how to create an animated page transition?
Asked on 08/01/2024
1 search
To create an animated page transition in SwiftUI, you can use custom transitions and modifiers to achieve the desired effect. Here are some steps and examples based on the content from WWDC sessions:
-
Using Built-in Transitions:
- SwiftUI provides built-in transitions like opacity, scale, and combined transitions. You can start with these to create simple animations.
- Example:
.transition(.opacity) .transition(.scale) .transition(.opacity.combined(with: .scale))
-
Creating Custom Transitions:
- For more complex animations, you can create custom transitions by conforming to the
Transition
protocol. - Example from the session "Create custom visual effects with SwiftUI":
struct Twirl: Transition { func body(content: Content, phase: TransitionPhase) -> some View { content .scaleEffect(phase == .identity ? 1 : 0.5) .opacity(phase == .identity ? 1 : 0) .rotationEffect(Angle(degrees: phase == .identity ? 0 : 360)) } } .transition(Twirl())
- For more complex animations, you can create custom transitions by conforming to the
-
Zoom Transitions:
- The new zoom transition in iOS 18 can be used to create a smooth and interactive transition effect.
- Example from the session "Enhance your UI animations and transitions":
.navigationTransitionStyle(.zoom)
-
Combining Effects:
- You can combine multiple effects like blur, rotation, and brightness to create a more dynamic transition.
- Example from the session "Create custom visual effects with SwiftUI":
.blur(radius: phase == .identity ? 0 : 10) .rotationEffect(Angle(degrees: phase == .identity ? 0 : -360)) .brightness(phase == .identity ? 0 : 0.5)
For a detailed walkthrough, you can refer to the following sessions:
- Create custom visual effects with SwiftUI (09:10)
- Enhance your UI animations and transitions (02:07)
These sessions provide comprehensive examples and explanations on how to implement and customize transitions in SwiftUI.
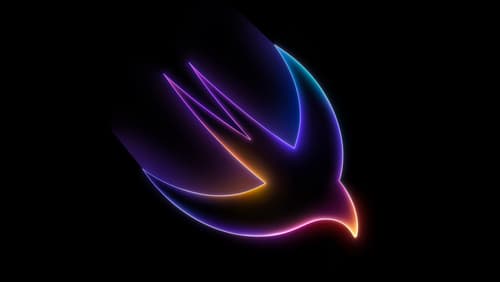
Platforms State of the Union
Discover the newest advancements on Apple platforms.
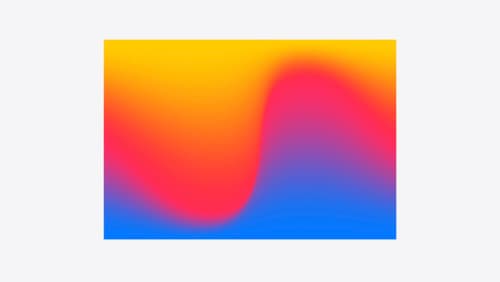
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.
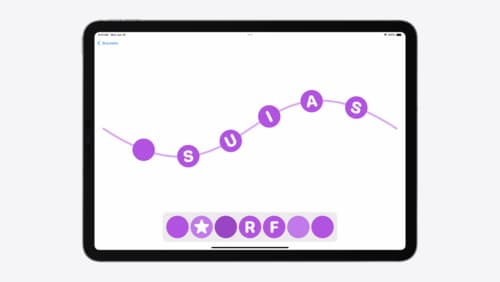
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.