do structs offer better performance than classes in swift
Generated on 7/31/2024
1 search
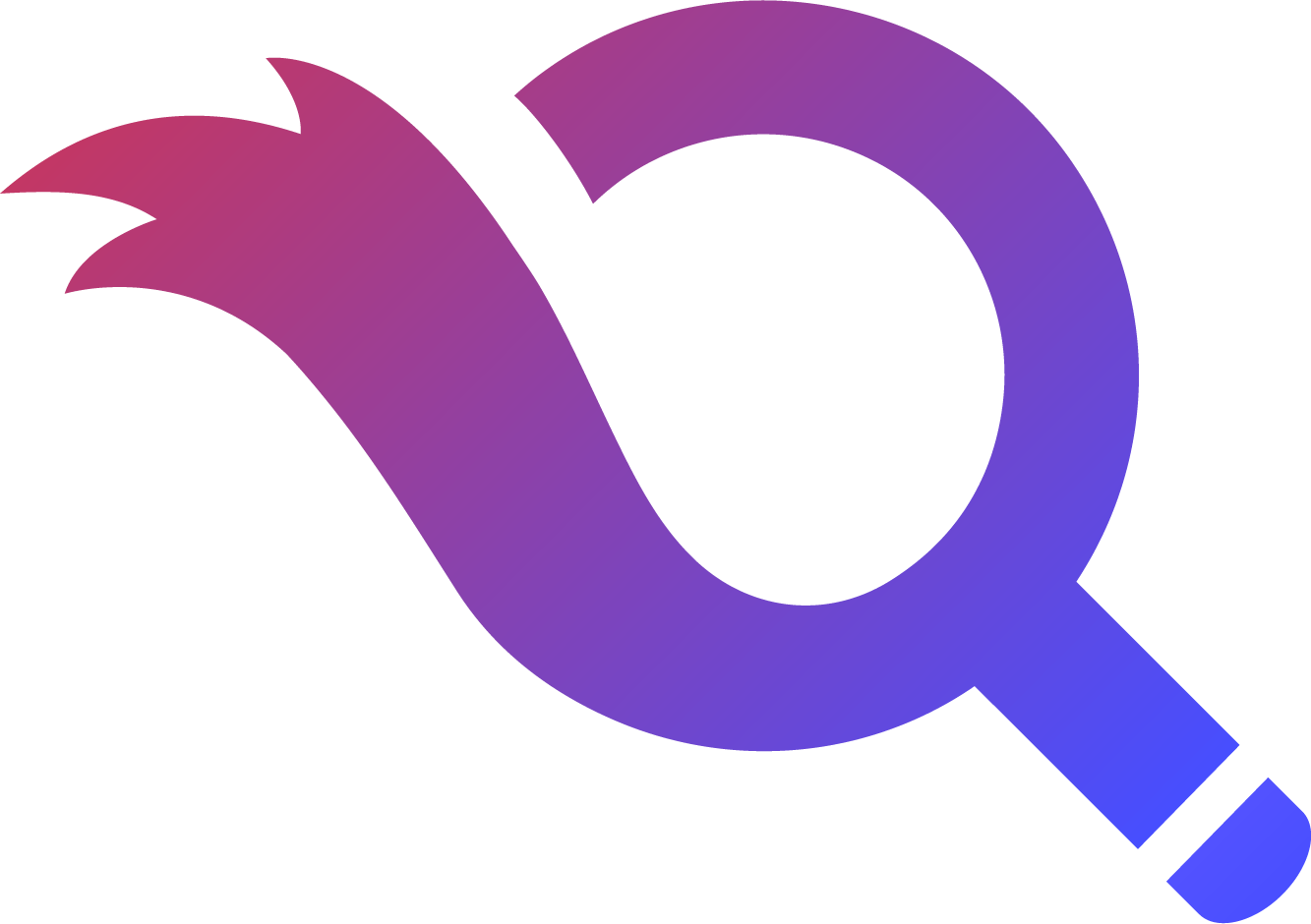
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
In Swift, structs and classes have different performance characteristics due to their underlying storage mechanisms and semantics.
Structs
- Inline Storage: Structs use inline storage, meaning their data is stored directly within the variable. This can lead to better performance for small types because it avoids heap allocations.
- Value Semantics: Structs have value semantics, meaning that when you copy a struct, you get a completely independent copy of the data. This can be beneficial for performance as it avoids the overhead of reference counting.
- Copying Costs: However, copying large structs can be expensive because it involves copying all of the stored properties. This can lead to higher memory usage if the struct is copied frequently.
Classes
- Out of Line Storage: Classes use out of line storage, meaning their data is stored on the heap, and the variable holds a reference to this data. This can be more efficient for larger types or when the data is shared across multiple instances.
- Reference Semantics: Classes have reference semantics, meaning that when you copy a class, you are just copying a reference to the same data. This can be more memory efficient and faster for large objects that are copied frequently.
- Reference Counting: Classes use automatic reference counting (ARC) to manage memory, which can introduce some overhead due to the need to increment and decrement reference counts.
Trade-offs
- Memory Allocation: Structs avoid heap allocations, which can be beneficial for small types. However, for larger types, the cost of copying can become significant.
- Copying Overhead: Classes avoid the overhead of copying large amounts of data by using references, but they incur the cost of reference counting.
Example from WWDC
In the session Explore Swift performance, it is explained that structs use inline storage and classes use out of line storage. This difference has major performance implications. For instance, copying a struct involves copying all of its stored properties, which can be costly if the struct is large or copied frequently. On the other hand, copying a class involves copying a reference, which is generally cheaper but involves reference counting overhead.
Conclusion
There is no one-size-fits-all answer to whether structs offer better performance than classes in Swift. The choice between structs and classes should be based on the specific use case, considering factors like the size of the data, the frequency of copying, and the need for value or reference semantics.
Relevant Sessions
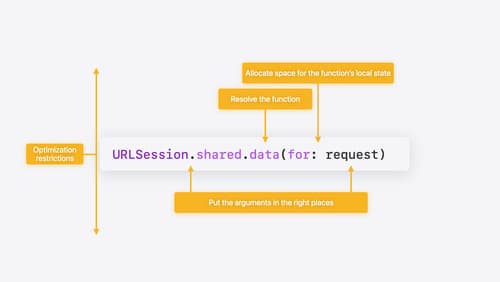
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
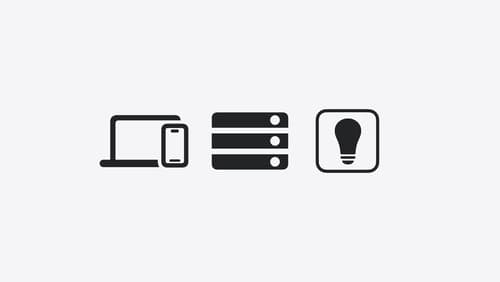
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.