how would I reimplement swipe actions for a lazyvstack?
Generated on 7/31/2024
1 search
To reimplement swipe actions for a LazyVStack
in SwiftUI, you can follow the principles discussed in the SwiftUI essentials session. Here are the steps you can take:
- Create a
LazyVStack
: This will be the container for your list of items. - Use
ForEach
to generate rows: Each row can represent an item in your list. - Add swipe actions to each row: You can use the
swipeActions
modifier to add actions to each row.
Here is a basic example:
import SwiftUI
struct ContentView: View {
@State private var items = ["Item 1", "Item 2", "Item 3"]
var body: some View {
LazyVStack {
ForEach(items, id: \.self) { item in
Text(item)
.swipeActions {
Button("Delete") {
if let index = items.firstIndex(of: item) {
items.remove(at: index)
}
}
.tint(.red)
}
}
}
}
}
Explanation:
- LazyVStack: This is used to create a vertically scrolling stack of views.
- ForEach: This iterates over the items in your list and creates a view for each item.
- swipeActions: This modifier is used to add swipe actions to each row. In this example, a "Delete" button is added, which removes the item from the list when tapped.
For more detailed information on how to use swipeActions
and other SwiftUI capabilities, you can refer to the SwiftUI essentials session.
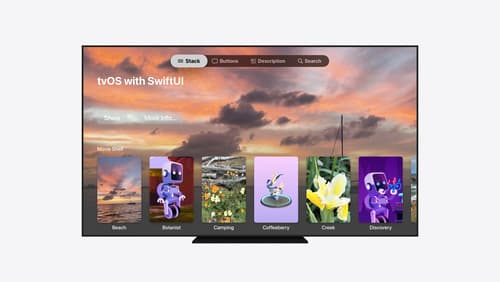
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
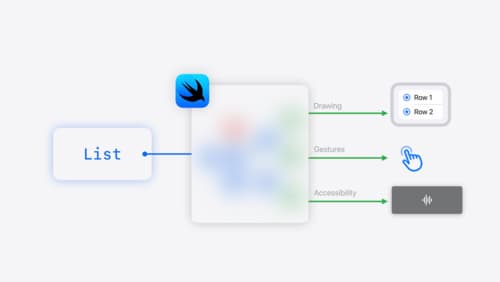
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
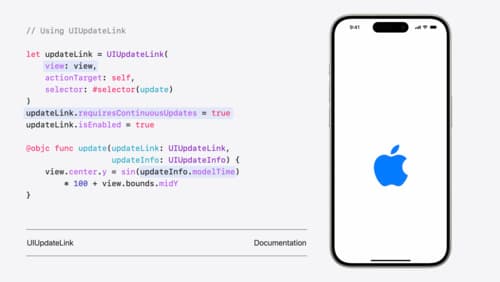
What’s new in UIKit
Explore everything new in UIKit, including tab and document launch experiences, transitions, and text and input changes. We’ll also discuss better-than-ever interoperability between UIKit and SwiftUI animations and gestures, as well as general improvements throughout UIKit.
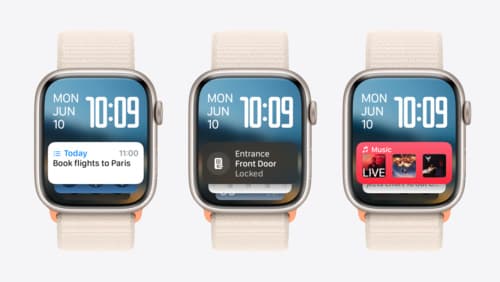
What’s new in watchOS 11
Explore new opportunities on Apple Watch, including bringing Double Tap support to your watchOS app, making your Smart Stack widgets even more relevant and interactive, and displaying your iOS Live Activities in the Smart Stack.