How to implement animated transitions
Generated on 8/2/2024
1 search
To implement animated transitions in your app, you can leverage the information from the following sessions presented at WWDC:
- Create custom visual effects with SwiftUI
- Enhance your UI animations and transitions
Using SwiftUI for Custom Transitions
In the session Create custom visual effects with SwiftUI, you can learn how to create custom transitions by conforming to the Transition
protocol. Here's a brief overview:
-
Define a Custom Transition:
- Create a new struct that conforms to the
Transition
protocol. - Implement the
transition
body function, which takescontent
andphase
parameters. - Use the
phase
value to conditionally style your view.
struct Twirl: Transition { func body(content: Content, phase: TransitionPhase) -> some View { content .scaleEffect(phase == .active ? 1.0 : 0.5) .opacity(phase == .active ? 1.0 : 0.0) .rotationEffect(Angle(degrees: phase == .active ? 0 : -360)) .brightness(phase == .active ? 0 : -0.5) } }
- Create a new struct that conforms to the
-
Apply the Custom Transition:
- Attach your custom transition to your view.
.transition(Twirl())
For more details, you can refer to the session Create custom visual effects with SwiftUI (10:13).
Using UIKit for Transitions
In the session Enhance your UI animations and transitions, you can learn about integrating SwiftUI animations with UIKit and AppKit views:
-
Zoom Transitions:
- Use the new zoom transition to enhance visual continuity in your app.
- Add the
navigationTransitionStyle
modifier to specify the zoom transition.
.navigationTransitionStyle(.zoom)
-
Bridging Animations:
- Use the new
animate
method on the context to bridge animations between SwiftUI and UIKit.
context.animate { UIView.animate(withDuration: 0.3) { // Your UIKit animation code } }
- Use the new
For more details, you can refer to the session Enhance your UI animations and transitions (09:49).
Additional Tips
- Combining Transitions: You can chain multiple transitions using the
combined
method. - Interactive Animations: Ensure your code is ready to handle transitions starting at any time, especially for continuously interactive transitions like the zoom transition.
For a comprehensive understanding, you can explore the chapters in the sessions mentioned above:
-
Enhance your UI animations and transitions
-
Create custom visual effects with SwiftUI
By following these guidelines and exploring the sessions, you can effectively implement animated transitions in your app.
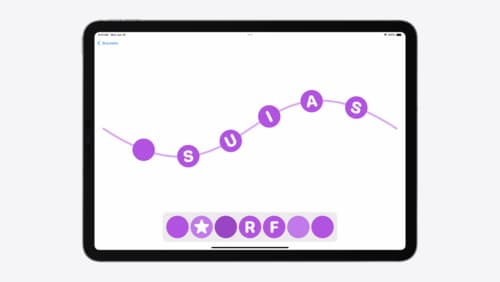
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.
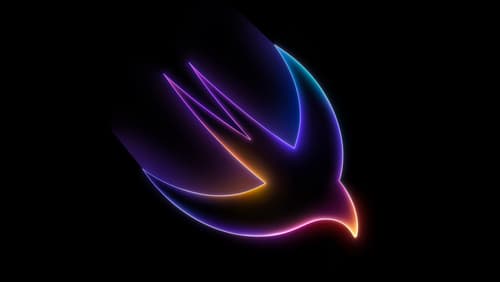
Platforms State of the Union
Discover the newest advancements on Apple platforms.
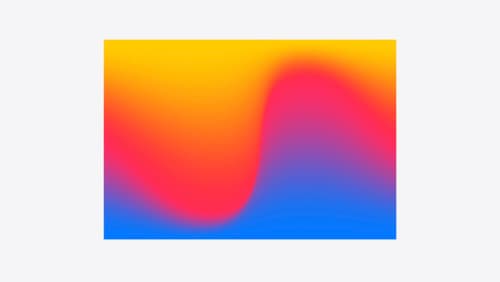
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.