my UIViewController currently only support portrait what do i have to change to support landscape in Objective-C
Generated on 8/2/2024
1 search
To support landscape orientation in your UIViewController
in Objective-C, you need to make a few changes to your app's settings and code. Here are the steps:
-
Update the Info.plist: Ensure that your app's
Info.plist
file includes support for landscape orientations. Add the following keys if they are not already present:<key>UISupportedInterfaceOrientations</key> <array> <string>UIInterfaceOrientationPortrait</string> <string>UIInterfaceOrientationLandscapeLeft</string> <string>UIInterfaceOrientationLandscapeRight</string> </array>
-
Override the Supported Interface Orientations in Your View Controller: In your
UIViewController
subclass, override thesupportedInterfaceOrientations
method to specify the orientations your view controller supports:- (UIInterfaceOrientationMask)supportedInterfaceOrientations { return UIInterfaceOrientationMaskPortrait | UIInterfaceOrientationMaskLandscapeLeft | UIInterfaceOrientationMaskLandscapeRight; }
-
Handle Orientation Changes: If you need to handle layout changes when the orientation changes, you can override the
viewWillTransitionToSize:withTransitionCoordinator:
method:- (void)viewWillTransitionToSize:(CGSize)size withTransitionCoordinator:(id<UIViewControllerTransitionCoordinator>)coordinator { [super viewWillTransitionToSize:size withTransitionCoordinator:coordinator]; [coordinator animateAlongsideTransition:^(id<UIViewControllerTransitionCoordinatorContext> context) { // Handle the orientation change [self updateLayoutForNewSize:size]; } completion:nil]; } - (void)updateLayoutForNewSize:(CGSize)size { // Update your layout here }
For more detailed information on handling different device orientations and ensuring your UI adapts correctly, you might find the session What’s new in UIKit helpful, especially the chapter on "Automatic trait tracking" which starts at 06:02.
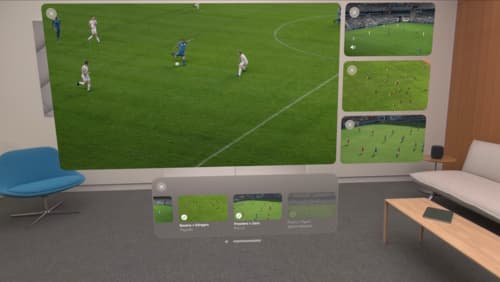
Explore multiview video playback in visionOS
Learn how AVExperienceController can enable playback of multiple videos on Apple Vision Pro. Review best practices for adoption and explore great use cases, like viewing a sports broadcast from different angles or watching multiple games simultaneously. And discover how to design a compelling and intuitive multiview experience in your app.
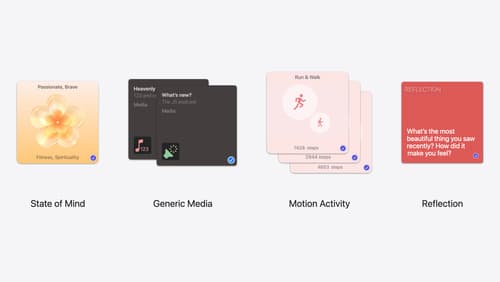
Enhanced suggestions for your journaling app
Find out how your journaling app can display journaling suggestions with richer content from the system. Explore new types of available content like state of mind data, reflection prompts, and support for third-party media content and motion-based activities.
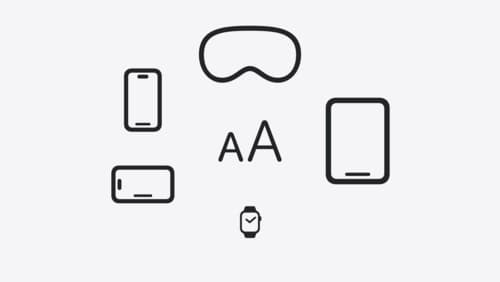
Get started with Dynamic Type
Dynamic Type lets people choose their preferred text size across the system and all of their apps. To help you get started supporting Dynamic Type, we’ll cover the fundamentals: How it works, how to find issues with scaling text in your app, and how to take practical steps using SwiftUI and UIKit to create a great Dynamic Type experience. We’ll also show how you can best use the Large Content Viewer to make navigation controls accessible to everyone.
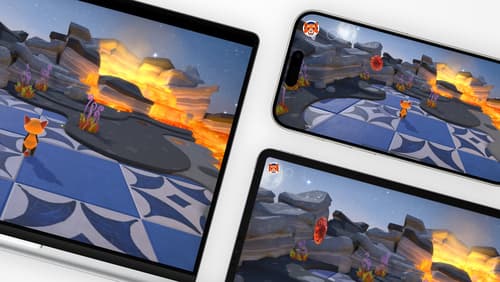
Design advanced games for Apple platforms
Learn how to adapt your high-end game so it feels at home on Mac, iPad, and iPhone. We’ll go over how to make your game look stunning on different displays, tailor your input and controls to be intuitive on each device, and take advantage of Apple technologies that deliver great player experiences.
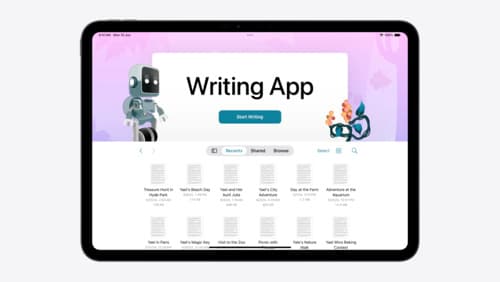
Evolve your document launch experience
Make your document-based app stand out, and bring its unique identity into focus with the new document launch experience. Learn how to leverage the new API to customize the first screen people see when they launch your app. Utilize the new system-provided design, and amend it with custom actions, delightful decorative views, and impressive animations.
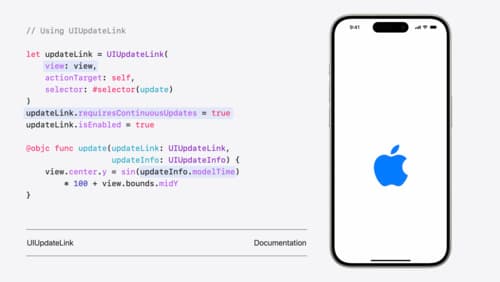
What’s new in UIKit
Explore everything new in UIKit, including tab and document launch experiences, transitions, and text and input changes. We’ll also discuss better-than-ever interoperability between UIKit and SwiftUI animations and gestures, as well as general improvements throughout UIKit.