how to play a apple music song in swiftui?
Asked on 07/31/2024
1 search
To play an Apple Music song in SwiftUI, you can use the MusicKit
framework, which provides APIs for integrating Apple Music into your app. Here's a basic example of how you might do this:
-
Import MusicKit: First, make sure to import the
MusicKit
framework. -
Request Authorization: Request authorization to access the user's Apple Music library.
-
Fetch and Play a Song: Use the
MusicPlayer
to play a song.
Here's a simple example:
import SwiftUI
import MusicKit
struct ContentView: View {
@State private var musicPlayer = MusicPlayer.shared
var body: some View {
VStack {
Button(action: {
Task {
do {
// Request authorization
let status = await MusicAuthorization.request()
guard status == .authorized else { return }
// Fetch a song by its ID
let songID = MusicItemID("your-song-id-here")
let song = try await MusicCatalogResourceRequest<Song>(matching: \.id, equalTo: songID).response().items.first
// Play the song
if let song = song {
musicPlayer.queue = [song]
try await musicPlayer.play()
}
} catch {
print("Error playing song: \(error)")
}
}
}) {
Text("Play Song")
}
}
}
}
Steps Explained:
- Import MusicKit: This allows you to use the MusicKit framework.
- Request Authorization: The app requests permission to access the user's Apple Music library.
- Fetch and Play a Song: The app fetches a song using its ID and then plays it using the
MusicPlayer
.
For more detailed information on integrating media playback in SwiftUI, you might find the session Tailor macOS windows with SwiftUI useful, as it discusses organizing and playing videos, which can be analogous to playing music.
If you need more specific details or examples, you can refer to the session Migrate your TVML app to SwiftUI, which covers building media apps with SwiftUI.
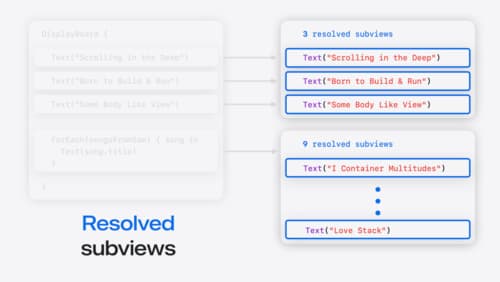
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.
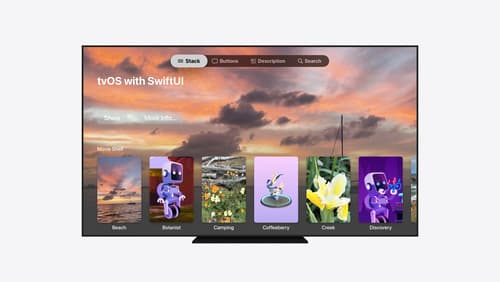
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
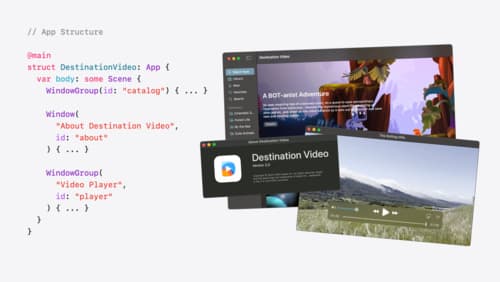
Tailor macOS windows with SwiftUI
Make your windows feel tailor-made for macOS. Fine-tune your app’s windows for focused purposes, ease of use, and to express functionality. Use SwiftUI to style window toolbars and backgrounds. Arrange your windows with precision, and make smart decisions about restoration and minimization.