how do I download a file asynchronously
Asked on 08/01/2024
1 search
To download a file asynchronously in Swift, you can use the async
and await
keywords, which are part of Swift's concurrency model. Here's a basic example of how you might do this:
- Define an async function to download the file:
import Foundation
func downloadFile(from url: URL) async throws -> Data {
let (data, _) = try await URLSession.shared.data(from: url)
return data
}
- Call the async function:
Since async
functions can only be called from other async
functions or from a context that supports concurrency, you might call this function from within an async
context, such as within a SwiftUI view or another async function.
import SwiftUI
struct ContentView: View {
@State private var fileData: Data?
var body: some View {
VStack {
if let fileData = fileData {
Text("File downloaded: \(fileData.count) bytes")
} else {
Text("Downloading file...")
.onAppear {
Task {
do {
let url = URL(string: "https://example.com/file")!
fileData = try await downloadFile(from: url)
} catch {
print("Failed to download file: \(error)")
}
}
}
}
}
}
}
Additional Context from WWDC Sessions
-
Concurrency in Swift: The
async
andawait
keywords are used to handle asynchronous operations in Swift. When an async function is called, theawait
keyword indicates that a suspension can occur on that line. This allows the CPU to perform other tasks while waiting for the asynchronous operation to complete. For more details, you can refer to the session A Swift Tour: Explore Swift’s features and design (18:50). -
Handling Asynchronous Conditions in Testing: If you are testing asynchronous code, you can use
withCheckedContinuation
orwithCheckedThrowingContinuation
to convert completion handlers to async/await syntax. This is useful for ensuring that your tests can properly await the completion of asynchronous operations. For more information, see the session Go further with Swift Testing (24:26).
Relevant Sessions
If you need more specific details or examples, feel free to ask!
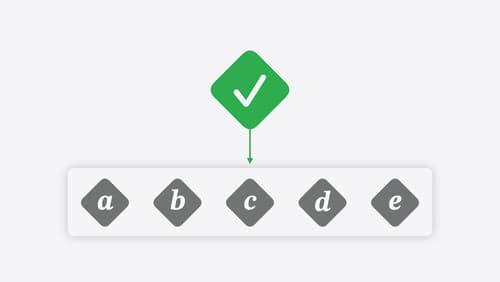
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
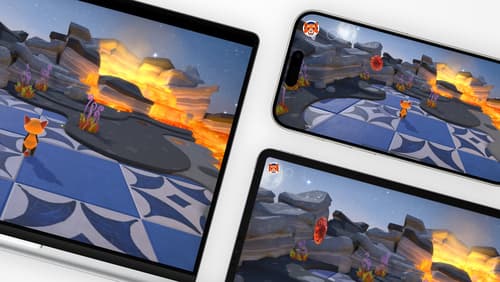
Design advanced games for Apple platforms
Learn how to adapt your high-end game so it feels at home on Mac, iPad, and iPhone. We’ll go over how to make your game look stunning on different displays, tailor your input and controls to be intuitive on each device, and take advantage of Apple technologies that deliver great player experiences.
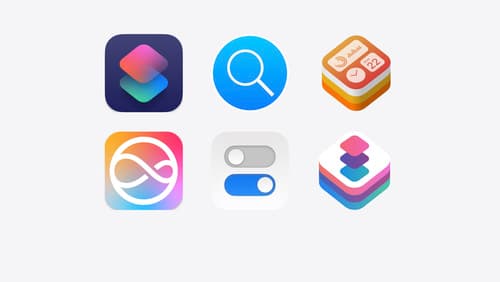
What’s new in App Intents
Learn about improvements and all-new features with App Intents, and discover how this framework can help you expose your app’s functionality to Siri, Spotlight, Shortcuts, and more. We’ll show you how to make your entities more meaningful to the platform with the Transferable API, File Representations, new IntentFile APIs, and Spotlight Indexing, opening up powerful functionality in Siri and the Shortcuts app. Empower your intents to take people deep into your app with URL Representable Entities. Explore new techniques to model your entities and intents with new APIs for error handling and union values.