How to implement a binding in SwiftUI?
Asked on 08/02/2024
1 search
To implement a binding in SwiftUI, you can follow these steps:
-
Define a State Property: In the parent view, define a state property that will hold the value you want to bind.
struct ParentView: View { @State private var rating: Int = 0 var body: some View { RatingView(rating: $rating) } }
-
Create a Binding: Pass the state property to the child view using the
$
prefix to create a binding.struct RatingView: View { @Binding var rating: Int var body: some View { VStack { Text("Rating: \(rating)") Button("Increase Rating") { rating += 1 } } } }
In this example, ParentView
has a state property rating
, and it passes a binding to RatingView
. The RatingView
can then read and modify the rating
value, and any changes will be reflected back in the ParentView
.
For more details, you can refer to the SwiftUI essentials session at WWDC 2024, which discusses how to use bindings to create a two-way reference to the state of another view.
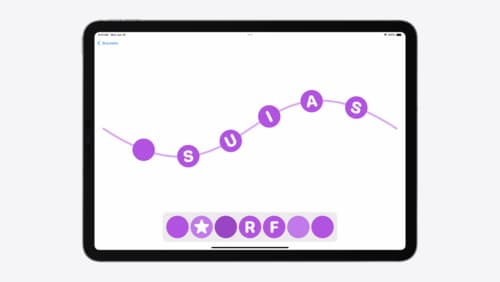
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.
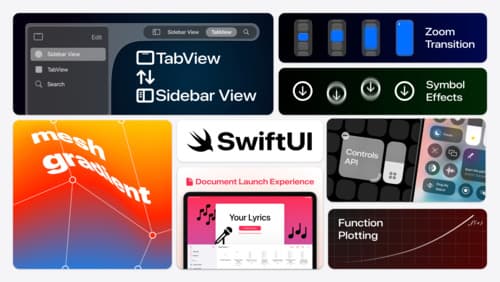
What’s new in SwiftUI
Learn how you can use SwiftUI to build great apps for any Apple platform. Explore a fresh new look and feel for tabs and documents on iPadOS. Improve your window management with new windowing APIs, and gain more control over immersive spaces and volumes in your visionOS apps. We’ll also take you through other exciting refinements that help you make expressive charts, customize and layout text, and so much more.
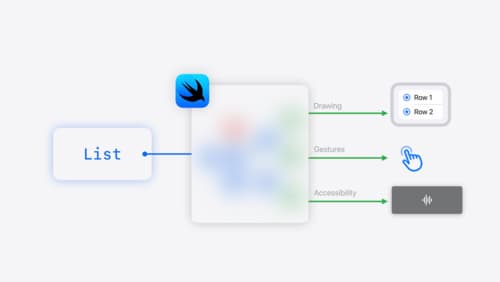
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.