How can I create a metal shader?
Generated on 8/2/2024
1 search
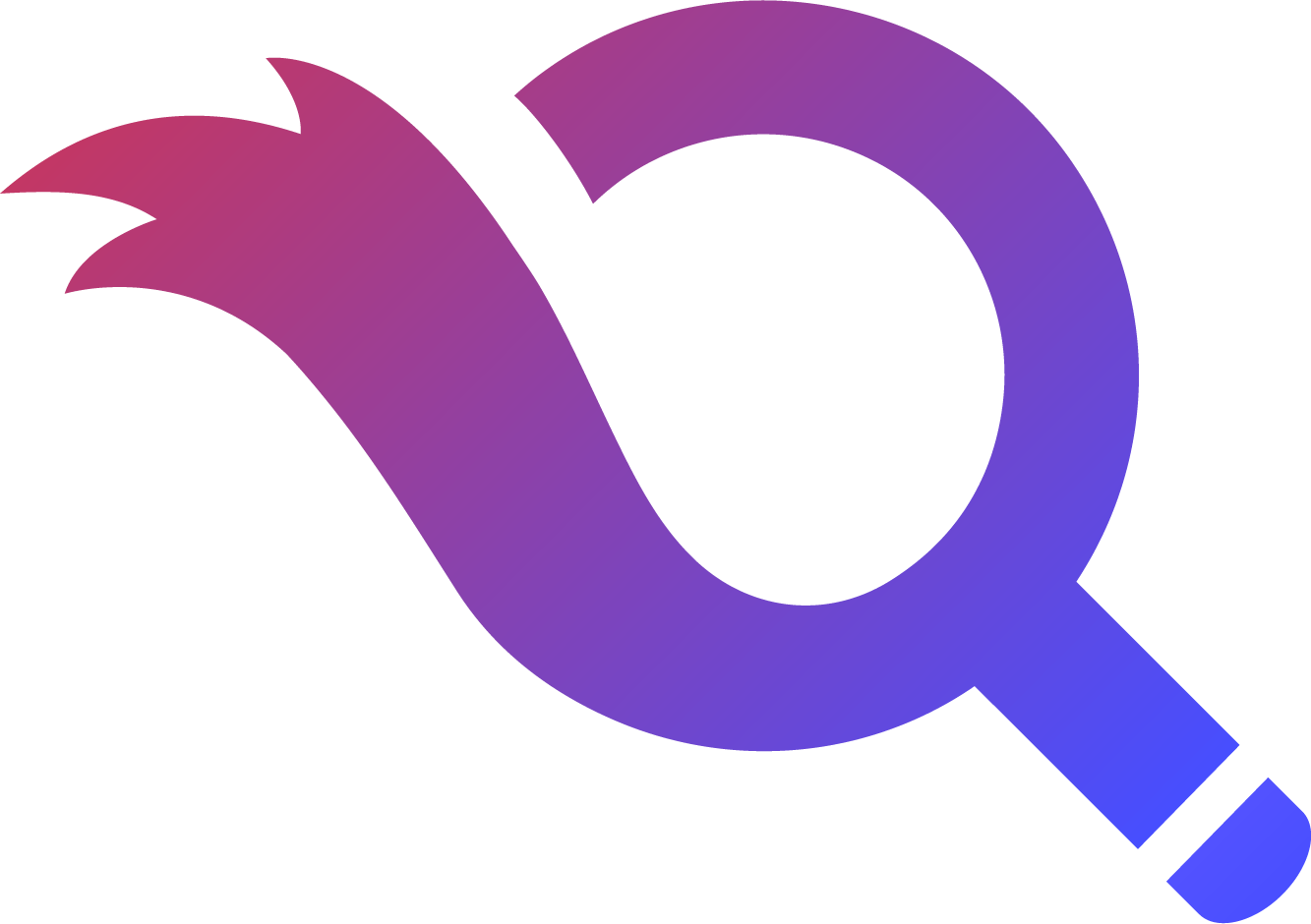
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To create a Metal shader, you can follow these steps:
-
Write the Shader in Metal Shading Language: Metal shaders are written in the Metal Shading Language (MSL). Here is an example of a simple shader function:
#include <metal_stdlib> using namespace metal; kernel void simpleShader(texture2d<float, access::write> outTexture [[texture(0)]], uint2 gid [[thread_position_in_grid]]) { float4 color = float4(1.0, 0.0, 0.0, 1.0); // Red color outTexture.write(color, gid); }
-
Compile the Shader: Use the Metal compiler to compile your shader code. This can be done using Xcode or command-line tools.
