how do I create an async context in swift
Generated on 8/1/2024
1 search
To create an async context in Swift, you can use the async
and await
keywords. Here's a brief overview of how you can do this:
- Marking a Function as Async: A function that may suspend is marked with the
async
keyword. - Awaiting an Async Function: When an async function is called, the
await
keyword is used to indicate that a suspension can occur on that line.
Here's an example of how you might use these features in a Swift function:
import Foundation
// Define an async function
func fetchData(from url: URL) async throws -> Data {
let (data, _) = try await URLSession.shared.data(from: url)
return data
}
// Call the async function
Task {
do {
let url = URL(string: "https://example.com")!
let data = try await fetchData(from: url)
print("Data received: \(data)")
} catch {
print("Failed to fetch data: \(error)")
}
}
In this example:
fetchData(from:)
is an async function that fetches data from a URL.- The
await
keyword is used to callfetchData(from:)
within aTask
.
For more detailed information on Swift's concurrency features, you can refer to the session A Swift Tour: Explore Swift’s features and design at WWDC 2024, which covers the basics of writing concurrent code in Swift, including tasks, async/await, and actors.
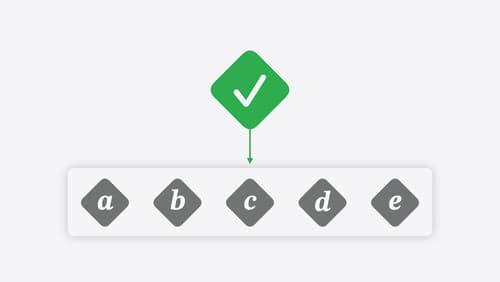
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
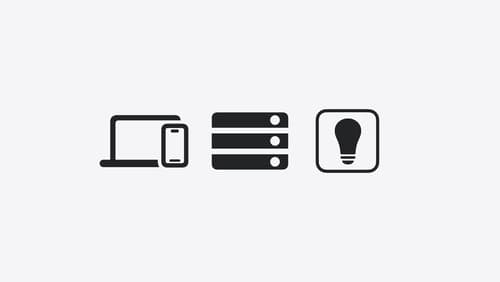
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
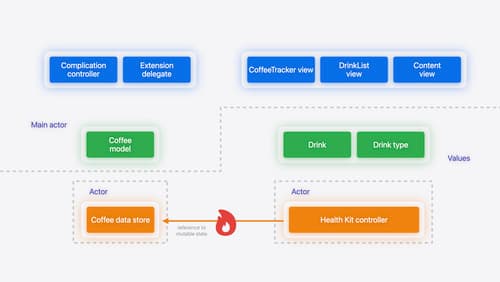
Migrate your app to Swift 6
Experience Swift 6 migration in action as we update an existing sample app. Learn how to migrate incrementally, module by module, and how the compiler helps you identify code that’s at risk of data races. Discover different techniques for ensuring clear isolation boundaries and eliminating concurrent access to shared mutable state.