What's the Swift's concurrency model
Generated on 7/31/2024
1 search
Swift's concurrency model is designed to simplify and enhance the safety of concurrent programming. Here are the key components and features:
-
Tasks: The fundamental unit of concurrency in Swift is a task, which represents an independent concurrent execution context. Tasks are lightweight and can be created in large numbers. They can execute concurrently, making them suitable for handling multiple operations simultaneously. Tasks can be awaited or canceled as needed (A Swift Tour: Explore Swift’s features and design).
-
Async/Await: Swift uses the
async
andawait
keywords to handle asynchronous operations. Anasync
function can suspend its execution to wait for an asynchronous operation to complete, allowing other tasks to run in the meantime. This helps in writing clear and concise asynchronous code (A Swift Tour: Explore Swift’s features and design). -
Actors: Actors are reference types that encapsulate mutable state and automatically protect it by serializing access. Only one task can execute on an actor at a time, ensuring thread safety. Calls to actor methods from outside the actor's context are asynchronous (A Swift Tour: Explore Swift’s features and design).
-
Main Actor: The
MainActor
is a special actor that ensures code runs on the main thread, which is crucial for UI updates. By isolating UI-related code to theMainActor
, Swift ensures that UI updates are thread-safe (Migrate your app to Swift 6). -
Data-Race Safety: Swift's concurrency model aims to eliminate data races, which occur when multiple threads access shared data simultaneously and at least one thread modifies it. Swift 6 introduces data-race safety by default, turning potential data race issues into compile-time errors. This significantly improves the reliability and security of concurrent code (What’s new in Swift).
-
Structured Concurrency: Swift's concurrency model encourages structured concurrency, where the lifetime of tasks is tied to the scope in which they are created. This helps in managing the lifecycle of tasks and ensures that resources are properly released when tasks complete (A Swift Tour: Explore Swift’s features and design).
For more detailed information, you can refer to the sessions:
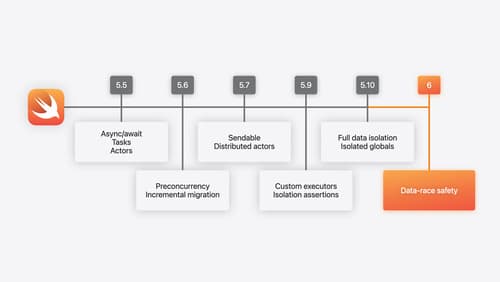
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
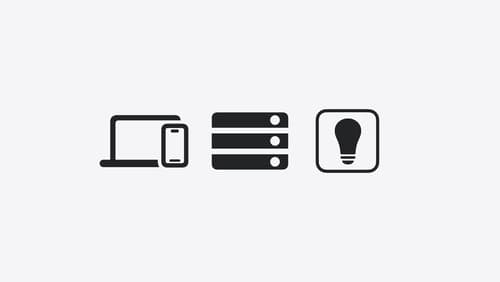
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
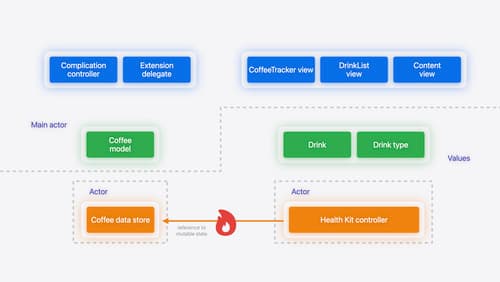
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.