how to synthesize vars
Asked on 08/01/2024
1 search
To synthesize variables in Swift, you can use the var
keyword to declare mutable variables. Here are some key points from the WWDC sessions that might help:
-
Declaring Variables:
- Use
var
to declare a mutable variable. - Use
let
to declare an immutable variable. - Example:
var x = 10 let y = 20
- Use
-
Handling Mutability:
- If you want to ensure a variable does not change, use
let
instead ofvar
. This guarantees immutability. - Example from A Swift Tour: Explore Swift’s features and design:
let username = "Alice"
- If you want to ensure a variable does not change, use
-
Memory Management:
- When a
var
is captured by an escaping closure, its lifetime is extended, and it may need to be heap allocated. - Example from Explore Swift performance:
var capturedVar = 10 let closure = { [capturedVar] in print(capturedVar) }
- When a
-
Concurrency and Data Races:
- Switching a
var
to alet
can help avoid data races in concurrent code. - Example from Migrate your app to Swift 6:
let safeVar = 10
- Switching a
-
Optimizing Performance:
- Swift can optimize variable usage to avoid unnecessary copies, especially with value types like arrays.
- Example from Explore Swift performance:
var array = [1, 2, 3] var anotherArray = array
For more detailed information, you can refer to the sessions mentioned above.
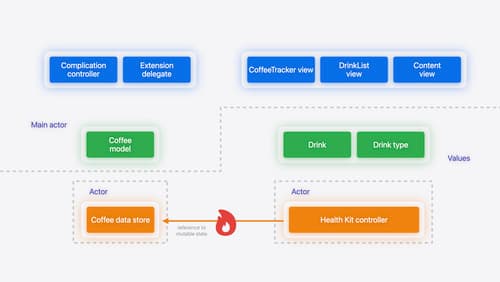
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
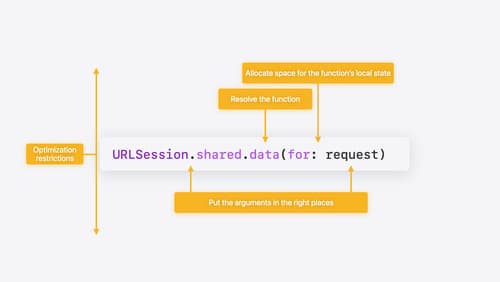
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.
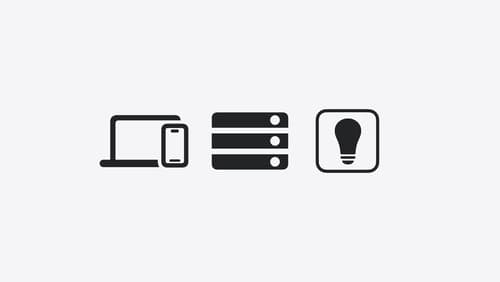
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.