How can I setup state in SwiftUI with a parameter ?
Generated on 8/2/2024
1 search
To set up state in SwiftUI with a parameter, you can use the @State
property wrapper to create an internal source of data for a view. SwiftUI manages the storage and provides it back for the view to read and write. Additionally, you can use @Binding
to create a two-way reference to the state of another view.
Here's an example from the SwiftUI essentials session:
-
Using
@State
:struct RatingView: View { @State private var rating: Int = 0 var body: some View { VStack { Text("Rating: \(rating)") HStack { Button("Increase") { rating += 1 } Button("Decrease") { rating -= 1 } } } } }
-
Using
@Binding
:struct RatingView: View { @Binding var rating: Int var body: some View { VStack { Text("Rating: \(rating)") HStack { Button("Increase") { rating += 1 } Button("Decrease") { rating -= 1 } } } } } struct RatingContainerView: View { @State private var rating: Int = 0 var body: some View { RatingView(rating: $rating) } }
In the example above, RatingView
uses @Binding
to receive a reference to the state from its parent view, RatingContainerView
. This way, the state is managed by the container view, and any changes in the RatingView
will be reflected in the RatingContainerView
.
For more details, you can refer to the SwiftUI essentials session.
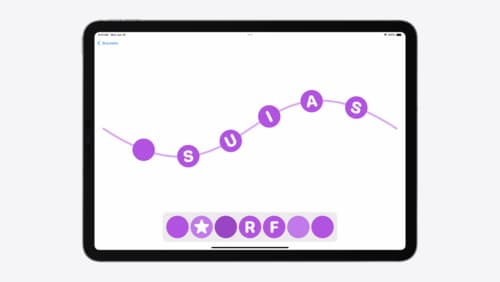
Enhance your UI animations and transitions
Explore how to adopt the zoom transition in navigation and presentations to increase the sense of continuity in your app, and learn how to animate UIKit views with SwiftUI animations to make it easier to build animations that feel continuous.
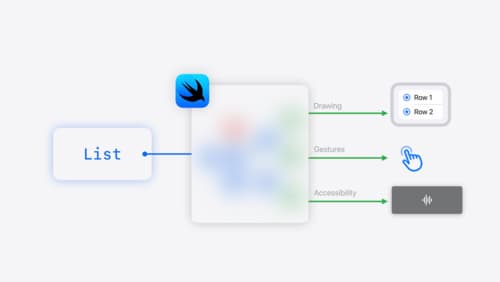
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
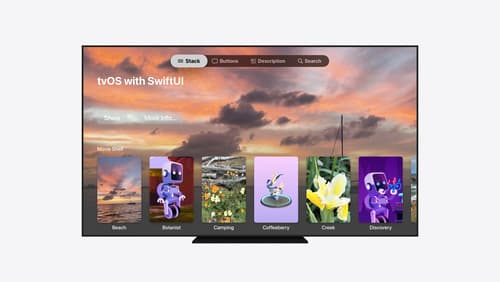
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.