how do i create a swiftUI view
Generated on 7/31/2024
1 search
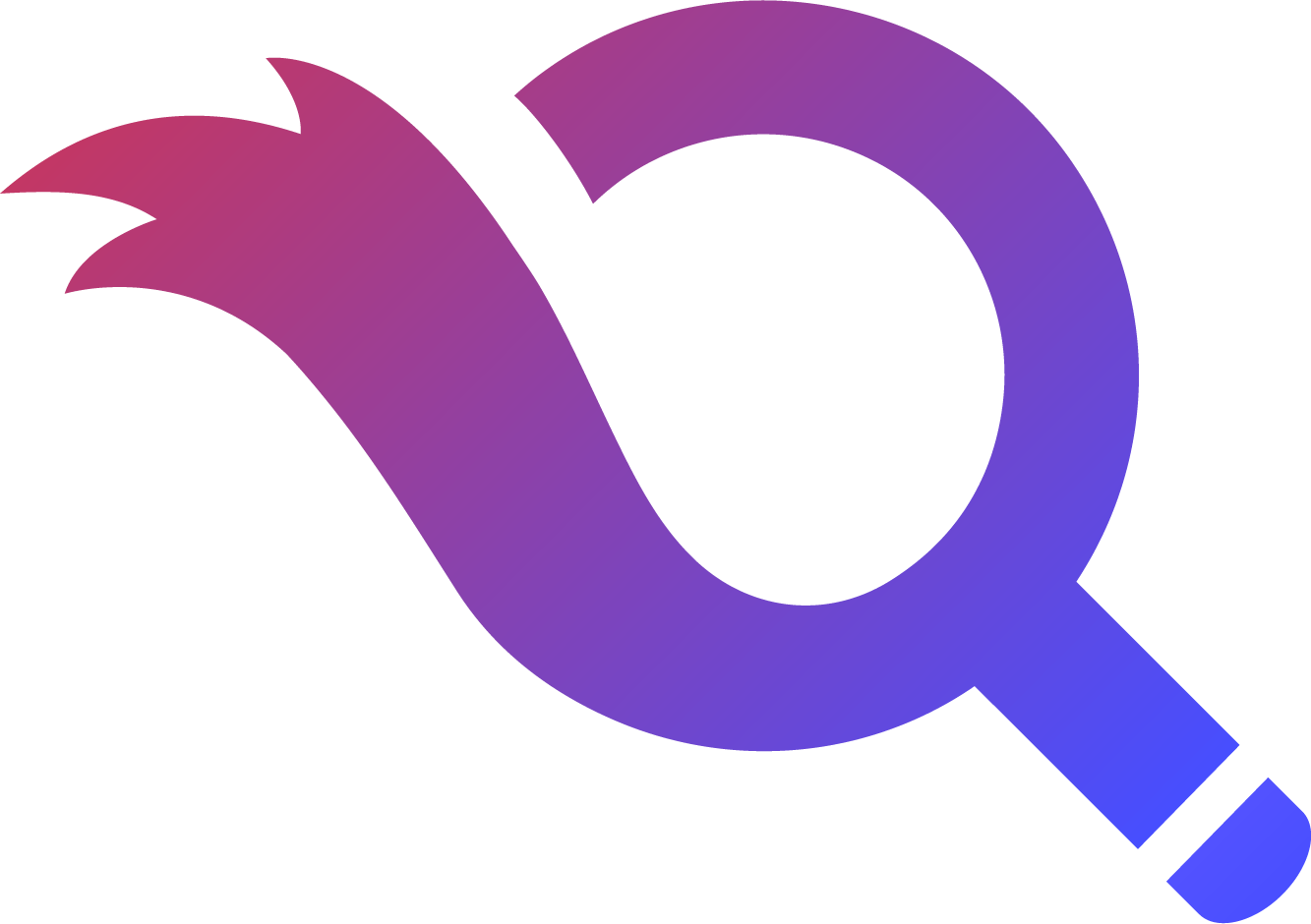
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To create a SwiftUI view, you can follow these steps:
-
Understand the Basics: SwiftUI views are the fundamental building blocks of user interfaces in SwiftUI. They are declarative, compositional, and state-driven. This means you describe what you want the UI to look like, and SwiftUI takes care of rendering it.
