reusable code
Asked on 08/01/2024
1 search
To write reusable code in Swift, you can leverage several techniques and features discussed in various WWDC sessions. Here are some key points:
-
Generics:
- Generics allow you to write flexible and reusable functions and types that can work with any type. For example, you can create a method that works with any collection type by using generics.
- Example from A Swift Tour: Explore Swift’s features and design: "I'll extend collection to add a method called uniqued. The implementation of uniqued should be simple. I can first create a set with the contents of the collection and then convert that set back into an array."
-
Parameterized Testing:
- Parameterized tests help you avoid code duplication in your test cases by allowing a single test function to run with different inputs.
- Example from Meet Swift Testing: "Instead, we can write all of these as a single test using a feature called parameterized testing. Let's transform this first test into a parameterized one."
-
Noncopyable Types:
- Using noncopyable types can help you write safer and more efficient code by preventing unintended copies of objects.
- Example from Consume noncopyable types in Swift: "Let's start with our runnable protocol from earlier. Our universe of types currently looks like this all runnable values are copyable. Bank transfer is not copyable, so it cannot be runnable either, but I want banktransfer to conform so I can use it generically."
-
Code Templates and Multi-Cursor Editing:
- Xcode provides features like code templates and multi-cursor editing to help you write repetitive code more efficiently.
- Example from Xcode essentials: "If you need to put similar code into several places, you can create a template with the same placeholders you see in code completion by surrounding text with these characters."
Relevant Sessions
- Consume noncopyable types in Swift
- Go further with Swift Testing
- Xcode essentials
- Meet Swift Testing
- A Swift Tour: Explore Swift’s features and design
These sessions provide a comprehensive overview of techniques and tools you can use to write reusable and maintainable code in Swift.
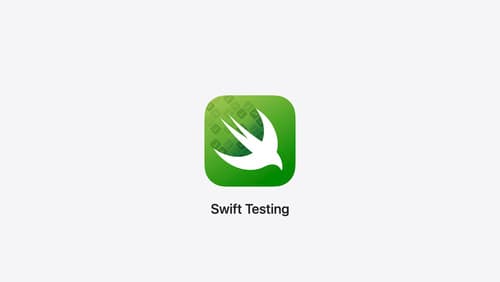
Meet Swift Testing
Introducing Swift Testing: a new package for testing your code using Swift. Explore the building blocks of its powerful new API, discover how it can be applied in common testing workflows, and learn how it relates to XCTest and open source Swift.
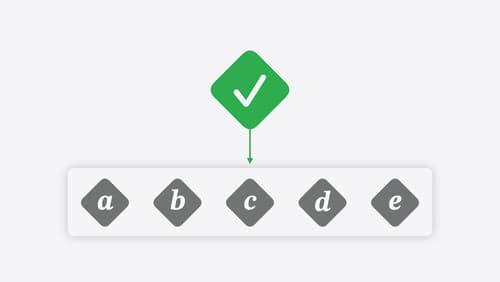
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.
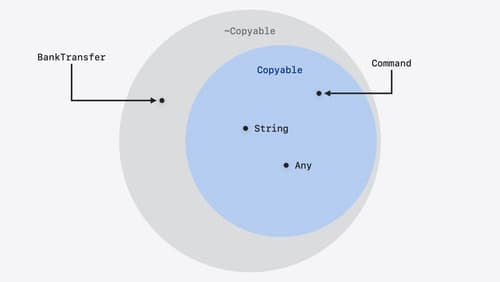
Consume noncopyable types in Swift
Get started with noncopyable types in Swift. Discover what copying means in Swift, when you might want to use a noncopyable type, and how value ownership lets you state your intentions clearly.