How can I make my code faster?
Generated on 8/2/2024
1 search
To make your code faster, you can consider several strategies and tools presented at WWDC:
-
Concurrency:
- Use concurrency to process tasks in parallel. For example, when processing multiple images, instead of processing them one at a time in a loop, you can use task groups to handle multiple tasks simultaneously. This approach can significantly speed up your code. You can limit the number of concurrent tasks to manage memory usage effectively. This was discussed in the session Discover Swift enhancements in the Vision framework.
-
Flame Graphs in Xcode:
- Utilize the new flame graph feature in Xcode 16 to identify performance bottlenecks in your code. The flame graph provides a high-level overview of trace execution, helping you spot issues quickly. For instance, if a function is taking up most of the execution time, you can parallelize the loading using a task group and move the execution to the background. This was highlighted in the session What’s new in Xcode 16.
-
Low-Level Performance Optimization:
- Focus on low-level performance considerations such as optimizing function calls, memory allocation, and value copying. Swift has a powerful optimizer, but the way you write your code can impact its effectiveness. Regularly monitor performance and automate measurements to identify regressions. This was covered in detail in the session Explore Swift performance.
-
Memory Management:
- Reduce reference counting overhead by enabling whole module optimization, profiling for expensive struct copies, and minimizing the use of reference types. Avoid circumventing ARC (Automatic Reference Counting) as it can lead to hard-to-debug memory leaks. These tips were discussed in the session Analyze heap memory.
-
Profiling and Debugging Tools:
- Use profiling tools to identify and optimize the most expensive parts of your code. For example, in game development, you can use performance heat maps to visualize and optimize shader performance. This was demonstrated in the session Port advanced games to Apple platforms.
By leveraging these strategies and tools, you can significantly improve the performance of your code.
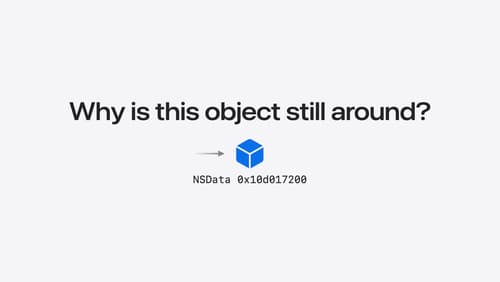
Analyze heap memory
Dive into the basis for your app’s dynamic memory: the heap! Explore how to use Instruments and Xcode to measure, analyze, and fix common heap issues. We’ll also cover some techniques and best practices for diagnosing transient growth, persistent growth, and leaks in your app.
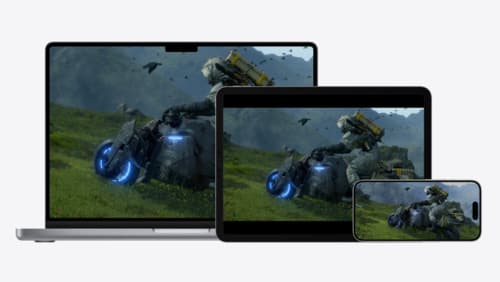
Port advanced games to Apple platforms
Discover how simple it can be to reach players on Apple platforms worldwide. We’ll show you how to evaluate your Windows executable on Apple silicon, start your game port with code samples, convert your shader code to Metal, and bring your game to Mac, iPhone, and iPad. Explore enhanced Metal tools that understand HLSL shaders to validate, debug, and profile your ported shaders on Metal.
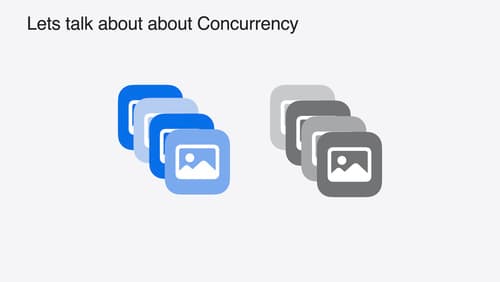
Discover Swift enhancements in the Vision framework
The Vision Framework API has been redesigned to leverage modern Swift features like concurrency, making it easier and faster to integrate a wide array of Vision algorithms into your app. We’ll tour the updated API and share sample code, along with best practices, to help you get the benefits of this framework with less coding effort. We’ll also demonstrate two new features: image aesthetics and holistic body pose.
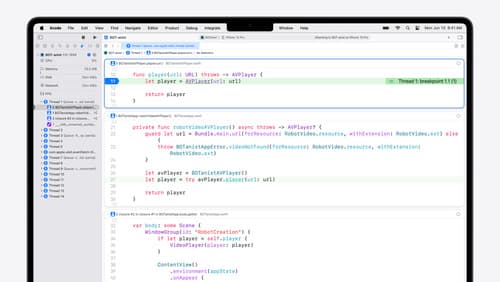
What’s new in Xcode 16
Discover the latest productivity and performance improvements in Xcode 16. Learn about enhancements to code completion, diagnostics, and Xcode Previews. Find out more about updates in builds and explore improvements in debugging and Instruments.
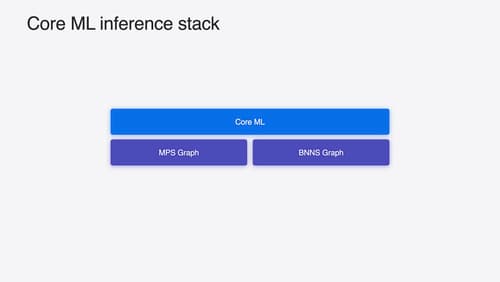
Deploy machine learning and AI models on-device with Core ML
Learn new ways to optimize speed and memory performance when you convert and run machine learning and AI models through Core ML. We’ll cover new options for model representations, performance insights, execution, and model stitching which can be used together to create compelling and private on-device experiences.
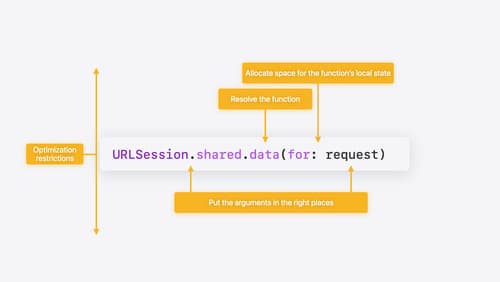
Explore Swift performance
Discover how Swift balances abstraction and performance. Learn what elements of performance to consider and how the Swift optimizer affects them. Explore the different features of Swift and how they’re implemented to further understand the tradeoffs available that can impact performance.