How do I use SwiftData?
Generated on 8/2/2024
1 search
To use SwiftData, you can follow these steps:
-
Import the Framework: Start by importing the SwiftData framework into your project.
-
Define Your Models: Use the
@Model
macro to define your data models. This macro helps in setting up the schema for your data.import SwiftData @Model class Trip { var destination: String var date: Date // Add other properties as needed }
-
Set Up Model Container: Use the
ModelContainer
to manage your models. You can configure it to use different data stores, including custom ones.let container = ModelContainer([Trip.self])
-
Use Queries: Populate your views by querying the model container. This can be done using the
@Query
property wrapper.@Query var trips: [Trip]
-
Custom Data Stores: If you need to use a custom data store, you can configure it by implementing the
DataStore
protocol and passing it to the model container.let customStore = CustomDataStore() let container = ModelContainer([Trip.self], store: customStore)
-
Track Changes: SwiftData provides a history API to track changes in your data, which is useful for syncing with remote servers or handling app extensions.
let history = try container.history()
For a detailed walkthrough, you can refer to the following sessions from WWDC 2024:
- What’s new in SwiftData
- Create a custom data store with SwiftData
- Track model changes with SwiftData history
These sessions cover everything from basic setup to advanced features like custom data stores and tracking changes.
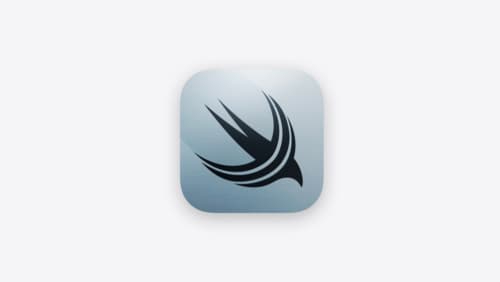
What’s new in SwiftData
SwiftData makes it easy to add persistence to your app with its expressive, declarative API. Learn about refinements to SwiftData, including compound uniqueness constraints, faster queries with #Index, queries in Xcode previews, and rich predicate expressions. Join us to explore how you can use all of these features to express richer models and improve performance in your app. To discover how to build a custom data store or use the history API in SwiftData, watch “Create a custom data store with SwiftData” and “Track model changes with SwiftData history”.
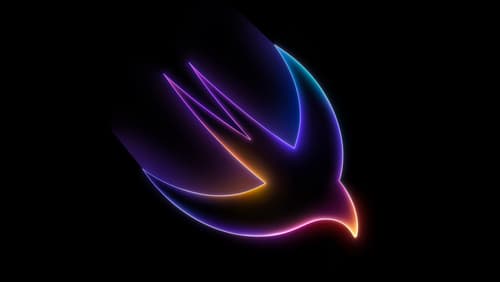
Platforms State of the Union
Discover the newest advancements on Apple platforms.
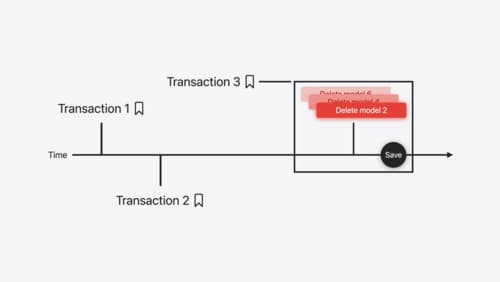
Track model changes with SwiftData history
Reveal the history of your model’s changes with SwiftData! Use the history API to understand when data store changes occurred, and learn how to use this information to build features like remote server sync and out-of-process change handing in your app. We’ll also cover how you can build support for the history API into a custom data store.
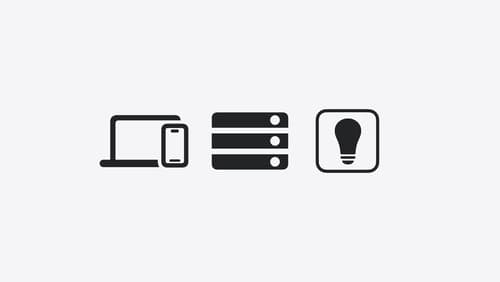
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
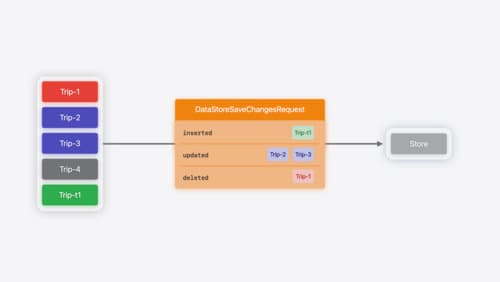
Create a custom data store with SwiftData
Combine the power of SwiftData’s expressive, declarative modeling API with your own persistence backend. Learn how to build a custom data store and explore how to progressively add persistence features in your app. To get the most out of this session, watch “Meet SwiftData” and “Model your schema with SwiftData” from WWDC23.