How can I use visualEffect to overlap cells when scrolling?
Asked on 08/02/2024
1 search
To create overlapping cells when scrolling using visual effects in SwiftUI, you can leverage the scrollTransition
and visualEffect
modifiers. These modifiers allow you to adjust the visual properties of your views based on their position within a scroll view.
Here's a step-by-step guide based on the session "Create custom visual effects with SwiftUI":
-
Scroll Transition Modifier:
- Use the
scrollTransition
modifier to change the visual properties of your views as they scroll. This can include properties like offset, scale, fade, and blur. - Example: You can use the y-position of a view to offset, scale, fade, and blur an element as it reaches the top of the scroll view.
- Use the
-
Visual Effect Modifier:
- The
visualEffect
modifier provides access to a content placeholder and a proxy. The proxy gives you the geometry values of the view, which you can use to change visual properties like hue, brightness, saturation, etc. - Example: You can take the location of the view from the proxy and use it to change the hue of your view, creating a gradient effect.
- The
Here is a code snippet to illustrate how you might implement this:
ScrollView {
ForEach(items) { item in
ItemView(item: item)
.scrollTransition { content, proxy in
let yPosition = proxy.frame(in: .global).minY
content
.offset(y: yPosition * 0.1)
.scaleEffect(1 - (yPosition / 1000))
.opacity(1 - (yPosition / 500))
.blur(radius: yPosition / 100)
}
.visualEffect { content, proxy in
let yPosition = proxy.frame(in: .global).minY
content
.hueRotation(Angle(degrees: Double(yPosition / 10)))
}
}
}
In this example:
- The
scrollTransition
modifier is used to offset, scale, fade, and blur theItemView
based on its y-position. - The
visualEffect
modifier is used to change the hue of theItemView
based on its y-position.
For more detailed information and examples, you can refer to the session "Create custom visual effects with SwiftUI" at the 00:04:07 timestamp.
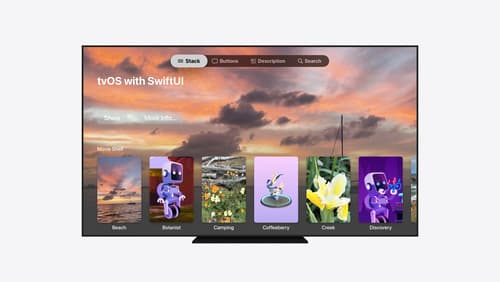
Migrate your TVML app to SwiftUI
SwiftUI helps you build great apps on all Apple platforms and is the preferred toolkit for bringing your content into the living room with tvOS 18. Learn how to use SwiftUI to create familiar layouts and controls from TVMLKit, and get tips and best practices.
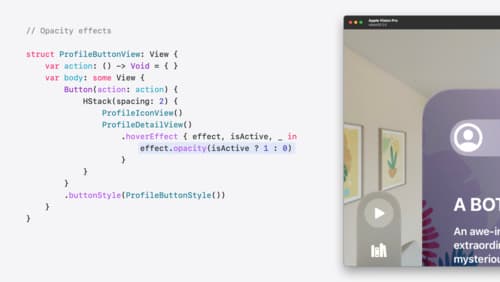
Create custom hover effects in visionOS
Learn how to develop custom hover effects that update views when people look at them. Find out how to build an expanding button effect that combines opacity, scale, and clip effects. Discover best practices for creating effects that are comfortable and respect people’s accessibility needs.
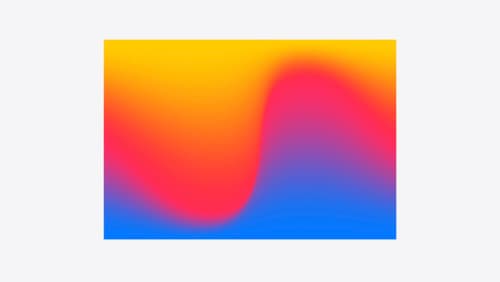
Create custom visual effects with SwiftUI
Discover how to create stunning visual effects in SwiftUI. Learn to build unique scroll effects, rich color treatments, and custom transitions. We’ll also explore advanced graphic effects using Metal shaders and custom text rendering.