How do I implement a selection in a focus mode extension?
Generated on 7/31/2024
1 search
To implement a selection in a focus mode extension, you can leverage the new capabilities in SwiftUI and AppKit as discussed in the WWDC sessions.
SwiftUI
In SwiftUI, you can manage text selection programmatically within text editing controls. This allows you to read properties of the selection, such as the selected ranges, and use this information to provide features like suggested completions or rhymes.
For example, you can use a binding to update the selection and read the selected text:
@State private var selectedText: String = ""
@State private var text: String = "Your editable text here"
var body: some View {
TextEditor(text: $text)
.onChange(of: text) { newValue in
// Update selectedText based on the new selection
}
}
You can also programmatically drive the focus state of a search field and add text suggestions:
@State private var searchTerm: String = ""
@FocusState private var isSearchFieldFocused: Bool
var body: some View {
TextField("Search", text: $searchTerm)
.focused($isSearchFieldFocused)
.onChange(of: searchTerm) { newValue in
// Provide suggestions based on the search term
}
}
For more details, you can refer to the session What’s new in SwiftUI.
AppKit
In AppKit, you can implement the NSViewContentSelectionInfo
protocol to provide geometry information about the selection, which helps in positioning context menus appropriately near the selection.
Here's a brief example of how you might implement this:
class CustomView: NSView, NSViewContentSelectionInfo {
var selectedRange: NSRange = NSRange(location: 0, length: 0)
func selectionRects(for range: NSRange) -> [NSValue] {
// Return an array of NSValue objects representing the selection rects
}
func selectionBounds(for range: NSRange) -> NSRect {
// Return the bounding rect for the selection
}
}
For more details, you can refer to the session What’s new in AppKit.
Relevant Sessions
These sessions provide comprehensive insights into managing text selection and focus states in both SwiftUI and AppKit.
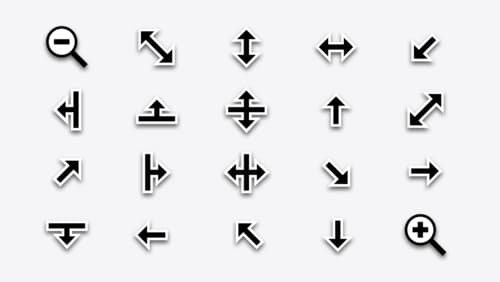
What’s new in AppKit
Discover the latest advances in Mac app development. Get an overview of the new features in macOS Sequoia, and how to adopt them in your app. Explore new ways to integrate your existing code with SwiftUI. Learn about the improvements made to numerous AppKit controls, like toolbars, menus, text input, and more.
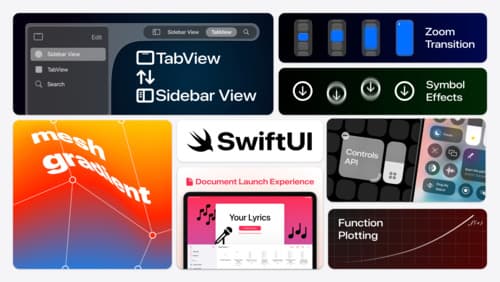
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
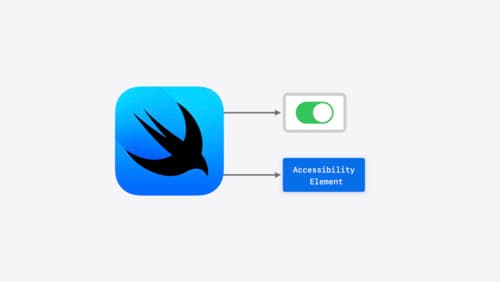
Catch up on accessibility in SwiftUI
SwiftUI makes it easy to build amazing experiences that are accessible to everyone. We’ll discover how assistive technologies understand and navigate your app through the rich accessibility elements provided by SwiftUI. We’ll also discuss how you can further customize these experiences by providing more information about your app’s content and interactions by using accessibility modifiers.
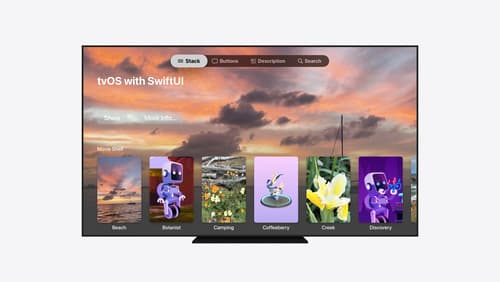
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
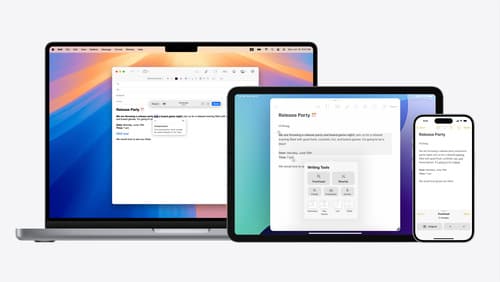
Get started with Writing Tools
Learn how Writing Tools help users proofread, rewrite, and transform text in your app. Get the details on how Writing Tools interact with your app so users can refine what they have written in any text view. Understand how text is retrieved and processed, and how to support Writing Tools in custom text views.