read raw piped data into cli application
Generated on 8/1/2024
1 search
To read raw piped data into a CLI application, you can leverage the Swift ArgumentParser library to handle command-line arguments and input. Here’s a brief overview of how you might approach this:
- Define Your Command: Create a struct conforming to
ParsableCommand
to define your CLI command. - Handle Input: Use the
stdin
to read raw piped data. - Process Data: Implement the logic to process the input data as needed.
Here’s a simple example:
import ArgumentParser
struct MyCLI: ParsableCommand {
@Argument(help: "The name of the user.")
var username: String
func run() throws {
// Read from stdin
let inputData = FileHandle.standardInput.readDataToEndOfFile()
if let inputString = String(data: inputData, encoding: .utf8) {
// Process the input data
print("Received input: \(inputString)")
} else {
print("Failed to read input")
}
}
}
MyCLI.main()
This example sets up a basic CLI tool that reads raw data from standard input and prints it. You can expand this to include more complex processing as needed.
For more detailed information on building CLI tools with Swift, you might find the session A Swift Tour: Explore Swift’s features and design useful, particularly the section on argument parsing and command-line tools.
Additionally, if you are working with server-side Swift, the session Explore the Swift on Server ecosystem covers building services and handling inputs, which might provide further insights.
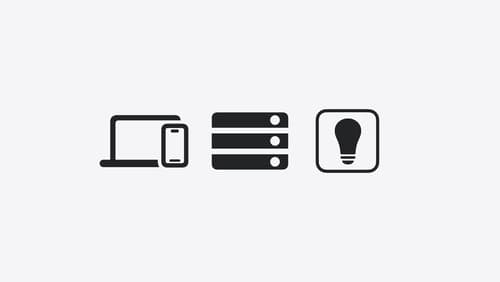
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
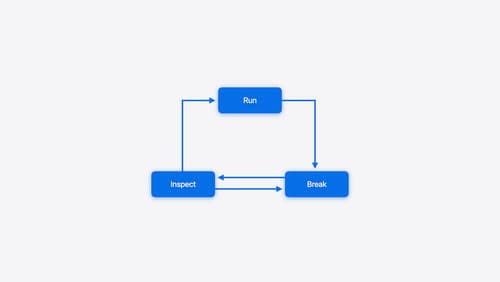
Run, Break, Inspect: Explore effective debugging in LLDB
Learn how to use LLDB to explore and debug codebases. We’ll show you how to make the most of crashlogs and backtraces, and how to supercharge breakpoints with actions and complex stop conditions. We’ll also explore how the “p” command and the latest features in Swift 6 can enhance your debugging experience.
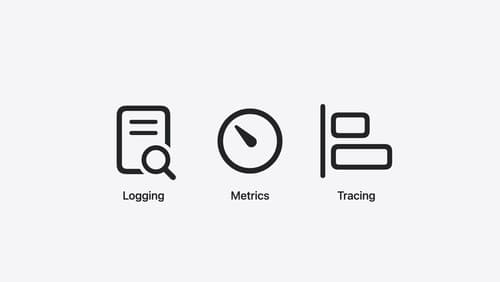
Explore the Swift on Server ecosystem
Swift is a great language for writing your server applications, and powers critical services across Apple’s cloud products. We’ll explore tooling, delve into the Swift server package ecosystem, and demonstrate how to interact with databases and add observability to applications.