-
Start with a Simple View: You can create a simple view using SwiftUI's declarative syntax. For example, you can create a horizontal stack (
HStack
) that contains a label, a spacer, and a text element.import SwiftUI struct ContentView: View { var body: some View { HStack { Label("Pet Tricks", systemImage: "star") Spacer() Text("Best Tricks") } } }
-
Use Built-in Views and Modifiers: SwiftUI provides a variety of built-in views like
Text
,Image
,Button
, and containers likeHStack
,VStack
, andZStack
. You can also use modifiers to customize these views. -
Handle State: SwiftUI views are state-driven. You can use state properties to manage the state of your views. For example, you can use
@State
to create a state property that updates the UI when its value changes.struct ContentView: View { @State private var petName: String = "Buddy" var body: some View { VStack { Text("Pet Name: \(petName)") Button("Change Name") { petName = "Max" } } } }
-
Composition: SwiftUI encourages breaking down your UI into smaller, reusable components. You can create custom views and compose them together.
struct PetView: View { var petName: String var body: some View { Text("Pet Name: \(petName)") } } struct ContentView: View { var body: some View { VStack { PetView(petName: "Buddy") PetView(petName: "Max") } } }
-
Advanced Customization: For more advanced use cases, SwiftUI provides APIs for creating custom controls, layouts, and even integrating with lower-level graphics frameworks like Metal.
For a more detailed introduction, you can watch the session SwiftUI essentials from WWDC 2024.
Relevant Sessions
These sessions cover the fundamentals of SwiftUI, how to use containers, and how to migrate existing apps to SwiftUI.
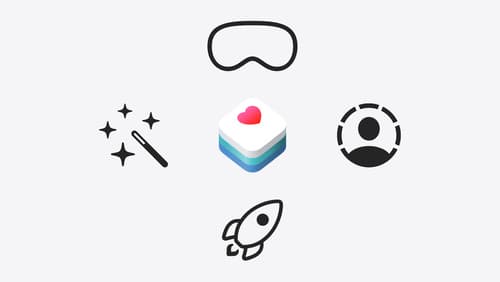
Get started with HealthKit in visionOS
Discover how to use HealthKit to create experiences that take full advantage of the spatial canvas. Learn the capabilities of HealthKit on the platform, find out how to bring an existing iPadOS app to visionOS, and explore the special considerations governing HealthKit during a Guest User session. You’ll also learn ways to use SwiftUI, Swift Charts, and Swift concurrency to craft innovative experiences with HealthKit.
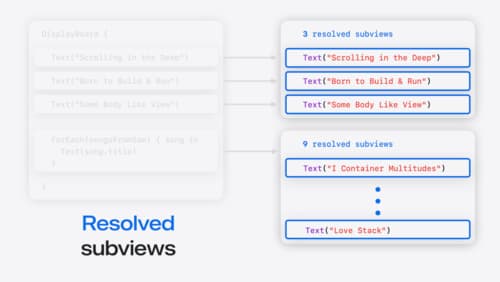
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.
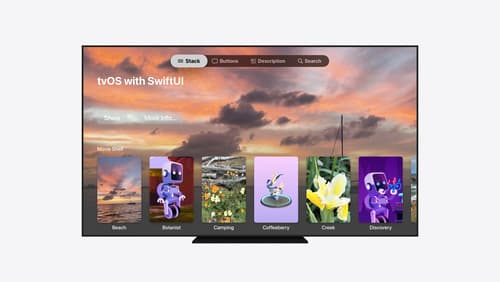
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
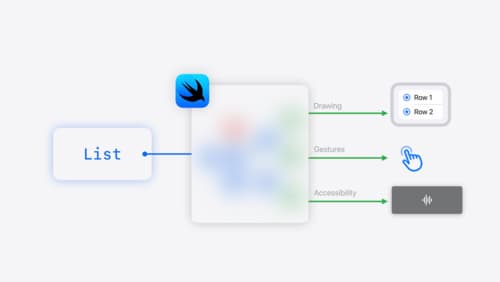
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.