test
Generated on 7/31/2024
5 searches
It looks like you're interested in testing features presented at WWDC. Here are some relevant sessions and details:
Sessions Mentioned
Key Points on Swift Testing
-
Parameterized Tests: Swift testing allows you to write parameterized tests, which can take one or more parameters. This helps in reducing code duplication and makes it easier to test multiple cases efficiently. Each argument in a parameterized test runs as its own test case, which can run in parallel, improving test coverage and performance. (Go further with Swift Testing)
-
Test Macros: The
@Test
macro is used to mark functions as tests. It can accept various traits to add information or change behavior, such as providing a display name or arguments to a test function. (What’s new in Xcode 16) -
Tags and Test Plans: Tags can be used to group tests together, and you can include or exclude tests from test plans based on these tags. This is useful for managing tests during different stages of development. (What’s new in Xcode 16)
-
Test Suites: Test suites allow you to group related tests together. Each test function in a suite is called on a new instance of its containing suite type, ensuring no unintentional state sharing. (Meet Swift Testing)
-
Async and Actor Isolation: Swift testing integrates seamlessly with Swift concurrency, supporting
async/await
and actor isolation. This makes it easier to write tests for asynchronous code. (Meet Swift Testing)
Example of Parameterized Testing
Here's an example of how parameterized testing can be used to test an enumeration of ice cream flavors:
enum IceCreamFlavor {
case vanilla, chocolate, strawberry, mint
var containsNuts: Bool {
switch self {
case .vanilla, .strawberry: return false
case .chocolate, .mint: return true
}
}
}
@testable import MyApp
import XCTest
final class IceCreamFlavorTests: XCTestCase {
@Test
func testContainsNuts(flavor: IceCreamFlavor) {
let expected = flavor == .chocolate || flavor == .mint
XCTAssertEqual(flavor.containsNuts, expected)
}
}
This test function will run for each case in the IceCreamFlavor
enumeration, checking if the containsNuts
property returns the expected value.
For more detailed information, you can watch the sessions:
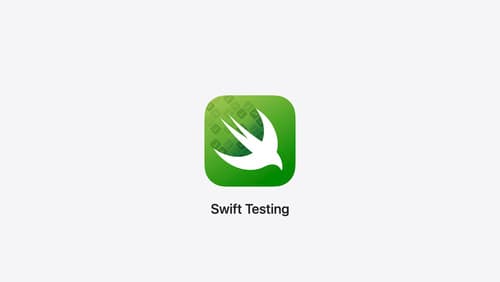
Meet Swift Testing
Introducing Swift Testing: a new package for testing your code using Swift. Explore the building blocks of its powerful new API, discover how it can be applied in common testing workflows, and learn how it relates to XCTest and open source Swift.
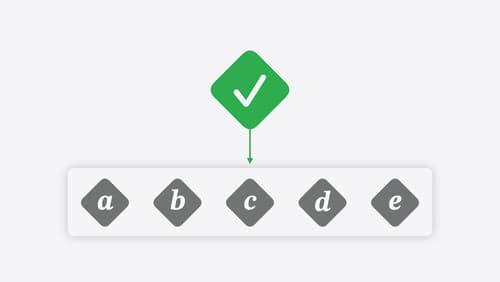
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
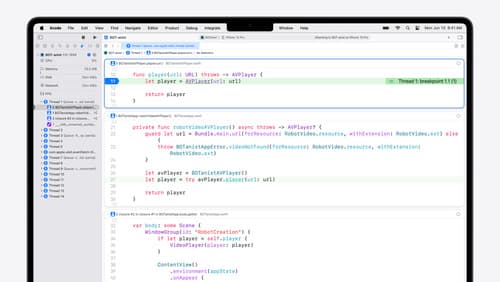
What’s new in Xcode 16
Discover the latest productivity and performance improvements in Xcode 16. Learn about enhancements to code completion, diagnostics, and Xcode Previews. Find out more about updates in builds and explore improvements in debugging and Instruments.