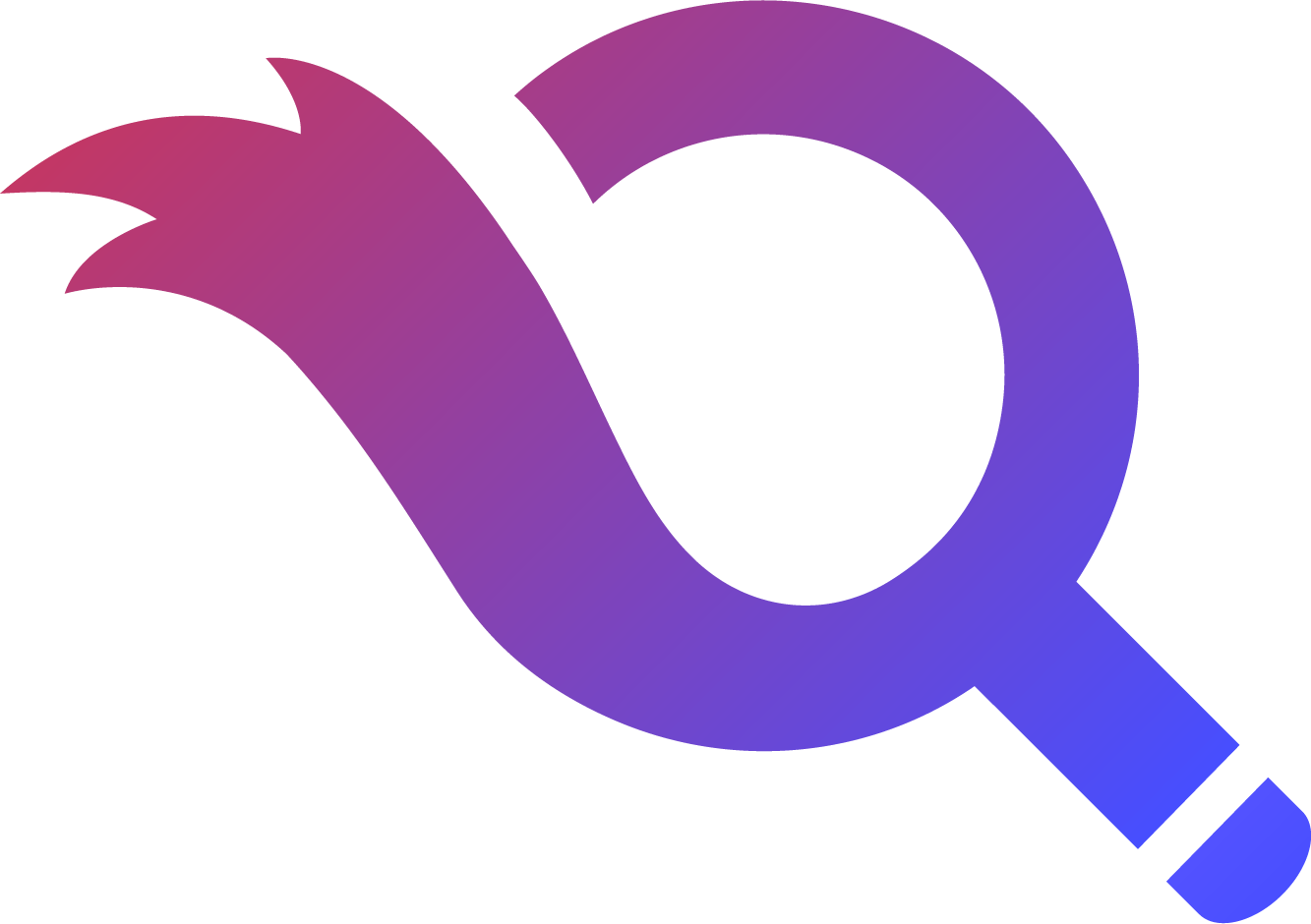
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
In Swift, the choice between using structs or classes depends on the specific requirements of your application, particularly regarding value semantics and memory management.
Structs
- Value Semantics: Structs in Swift are value types, which means that each instance keeps a unique copy of its data. When you assign or pass a struct, you get a copy of the data, making it easier to reason about state and avoid unintended side effects.
- Inline Storage: Structs use inline storage, which can be more efficient for small data types because it avoids heap allocation. However, copying large structs can be costly in terms of performance (Explore Swift performance).
- Use Cases: Structs are ideal for representing simple data structures that do not require shared mutable state. They are commonly used for models, configurations, and other data that benefits from immutability and value semantics (A Swift Tour: Explore Swift’s features and design).
Classes
- Reference Semantics: Classes are reference types, meaning that instances are shared and changes to one instance affect all references to that instance. This is useful for managing shared mutable state.
- Out of Line Storage: Classes use out of line storage, which means they store their data on the heap and are referenced by pointers. This can be more efficient for large data structures that are frequently copied, as only the reference is copied, not the data itself (Explore Swift performance).
- Automatic Reference Counting (ARC): Swift uses ARC to manage the memory of class instances, which can introduce complexity such as reference cycles that need to be managed with weak references (A Swift Tour: Explore Swift’s features and design).
- Use Cases: Classes are suitable for scenarios where you need shared mutable state, identity, or inheritance. They are often used for managing complex state, implementing design patterns like Singleton, or when you need polymorphism (A Swift Tour: Explore Swift’s features and design).
Summary
- Use Structs: When you need value semantics, immutability, and efficient memory usage for small data types.
- Use Classes: When you need reference semantics, shared mutable state, or inheritance.
For more detailed information, you can refer to the sessions:
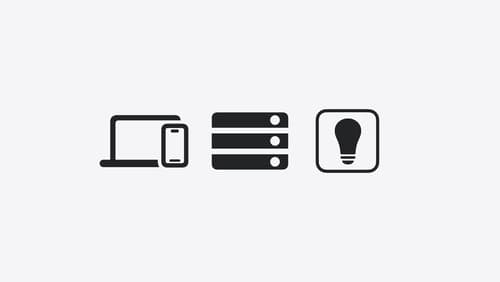
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
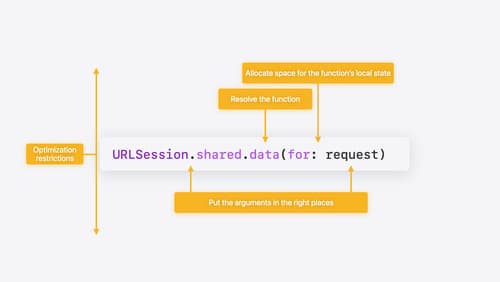
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.