how do i make a swiftui view that displays localUser.name.first automatically update when first changes? localUser and name are both classes, and first is a string
Asked on 08/01/2024
1 search
To make a SwiftUI view that automatically updates when localUser.name.first
changes, you need to use the @ObservableObject
and @Published
property wrappers. Here's a step-by-step guide:
-
Define the ObservableObject: Make
localUser
conform toObservableObject
and mark thefirst
property with@Published
. -
Use the ObservableObject in your View: Use the
@ObservedObject
property wrapper in your SwiftUI view to observe changes tolocalUser
.
Here's an example implementation:
import SwiftUI
import Combine
// Define the classes
class Name: ObservableObject {
@Published var first: String
init(first: String) {
self.first = first
}
}
class LocalUser: ObservableObject {
@Published var name: Name
init(name: Name) {
self.name = name
}
}
// Define the SwiftUI view
struct ContentView: View {
@ObservedObject var localUser: LocalUser
var body: some View {
Text(localUser.name.first)
.padding()
}
}
// Example usage
@main
struct MyApp: App {
var body: some Scene {
WindowGroup {
ContentView(localUser: LocalUser(name: Name(first: "John")))
}
}
}
In this example:
Name
andLocalUser
are both classes that conform toObservableObject
.- The
first
property inName
is marked with@Published
to notify SwiftUI of changes. - The
ContentView
uses@ObservedObject
to observe changes tolocalUser
.
When localUser.name.first
changes, SwiftUI will automatically update the view to reflect the new value.
For more details on state management in SwiftUI, you can refer to the SwiftUI essentials session from WWDC 2024.
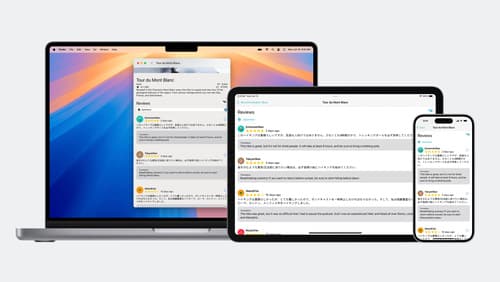
Meet the Translation API
Discover how you can translate text across different languages in your app using the new Translation framework. We’ll show you how to quickly display translations in the system UI, and how to translate larger batches of text for your app’s UI.
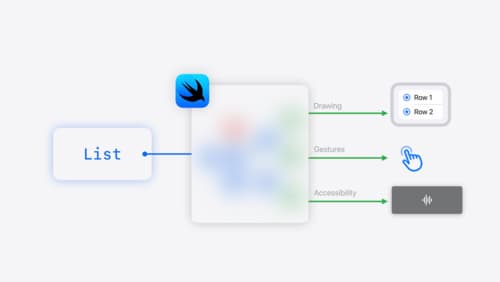
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
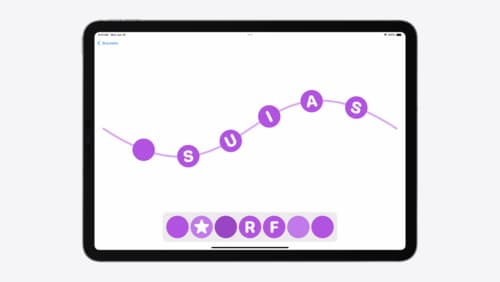
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.