how do you select an item in a NSMenu
Asked on 07/31/2024
1 search
To select an item in an NSMenu
, you can use the NSMenu
API provided by AppKit. Here is a basic example of how you can create an NSMenu
, add items to it, and select an item programmatically:
import Cocoa
// Create a new NSMenu
let menu = NSMenu(title: "Example Menu")
// Add items to the menu
menu.addItem(withTitle: "Item 1", action: #selector(menuItemSelected(_:)), keyEquivalent: "")
menu.addItem(withTitle: "Item 2", action: #selector(menuItemSelected(_:)), keyEquivalent: "")
menu.addItem(withTitle: "Item 3", action: #selector(menuItemSelected(_:)), keyEquivalent: "")
// Function to handle menu item selection
@objc func menuItemSelected(_ sender: NSMenuItem) {
print("Selected item: \(sender.title)")
}
// Select an item programmatically
if let itemToSelect = menu.item(withTitle: "Item 2") {
menu.performActionForItem(at: menu.index(of: itemToSelect))
}
In this example:
- An
NSMenu
is created and items are added to it. - Each item has an associated action (
menuItemSelected(_:)
) that is called when the item is selected. - The
performActionForItem(at:)
method is used to programmatically select an item by its index.
For more detailed information on NSMenu
and its capabilities, you can refer to the What’s new in AppKit session from WWDC 2024.
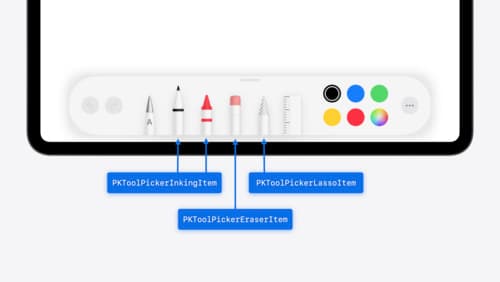
Squeeze the most out of Apple Pencil
New in iOS 18, iPadOS 18, and visionOS 2, the PencilKit tool picker gains the ability to have completely custom tools, with custom attributes. Learn how to express your custom drawing experience in the tool picker using the same great tool picking experience available across the system. Discover how to access the new features of the Apple Pencil Pro, including roll angle, the squeeze gesture, and haptic feedback.
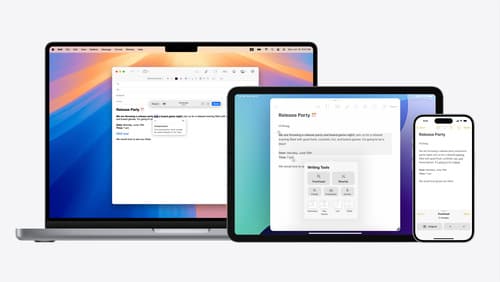
Get started with Writing Tools
Learn how Writing Tools help users proofread, rewrite, and transform text in your app. Get the details on how Writing Tools interact with your app so users can refine what they have written in any text view. Understand how text is retrieved and processed, and how to support Writing Tools in custom text views.
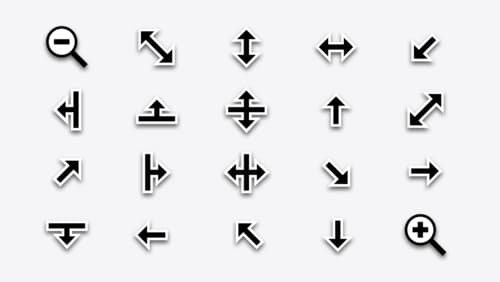
What’s new in AppKit
Discover the latest advances in Mac app development. Get an overview of the new features in macOS Sequoia, and how to adopt them in your app. Explore new ways to integrate your existing code with SwiftUI. Learn about the improvements made to numerous AppKit controls, like toolbars, menus, text input, and more.