How do I get the user’s location on iOS?
Asked on 07/31/2024
1 search
To get the user's location on iOS, you can use the CoreLocation framework. Here are the steps to do this:
-
Request Authorization: Before accessing the user's location, you need to request authorization. This can be done using the
CLLocationManager
and methods likerequestWhenInUseAuthorization
orrequestAlwaysAuthorization
. -
Handle Authorization States: You need to handle different authorization states such as
notDetermined
,restricted
,denied
,authorizedWhenInUse
, andauthorizedAlways
. -
Use CoreLocation APIs: Once you have the necessary authorization, you can use CoreLocation APIs to get location updates.
Here is a simplified example of how you can achieve this:
import CoreLocation
class LocationManager: NSObject, CLLocationManagerDelegate {
private let locationManager = CLLocationManager()
override init() {
super.init()
locationManager.delegate = self
locationManager.requestWhenInUseAuthorization()
}
func startUpdatingLocation() {
if CLLocationManager.locationServicesEnabled() {
locationManager.startUpdatingLocation()
}
}
func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) {
switch status {
case .notDetermined:
// Request authorization
locationManager.requestWhenInUseAuthorization()
case .restricted, .denied:
// Handle denied state
print("Location access denied")
case .authorizedWhenInUse, .authorizedAlways:
// Start updating location
locationManager.startUpdatingLocation()
@unknown default:
break
}
}
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
if let location = locations.last {
print("Location: \(location.coordinate.latitude), \(location.coordinate.longitude)")
}
}
}
Relevant Sessions from WWDC
- What’s new in location authorization - This session covers the new declarative way to handle location authorization using
CLServiceSession
and diagnostic properties. - Bring context to today’s weather - This session includes examples of using location coordinates to fetch weather data.
For more detailed information, you can refer to the session What’s new in location authorization.
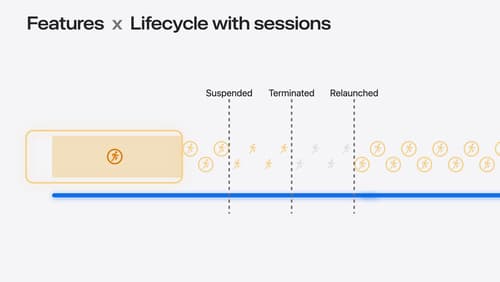
What’s new in location authorization
Location authorization is turning 2.0. Learn about new recommendations and techniques to get the authorization you need, and a new system of diagnostics that can let you know when an authorization goal can’t be met.
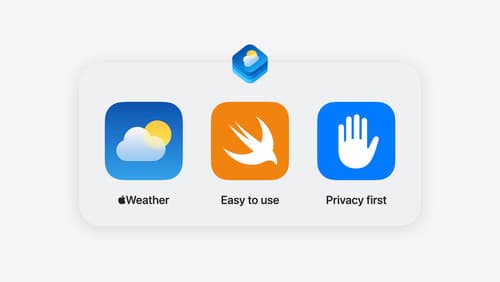
Bring context to today’s weather
Harness the power of WeatherKit to get detailed weather forecast data such as precipitation amounts by type, cloud cover by altitude, or maximum wind speed. Find out how you can summarize weather by different parts of the day and highlight significant upcoming changes to temperature or precipitation. Understand how you can compare current weather to the past through our Historical Comparisons dataset and dive into historical weather statistics for any location in the world. We’ll also explore how you can do all of this faster with our Swift and REST APIs.
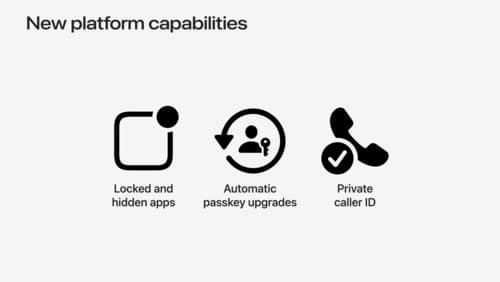
What’s new in privacy
At Apple, we believe privacy is a fundamental human right. Learn about new and improved permission flows and other features that manage data in a privacy-preserving way, so that you can focus on creating great app experiences.