Diagnose bad_ex
Generated on 8/2/2024
1 search
To diagnose the bad_ex
error, you can leverage several debugging and testing techniques discussed in various WWDC sessions. Here are some relevant points and sessions that might help:
-
Using Breakpoints in Xcode:
- You can set a Swift error breakpoint to stop immediately where any error is thrown. This can help you identify the exact location and context of the error.
- For more details, refer to the Xcode essentials session, specifically the chapter on "Setting breakpoints".
-
Inspecting Program State with LLDB:
- Utilize LLDB's backtrace feature to understand the sequence of function calls leading to the error. This can help you trace back to the root cause.
- For more information, see the Run, Break, Inspect: Explore effective debugging in LLDB session, particularly the chapter on "Crashlogs & starting the program".
-
Handling Known Issues in Tests:
- If the error is related to a known issue that you cannot immediately fix, you can use the
with known issue
function to document it and prevent it from obscuring other test results. - This is covered in the Go further with Swift Testing session, under the chapter "Tests with known issues".
- If the error is related to a known issue that you cannot immediately fix, you can use the
-
Error Handling in Swift:
- Ensure that your code handles errors gracefully and that you have tests in place to verify both success and failure cases.
- Refer to the Go further with Swift Testing session, especially the chapter on "Expectations".
By combining these techniques, you should be able to diagnose and address the bad_ex
error more effectively. If you need more specific guidance on any of these points, feel free to ask!
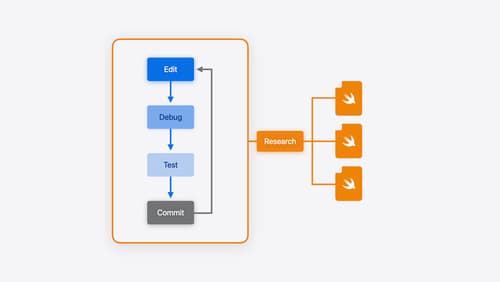
Xcode essentials
Edit, debug, commit, repeat. Explore the suite of tools in Xcode that help you iterate quickly when developing apps. Discover tips and tricks to help optimize and boost your development workflow.
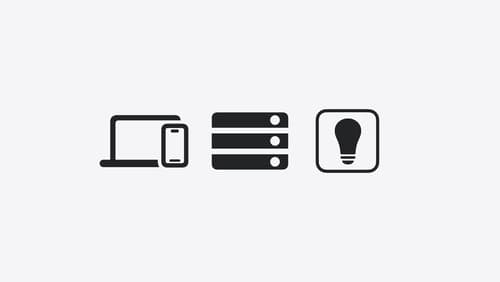
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
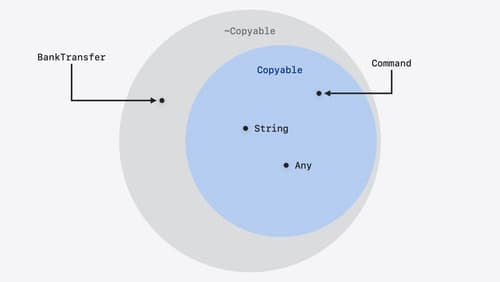
Consume noncopyable types in Swift
Get started with noncopyable types in Swift. Discover what copying means in Swift, when you might want to use a noncopyable type, and how value ownership lets you state your intentions clearly.
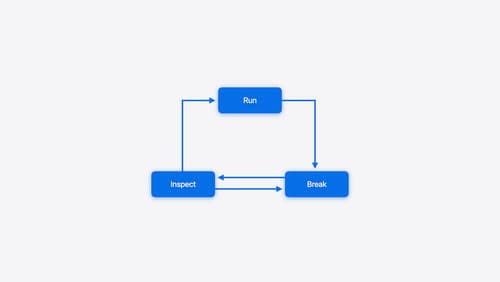
Run, Break, Inspect: Explore effective debugging in LLDB
Learn how to use LLDB to explore and debug codebases. We’ll show you how to make the most of crashlogs and backtraces, and how to supercharge breakpoints with actions and complex stop conditions. We’ll also explore how the “p” command and the latest features in Swift 6 can enhance your debugging experience.
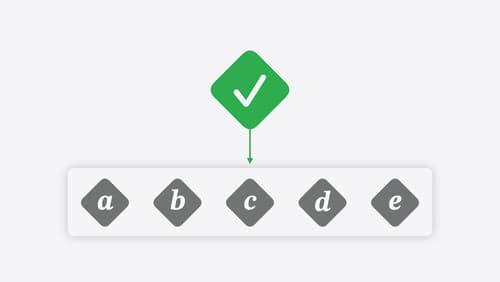
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.