Sample code to play HLS content
Asked on 08/01/2024
1 search
To play HLS content and enhance ad experiences with HLS interstitials, you can refer to the session Enhance ad experiences with HLS interstitials from WWDC 2024. This session provides a comprehensive overview of how to insert ads or other auxiliary content into your main content using HLS interstitials.
Here's a basic example of how you might set up HLS interstitials in your app:
import AVFoundation
import AVKit
// Create an AVPlayer with your primary content URL
let primaryContentURL = URL(string: "https://example.com/primary.m3u8")!
let player = AVPlayer(url: primaryContentURL)
// Create an AVPlayerViewController and present it
let playerViewController = AVPlayerViewController()
playerViewController.player = player
// Present the player view controller
present(playerViewController, animated: true) {
player.play()
}
// Schedule an interstitial at 5 seconds into the content
let interstitialURL = URL(string: "https://example.com/interstitial.m3u8")!
let interstitialEvent = AVPlayerInterstitialEvent(primaryItem: player.currentItem!, time: CMTime(seconds: 5, preferredTimescale: 1), templateItems: [AVPlayerItem(url: interstitialURL)])
// Add the interstitial event to the player
player.currentItem?.interstitialEventMonitor.add(interstitialEvent)
This code sets up an AVPlayer
to play primary content and schedules an interstitial to play at 5 seconds into the content. The interstitial content will play and then resume the primary content after the interstitial completes.
For more detailed information and advanced usage, you can watch the session Enhance ad experiences with HLS interstitials from WWDC 2024.
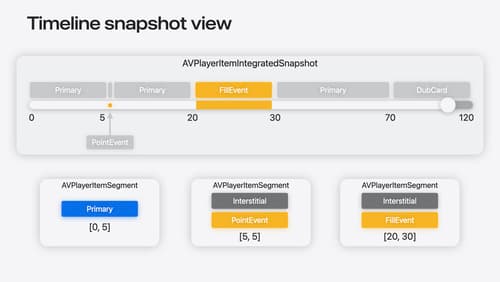
Enhance ad experiences with HLS interstitials
Explore how HLS Interstitials can help you seamlessly insert advertisements into your HLS content. We’ll also show you how to use integrated timeline to tune your UI experience and build SharePlay for interstitials.
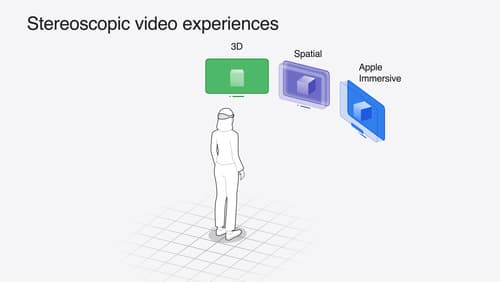
Build compelling spatial photo and video experiences
Learn how to adopt spatial photos and videos in your apps. Explore the different types of stereoscopic media and find out how to capture spatial videos in your iOS app on iPhone 15 Pro. Discover the various ways to detect and present spatial media, including the new QuickLook Preview Application API in visionOS. And take a deep dive into the metadata and stereo concepts that make a photo or video spatial.
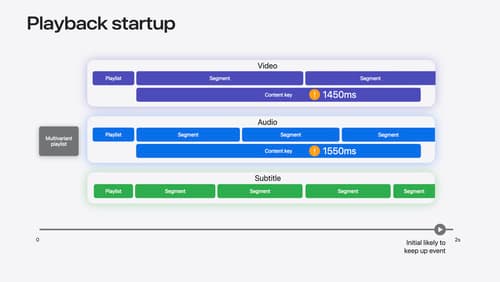
Discover media performance metrics in AVFoundation
Discover how you can monitor, analyze, and improve user experience with the new media performance APIs. Explore how to monitor AVPlayer performance for HLS assets using different AVMetricEvents, and learn how to use these metrics to understand and triage player performance issues.