-
Integrate with SwiftUI (if applicable): If you are using SwiftUI, you can instantiate and apply the shader to a view. Here’s an example of how to do this:
import SwiftUI struct ContentView: View { var body: some View { Rectangle() .fill(Color.clear) .layerEffect(ShaderLibrary.simpleShader(), maxSampleOffset: .zero) } }
In this example,
ShaderLibrary.simpleShader()
refers to the Metal shader function you defined earlier. -
Use Metal API for Advanced Graphics: For more advanced graphics and compute tasks, you can use the Metal API directly. This involves creating a Metal device, command queue, and other resources to manage and execute your shaders.
For more detailed guidance, you can refer to the following sessions from WWDC:
These sessions provide comprehensive information on creating and using Metal shaders in different contexts.
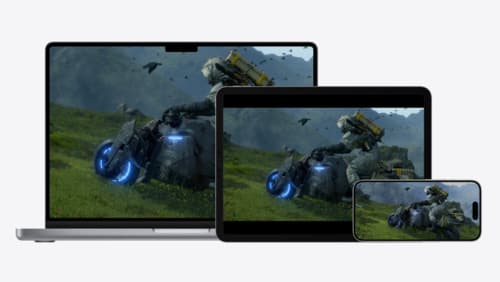
Port advanced games to Apple platforms
Discover how simple it can be to reach players on Apple platforms worldwide. We’ll show you how to evaluate your Windows executable on Apple silicon, start your game port with code samples, convert your shader code to Metal, and bring your game to Mac, iPhone, and iPad. Explore enhanced Metal tools that understand HLSL shaders to validate, debug, and profile your ported shaders on Metal.
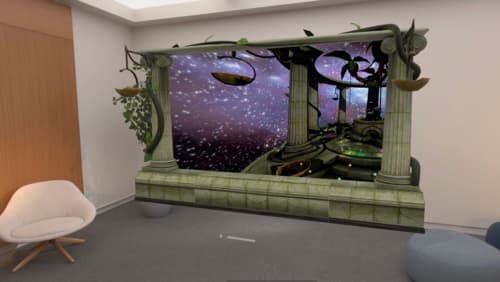
Bring your iOS or iPadOS game to visionOS
Discover how to transform your iOS or iPadOS game into a uniquely visionOS experience. Increase the immersion (and fun factor!) with a 3D frame or an immersive background. And invite players further into your world by adding depth to the window with stereoscopy or head tracking.
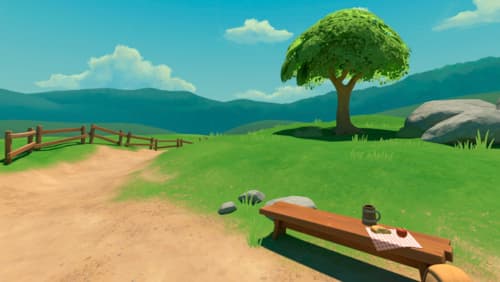
Optimize your 3D assets for spatial computing
Dive into an end-to-end workflow for optimized 3D asset creation. Discover best practices for optimizing meshes, materials, and textures in your digital content creation tool. Learn how to harness shader graph, baking, and material instances to enhance your 3D scene while optimizing performance. Take advantage of native tools to work more effectively with your assets and improve your app’s performance.
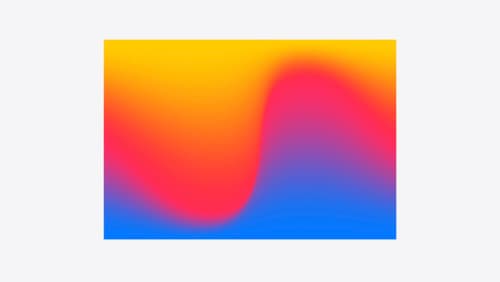
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.