How to I build a CollectionVIew?
Generated on 8/2/2024
1 search
To build a CollectionView in SwiftUI, you can use a combination of List
, ForEach
, and other container views. Here are some key points and steps to help you get started:
-
Using
List
andForEach
:List
is a powerful container view in SwiftUI that can be used to create a collection of views.ForEach
is used to generate views for each element in a collection and provide those to its container.
struct ContentView: View { let items = ["Item 1", "Item 2", "Item 3"] var body: some View { List { ForEach(items, id: \.self) { item in Text(item) } } } }
This example creates a simple list of text items.
-
Customizing with
ViewBuilder
:- You can use
ViewBuilder
to create more complex and flexible compositions.
struct CustomContainer<Content: View>: View { let content: () -> Content init(@ViewBuilder content: @escaping () -> Content) { self.content = content } var body: some View { VStack { content() } } } struct ContentView: View { var body: some View { CustomContainer { Text("Item 1") Text("Item 2") Text("Item 3") } } }
This example shows how to create a custom container that uses
ViewBuilder
to accept a collection of views. - You can use
-
Advanced Composition:
- You can combine static and dynamic content within the same container using
ForEach
.
struct ContentView: View { let items = ["Item 1", "Item 2", "Item 3"] var body: some View { List { Text("Static Item") ForEach(items, id: \.self) { item in Text(item) } } } }
This example combines a static text item with a dynamic list of items.
- You can combine static and dynamic content within the same container using
-
Sections:
- To organize content into sections, you can use
Section
within aList
.
struct ContentView: View { let items = ["Item 1", "Item 2", "Item 3"] var body: some View { List { Section(header: Text("Section 1")) { ForEach(items, id: \.self) { item in Text(item) } } Section(header: Text("Section 2")) { ForEach(items, id: \.self) { item in Text(item) } } } } }
This example creates a list with two sections, each containing a dynamic list of items.
- To organize content into sections, you can use
For more detailed information and examples, you can refer to the Demystify SwiftUI containers session from WWDC 2024, which covers topics like composition, sections, and customization in SwiftUI containers.
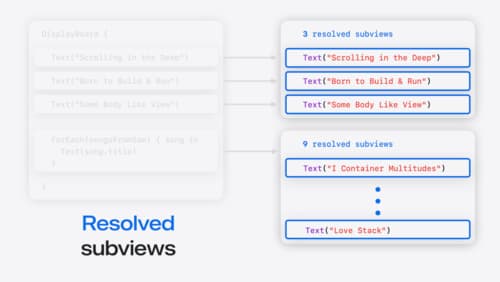
Demystify SwiftUI containers
Learn about the capabilities of SwiftUI container views and build a mental model for how subviews are managed by their containers. Leverage new APIs to build your own custom containers, create modifiers to customize container content, and give your containers that extra polish that helps your apps stand out.
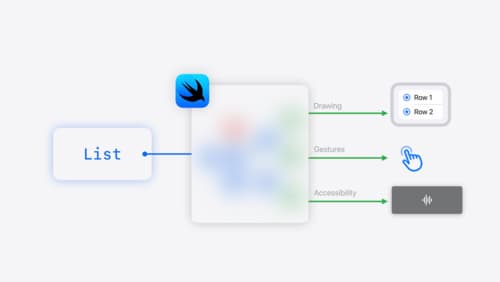
SwiftUI essentials
Join us on a tour of SwiftUI, Apple’s declarative user interface framework. Learn essential concepts for building apps in SwiftUI, like views, state variables, and layout. Discover the breadth of APIs for building fully featured experiences and crafting unique custom components. Whether you’re brand new to SwiftUI or an experienced developer, you’ll learn how to take advantage of what SwiftUI has to offer when building great apps.
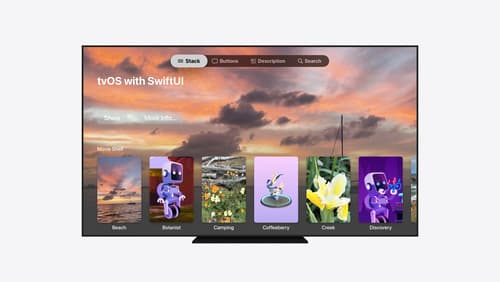
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.