how to add swiftlint to third party packages
Generated on 7/31/2024
1 search
To add SwiftLint to third-party packages, you can follow these general steps:
-
Add SwiftLint as a Dependency: You need to add SwiftLint as a dependency in your
Package.swift
file. This can be done by including it in thedependencies
array.// swift-tools-version:5.3 import PackageDescription let package = Package( name: "YourPackageName", dependencies: [ .package(url: "https://github.com/realm/SwiftLint.git", from: "0.43.0") ], targets: [ .target( name: "YourTargetName", dependencies: ["SwiftLint"] ) ] )
-
Configure SwiftLint: Create a
.swiftlint.yml
file in the root of your package to configure the rules and settings for SwiftLint.disabled_rules: # rule identifiers to exclude from running - line_length - type_name opt_in_rules: # some rules are only opt-in - empty_count - missing_docs included: # paths to include during linting. `--path` is ignored if present. - Source excluded: # paths to ignore during linting. Takes precedence over `included`. - Carthage - Pods
-
Run SwiftLint: You can run SwiftLint manually from the command line or integrate it into your build process. To run it manually, navigate to your package directory and execute:
swift run swiftlint
-
Integrate with Xcode: If you are using Xcode, you can add a build phase to run SwiftLint automatically. Go to your Xcode project settings, select your target, and add a new "Run Script Phase" with the following script:
if which swiftlint >/dev/null; then swiftlint else echo "warning: SwiftLint not installed, download from https://github.com/realm/SwiftLint" fi
For more detailed information on working with Swift packages, you can refer to the session A Swift Tour: Explore Swift’s features and design.
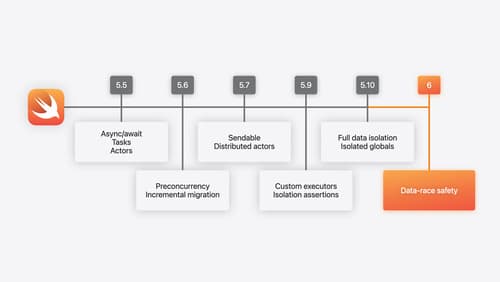
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
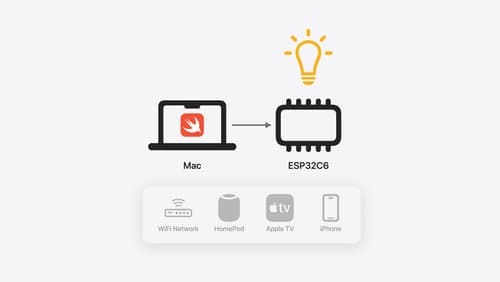
Go small with Embedded Swift
Embedded Swift brings the safety and expressivity of Swift to constrained environments. Explore how Embedded Swift runs on a variety of microcontrollers through a demonstration using an off-the-shelf Matter device. Learn how the Embedded Swift subset packs the benefits of Swift into a tiny footprint with no runtime, and discover plenty of resources to start your own Embedded Swift adventure.
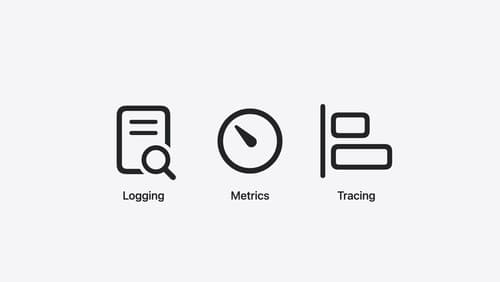
Explore the Swift on Server ecosystem
Swift is a great language for writing your server applications, and powers critical services across Apple’s cloud products. We’ll explore tooling, delve into the Swift server package ecosystem, and demonstrate how to interact with databases and add observability to applications.
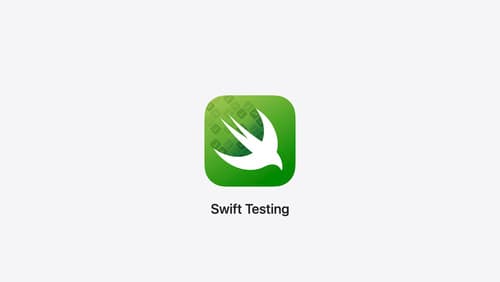
Meet Swift Testing
Introducing Swift Testing: a new package for testing your code using Swift. Explore the building blocks of its powerful new API, discover how it can be applied in common testing workflows, and learn how it relates to XCTest and open source Swift.
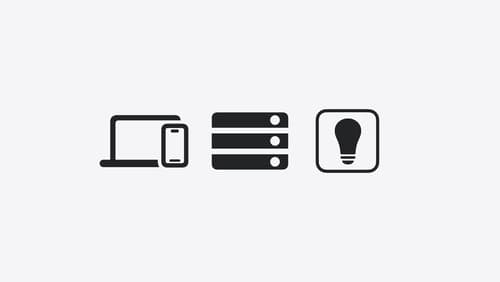
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
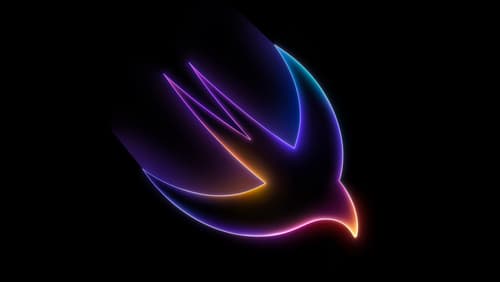
Platforms State of the Union
Discover the newest advancements on Apple platforms.