how to rerender a uikit view via swiftui?
Generated on 7/31/2024
1 search
To rerender a UIKit view via SwiftUI, you can use the UIViewRepresentable
protocol to wrap your UIKit view and integrate it into your SwiftUI view hierarchy. This allows you to update the UIKit view using SwiftUI's declarative syntax.
Here's a step-by-step guide based on the information from the WWDC sessions:
-
Create a
UIViewRepresentable
: This protocol allows you to create and update a UIKit view using SwiftUI.struct MyUIKitView: UIViewRepresentable { func makeUIView(context: Context) -> UIView { // Create and return the UIKit view } func updateUIView(_ uiView: UIView, context: Context) { // Update the UIKit view with new data } }
-
Use the
UIViewRepresentable
in SwiftUI: You can now use this representable view in your SwiftUI view hierarchy like any other SwiftUI view.struct ContentView: View { var body: some View { MyUIKitView() } }
-
Animating UIKit Views with SwiftUI Animations: If you need to animate the UIKit view, you can bridge the animation from SwiftUI to UIKit. Use the new
animate
method on the context to apply any animation on the transaction associated with the update.struct MyUIKitView: UIViewRepresentable { @Binding var isOpen: Bool func makeUIView(context: Context) -> UIView { // Create and return the UIKit view } func updateUIView(_ uiView: UIView, context: Context) { // Bridge the animation from SwiftUI to UIKit UIView.animate(withDuration: context.transaction.animation?.duration ?? 0) { uiView.alpha = self.isOpen ? 1.0 : 0.0 } } }
For more detailed information, you can refer to the following sessions from WWDC:
- SwiftUI essentials (20:45)
- Enhance your UI animations and transitions (10:23)
These sessions cover the interoperability between SwiftUI and UIKit, and how to animate UIKit views using SwiftUI animations.
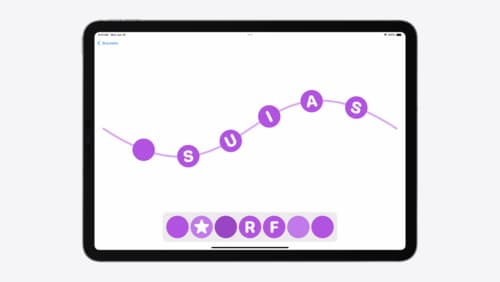
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.
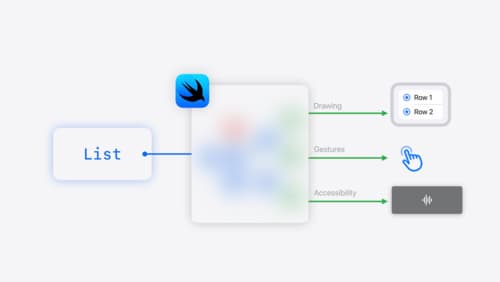
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.