how to create multiple swift packages and add them as dependency on another package
Generated on 7/31/2024
1 search
To create multiple Swift packages and add them as dependencies on another package, you can follow these steps:
-
Create Swift Packages:
- Use the Swift Package Manager (SPM) to create your packages. You can do this from the command line:
swift package init --type library
- This command will create a new Swift package with a library target.
- Use the Swift Package Manager (SPM) to create your packages. You can do this from the command line:
-
Define Dependencies:
- In each package, you can define dependencies in the
Package.swift
file. For example:// swift-tools-version:5.5 import PackageDescription let package = Package( name: "MyLibrary", products: [ .library( name: "MyLibrary", targets: ["MyLibrary"]), ], dependencies: [ // Dependencies declare other packages that this package depends on. .package(url: "https://github.com/another/package.git", from: "1.0.0"), ], targets: [ .target( name: "MyLibrary", dependencies: []), .testTarget( name: "MyLibraryTests", dependencies: ["MyLibrary"]), ] )
- In each package, you can define dependencies in the
-
Add Dependencies to Another Package:
- To add these packages as dependencies to another package, you need to update the
Package.swift
file of the main package:// swift-tools-version:5.5 import PackageDescription let package = Package( name: "MainPackage", products: [ .executable( name: "MainPackage", targets: ["MainPackage"]), ], dependencies: [ .package(path: "../MyLibrary1"), .package(path: "../MyLibrary2"), ], targets: [ .target( name: "MainPackage", dependencies: ["MyLibrary1", "MyLibrary2"]), ] )
- To add these packages as dependencies to another package, you need to update the
-
Build and Test:
- You can build and test your packages using the command line:
swift build swift test
- You can build and test your packages using the command line:
For more detailed information on working with Swift packages, you can refer to the session A Swift Tour: Explore Swift’s features and design which covers code organization and the use of Swift Package Manager.
Relevant Sessions
- A Swift Tour: Explore Swift’s features and design
- What’s new in Swift
- Explore the Swift on Server ecosystem
These sessions provide insights into creating and managing Swift packages, as well as using them in different environments.
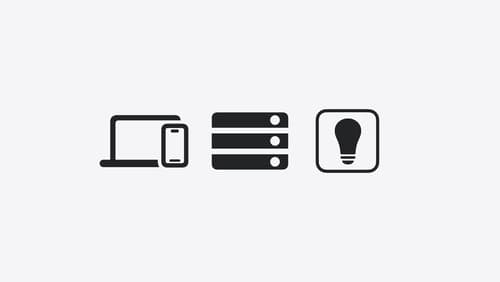
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
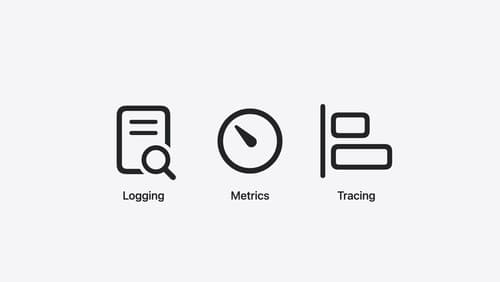
Explore the Swift on Server ecosystem
Swift is a great language for writing your server applications, and powers critical services across Apple’s cloud products. We’ll explore tooling, delve into the Swift server package ecosystem, and demonstrate how to interact with databases and add observability to applications.
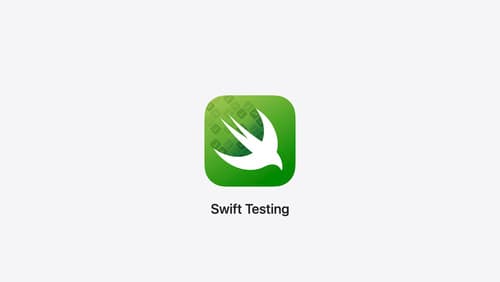
Meet Swift Testing
Introducing Swift Testing: a new package for testing your code using Swift. Explore the building blocks of its powerful new API, discover how it can be applied in common testing workflows, and learn how it relates to XCTest and open source Swift.
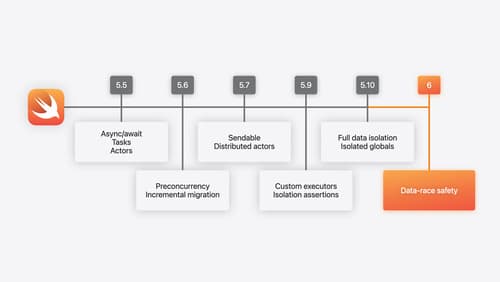
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
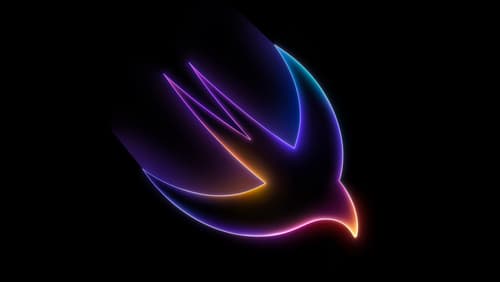
Platforms State of the Union
Discover the newest advancements on Apple platforms.