What's the best way to use async in Swift
Generated on 8/1/2024
1 search
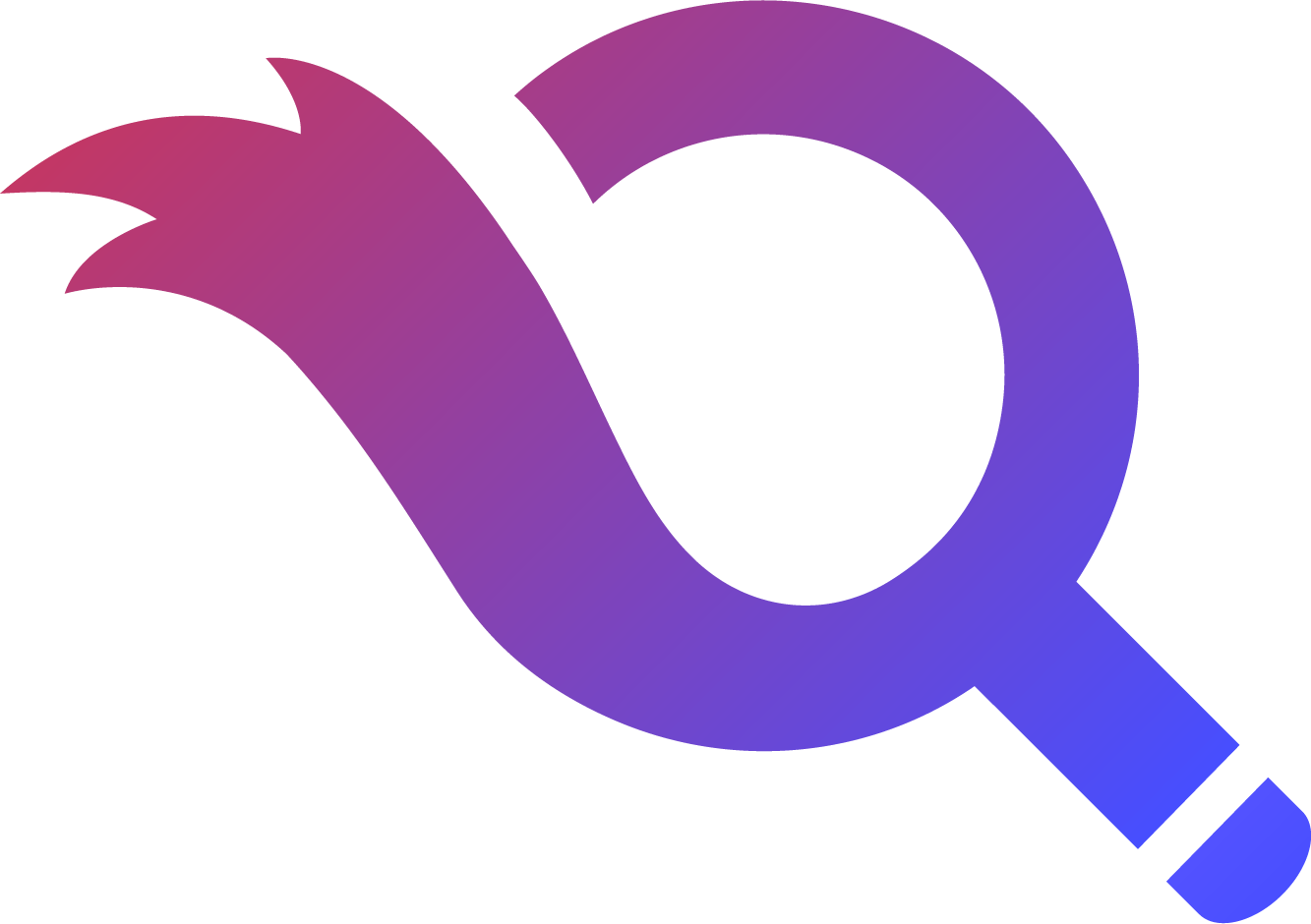
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
The best way to use async in Swift involves leveraging the async
and await
keywords to handle asynchronous operations. Here are the key points:
-
Task Suspension: When a task is waiting for an asynchronous operation to complete, it suspends to yield the CPU to other tasks. This is modeled in code using the
async
andawait
syntax. A function that may suspend is marked with theasync
keyword, and when calling an async function, theawait
keyword is used to indicate that a suspension can occur on that line. This allows other tasks to run while waiting for the async operation to complete. -
Concurrency Features: Swift's concurrency features include tasks, async/await, and actors. Tasks represent independent concurrent execution contexts and are lightweight, allowing for the creation of many tasks. Tasks can execute concurrently, making them suitable for handling operations like HTTP requests.
-
Actors: Actors are reference types that encapsulate shared mutable state and automatically protect their state by serializing accesses. Calls to actor methods from outside the context of the actor are asynchronous, ensuring safe concurrent access.
-
Testing with Async: When writing concurrent test code, you can use the same concurrency features in Swift as in production code. The
await
keyword works the same way in tests, allowing other test code to keep the CPU busy while work is pending. For older code using completion handlers, Swift provides async overloads or you can usewithCheckedContinuation
to convert it to an awaitable expression.
For more detailed information, you can refer to the following sessions from WWDC 2024:
- A Swift Tour: Explore Swift’s features and design (18:33)
- Migrate your app to Swift 6 (08:53)
- Go further with Swift Testing (24:26)
These sessions cover the basics of writing concurrent code in Swift, the use of actors, and techniques for testing asynchronous conditions.
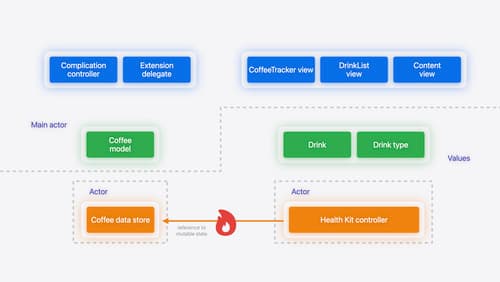
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.
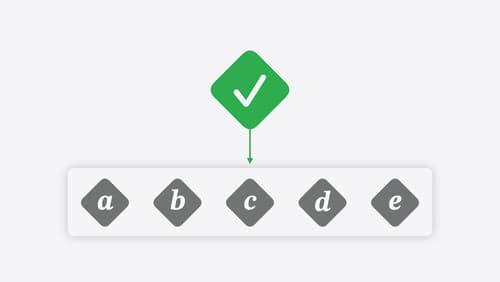
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
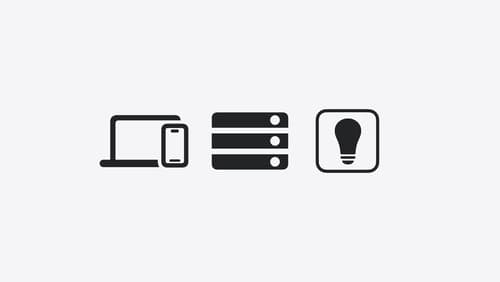
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.