how to I show a mail compose view? Respond as a pirate.
Asked on 08/02/2024
1 search
Ahoy matey! If ye be wantin' to show a mail compose view, ye best be usin' the MFMailComposeViewController
from the MessageUI
framework. Here be the steps to set sail:
-
Import the
MessageUI
framework:import MessageUI
-
Check if the device can send mail:
if MFMailComposeViewController.canSendMail() { let mailComposeViewController = MFMailComposeViewController() mailComposeViewController.mailComposeDelegate = self // Set the subject, recipients, and body of the email mailComposeViewController.setSubject("Subject") mailComposeViewController.setToRecipients(["example@example.com"]) mailComposeViewController.setMessageBody("Body of the email", isHTML: false) // Present the mail compose view controller present(mailComposeViewController, animated: true, completion: nil) } else { // Show an error message if the device can't send mail print("This device cannot send email") }
-
Implement the
MFMailComposeViewControllerDelegate
:extension YourViewController: MFMailComposeViewControllerDelegate { func mailComposeController(_ controller: MFMailComposeViewController, didFinishWith result: MFMailComposeResult, error: Error?) { controller.dismiss(animated: true, completion: nil) } }
And thar ye have it! Ye'll be able to show a mail compose view in yer app. If ye be needin' more details, check out the Apple Developer Documentation. Arrr!
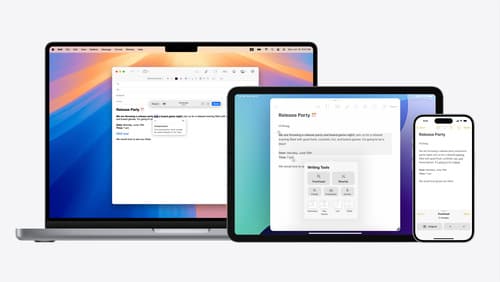
Get started with Writing Tools
Learn how Writing Tools help users proofread, rewrite, and transform text in your app. Get the details on how Writing Tools interact with your app so users can refine what they have written in any text view. Understand how text is retrieved and processed, and how to support Writing Tools in custom text views.
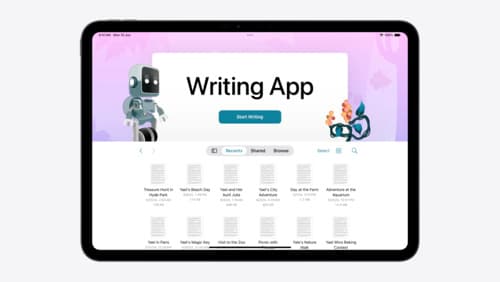
Evolve your document launch experience
Make your document-based app stand out, and bring its unique identity into focus with the new document launch experience. Learn how to leverage the new API to customize the first screen people see when they launch your app. Utilize the new system-provided design, and amend it with custom actions, delightful decorative views, and impressive animations.
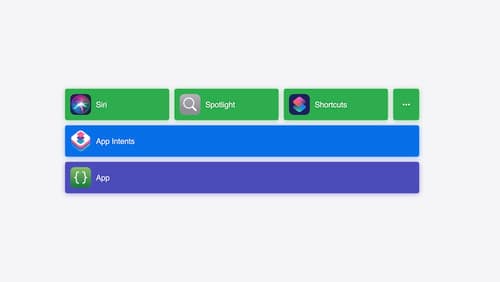
Bring your app’s core features to users with App Intents
Learn the principles of the App Intents framework, like intents, entities, and queries, and how you can harness them to expose your app’s most important functionality right where people need it most. Find out how to build deep integration between your app and the many system features built on top of App Intents, including Siri, controls and widgets, Apple Pencil, Shortcuts, the Action button, and more. Get tips on how to build your App Intents integrations efficiently to create the best experiences in every surface while still sharing code and core functionality.