How do I support consuming SPM packages in CocoaPods dependencies?
Asked on 08/02/2024
1 search
To support consuming Swift Package Manager (SPM) packages in CocoaPods dependencies, you can follow these general steps:
-
Create a Swift Package: First, you need to create a Swift package that includes the dependencies you want to use. You can do this using the Swift Package Manager from the command line or within Xcode.
-
Add Dependencies: In your Swift package, add the dependencies you need by specifying them in the
Package.swift
file. For example:dependencies: [ .package(url: "https://github.com/apple/swift-argument-parser", from: "1.0.0"), ],
-
Build the Package: Use the Swift Package Manager to build and test your package to ensure everything is working correctly. You can do this from the command line:
swift build swift test
-
Integrate with CocoaPods: To use the Swift package in a CocoaPods project, you can create a podspec file for your Swift package. This file describes the package and its dependencies. Here is an example of a simple podspec file:
Pod::Spec.new do |s| s.name = 'MySwiftPackage' s.version = '1.0.0' s.summary = 'A short description of MySwiftPackage.' s.description = <<-DESC A longer description of MySwiftPackage. DESC s.homepage = 'https://example.com/MySwiftPackage' s.license = { :type => 'MIT', :file => 'LICENSE' } s.author = { 'Author' => 'author@example.com' } s.source = { :git => 'https://github.com/username/MySwiftPackage.git', :tag => s.version.to_s } s.source_files = 'Sources/**/*.swift' s.swift_version = '5.0' s.dependency 'SwiftPackageDependency', '~> 1.0' end
-
Publish the Pod: Once your podspec file is ready, you can publish your pod to the CocoaPods repository. This will make it available for other projects to use.
For more detailed guidance, you can refer to the Swift Package Manager documentation and the CocoaPods guides.
Relevant Sessions from WWDC
These sessions provide insights into Swift Package Manager, Swift 6, and other related topics that might be useful for your integration.
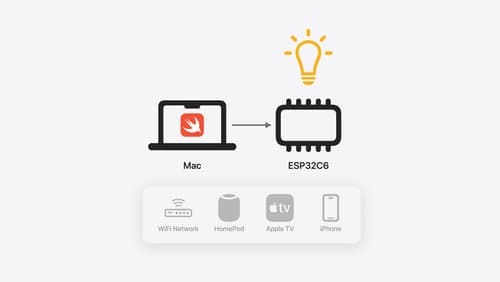
Go small with Embedded Swift
Embedded Swift brings the safety and expressivity of Swift to constrained environments. Explore how Embedded Swift runs on a variety of microcontrollers through a demonstration using an off-the-shelf Matter device. Learn how the Embedded Swift subset packs the benefits of Swift into a tiny footprint with no runtime, and discover plenty of resources to start your own Embedded Swift adventure.
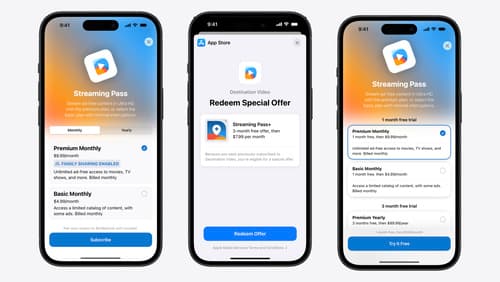
What’s new in StoreKit and In-App Purchase
Learn how to build and deliver even better purchase experiences using the App Store In-App Purchase system. We’ll demo new StoreKit views control styles and new APIs to improve your subscription customization, discuss new fields for transaction-level information, and explore new testability in Xcode. We’ll also review an important StoreKit deprecation.
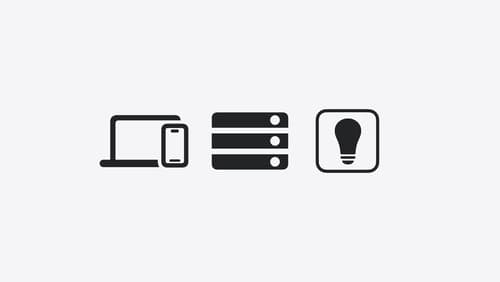
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.