how to add semantic search to my app?
Generated on 7/31/2024
1 search
To add semantic search to your app, you can leverage the Core Spotlight framework, which now supports query understanding with SemanticSearch. Here are the steps to get started:
-
Donate Searchable Content:
- First, you need to donate searchable content to Spotlight. This involves creating
CSSearchableItem
objects for the content you want to be searchable. Each item should have a unique identifier and an attribute set that describes the content. - Example: In a journaling app, each journal entry can be a searchable item.
let attributeSet = CSSearchableItemAttributeSet(itemContentType: kUTTypeText as String) attributeSet.title = "Journal Entry Title" attributeSet.contentDescription = "Journal Entry Content" let item = CSSearchableItem(uniqueIdentifier: "uniqueID", domainIdentifier: "journalEntries", attributeSet: attributeSet) CSSearchableIndex.default().indexSearchableItems([item]) { error in if let error = error { print("Indexing error: \(error.localizedDescription)") } else { print("Search item successfully indexed!") } }
- First, you need to donate searchable content to Spotlight. This involves creating
-
Configure Queries:
- Semantic search is enabled by default, but you can configure your queries to return ranked results using the same machine learning models that Spotlight uses. You can also configure a suggestions menu in your app.
- Use
CSUserQueryContext
to configure the query according to your UI needs.
let query = CSSearchQuery(queryString: "search terms", attributes: ["title", "contentDescription"]) query.foundItemsHandler = { items in // Handle found items } query.completionHandler = { error in if let error = error { print("Search error: \(error.localizedDescription)") } else { print("Search completed successfully!") } } query.start()
-
Boost Ranking of Search Results:
- You can boost the ranking of search results that are most relevant to the user by using the
compareByRank
comparator and setting priorities for indexed entities.
let sortedItems = items.sorted { $0.rank > $1.rank }
- You can boost the ranking of search results that are most relevant to the user by using the
-
Integrate with App Intents:
- If your application is already indexing content in Spotlight via the
CSSearchableItem
API, you can use the newassociateAppEntity
method to associate an app entity with your searchable item before you index it. This allows the new semantic search to find information about your app entity.
let entity = MyAppEntity(identifier: "uniqueID", displayName: "Journal Entry") CSSearchableIndex.default().indexSearchableItems([item]) { error in if let error = error { print("Indexing error: \(error.localizedDescription)") } else { print("Search item successfully indexed!") } }
- If your application is already indexing content in Spotlight via the
For a detailed walkthrough, you can refer to the session Support semantic search with Core Spotlight from WWDC 2024.
Relevant Sessions
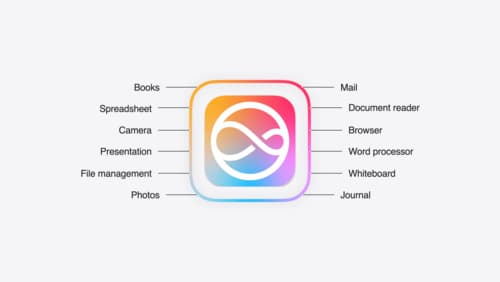
Bring your app to Siri
Learn how to use App Intents to expose your app’s functionality to Siri. Understand which intents are already available for your use, and how to create custom intents to integrate actions from your app into the system. We’ll also cover what metadata to provide, making your entities searchable via Spotlight, annotating onscreen references, and much more.
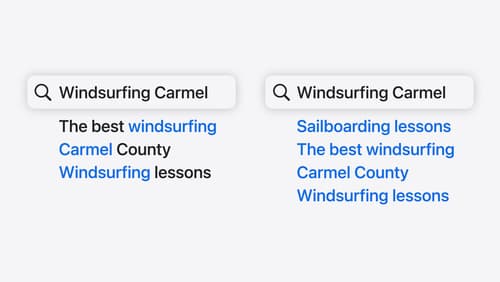
Support semantic search with Core Spotlight
Learn how to provide semantic search results in your app using Core Spotlight. Understand how to make your app’s content available in the user’s private, on-device index so people can search for items using natural language. We’ll also share how to optimize your app’s performance by scheduling indexing activities. To get the most out of this session, we recommend first checking out Core Spotlight documentation on the Apple Developer website.
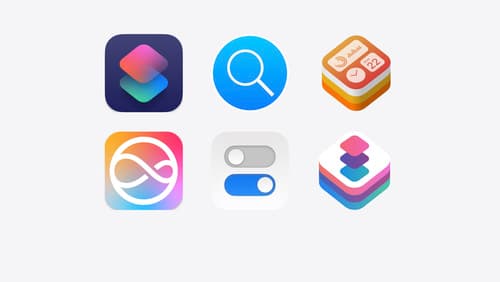
What’s new in App Intents
Learn about improvements and all-new features with App Intents, and discover how this framework can help you expose your app’s functionality to Siri, Spotlight, Shortcuts, and more. We’ll show you how to make your entities more meaningful to the platform with the Transferable API, File Representations, new IntentFile APIs, and Spotlight Indexing, opening up powerful functionality in Siri and the Shortcuts app. Empower your intents to take people deep into your app with URL Representable Entities. Explore new techniques to model your entities and intents with new APIs for error handling and union values.