how do i pass a "@State var purchaseManager" as environment to a child view? purchaseManager is @Observable.
Asked on 08/01/2024
1 search
To pass a @State
variable like @State var purchaseManager
as an environment object to a child view in SwiftUI, you need to follow these steps:
-
Declare the Observable Object: Ensure that
purchaseManager
conforms to theObservableObject
protocol.class PurchaseManager: ObservableObject { // Your properties and methods here }
-
Initialize the State Variable: Initialize the
@State
variable in your parent view.struct ParentView: View { @State var purchaseManager = PurchaseManager() var body: some View { ChildView() .environmentObject(purchaseManager) } }
-
Use the Environment Object in the Child View: In the child view, use the
@EnvironmentObject
property wrapper to access thepurchaseManager
.struct ChildView: View { @EnvironmentObject var purchaseManager: PurchaseManager var body: some View { // Use purchaseManager here } }
This way, the purchaseManager
state variable is passed down to the child view as an environment object, allowing the child view to observe and react to changes in purchaseManager
.
For more details on state management in SwiftUI, you can refer to the SwiftUI essentials session from WWDC 2024.
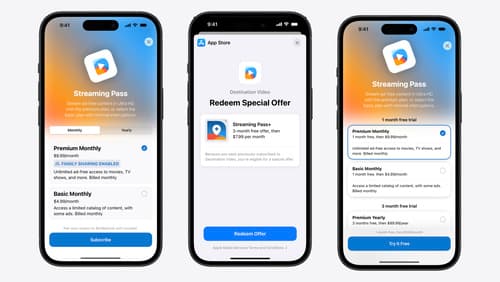
What’s new in StoreKit and In-App Purchase
Learn how to build and deliver even better purchase experiences using the App Store In-App Purchase system. We’ll demo new StoreKit views control styles and new APIs to improve your subscription customization, discuss new fields for transaction-level information, and explore new testability in Xcode. We’ll also review an important StoreKit deprecation.
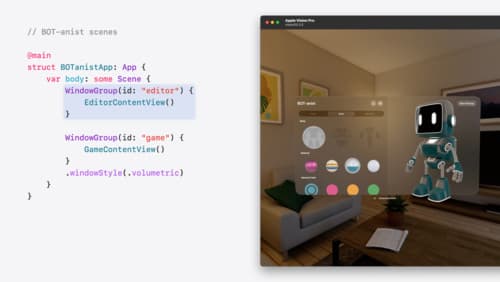
Work with windows in SwiftUI
Learn how to create great single and multi-window apps in visionOS, macOS, and iPadOS. Discover tools that let you programmatically open and close windows, adjust position and size, and even replace one window with another. We’ll also explore design principles for windows that help people use your app within their workflows.
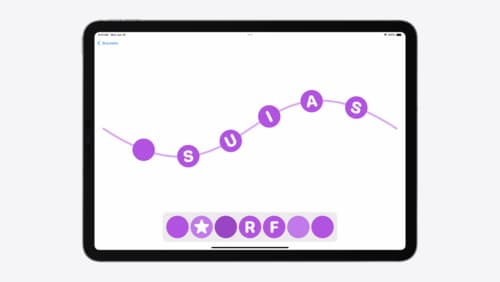
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.