How do I use a mesh gradient?
Generated on 8/1/2024
1 search
To use a mesh gradient in SwiftUI, you can follow these steps:
-
Define the Grid: Start by defining the rows and columns of your grid using the width and height parameters. For example, you might use a 3x3 grid.
-
Set Coordinates: Define the x and y coordinates on this grid. Points in the grid are defined using
simd_float2
values, which take a value from 0 to 1 on the x and y axes. -
Assign Colors: Add a corresponding color for each of these points. SwiftUI will interpolate between these colors to create a smooth gradient.
-
Adjust Control Points: You can move the control points to create different visual effects. The colors will move to match the new positions of these points.
-
Experiment: Play around with the values like the position of control points, grid size, and color palette to create unique visual effects.
Here's a brief example of how you might set this up in code:
import SwiftUI
struct MeshGradientView: View {
var body: some View {
MeshGradient(
colors: [
.red, .green, .blue,
.yellow, .purple, .orange,
.pink, .gray, .cyan
],
grid: (rows: 3, columns: 3),
points: [
simd_float2(0, 0), simd_float2(0.5, 0), simd_float2(1, 0),
simd_float2(0, 0.5), simd_float2(0.5, 0.5), simd_float2(1, 0.5),
simd_float2(0, 1), simd_float2(0.5, 1), simd_float2(1, 1)
]
)
.frame(width: 300, height: 300)
}
}
For more detailed information, you can refer to the Create custom visual effects with SwiftUI session at the 07:10 mark.
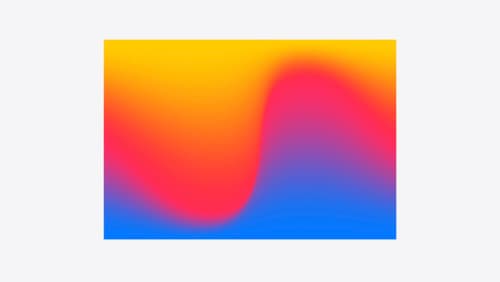
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.
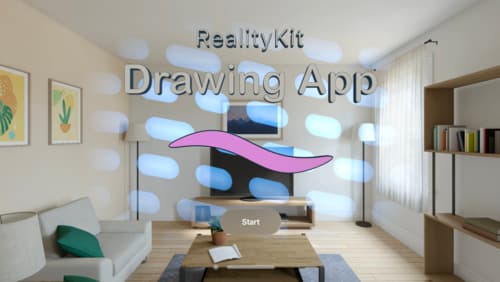
Build a spatial drawing app with RealityKit
Harness the power of RealityKit through the process of building a spatial drawing app. As you create an eye-catching spatial experience that integrates RealityKit with ARKit and SwiftUI, you’ll explore how resources work in RealityKit and how to use features like low-level mesh and texture APIs to achieve fast updates of the users’ brush strokes.