how can i define a TextEditor with word wrap and max 4 lines of its content visible?
Asked on 08/01/2024
1 search
To define a TextEditor
in SwiftUI with word wrap and a maximum of 4 lines of its content visible, you can use the .frame
modifier to limit the height of the TextEditor
. Here is an example:
import SwiftUI
struct ContentView: View {
@State private var text: String = ""
var body: some View {
TextEditor(text: $text)
.frame(maxHeight: 100) // Adjust the height as needed to fit 4 lines
.lineLimit(4) // This ensures the TextEditor does not grow beyond 4 lines
.padding()
.border(Color.gray, width: 1)
}
}
In this example:
- The
.frame(maxHeight: 100)
limits the height of theTextEditor
. You may need to adjust the height value to fit exactly 4 lines based on your font size and line spacing. - The
.lineLimit(4)
ensures that theTextEditor
does not grow beyond 4 lines.
For more detailed information on text handling and customization, you can refer to the session Get started with Writing Tools at WWDC 2024.
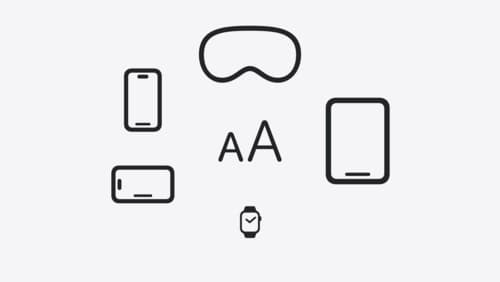
Get started with Dynamic Type
Dynamic Type lets people choose their preferred text size across the system and all of their apps. To help you get started supporting Dynamic Type, we’ll cover the fundamentals: How it works, how to find issues with scaling text in your app, and how to take practical steps using SwiftUI and UIKit to create a great Dynamic Type experience. We’ll also show how you can best use the Large Content Viewer to make navigation controls accessible to everyone.
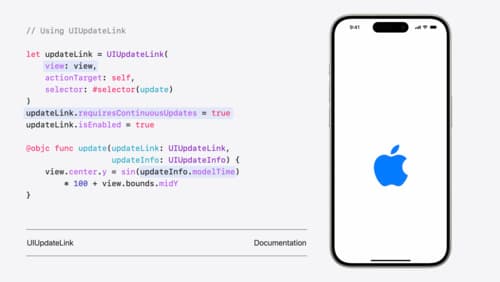
What’s new in UIKit
Explore everything new in UIKit, including tab and document launch experiences, transitions, and text and input changes. We’ll also discuss better-than-ever interoperability between UIKit and SwiftUI animations and gestures, as well as general improvements throughout UIKit.
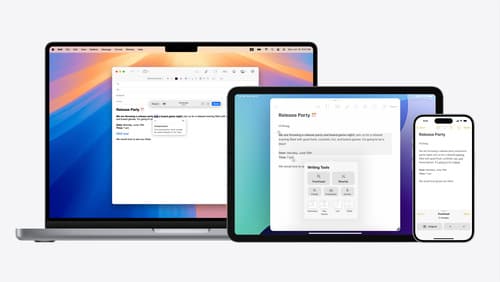
Get started with Writing Tools
Learn how Writing Tools help users proofread, rewrite, and transform text in your app. Get the details on how Writing Tools interact with your app so users can refine what they have written in any text view. Understand how text is retrieved and processed, and how to support Writing Tools in custom text views.