how to use swift enums
Generated on 8/2/2024
1 search
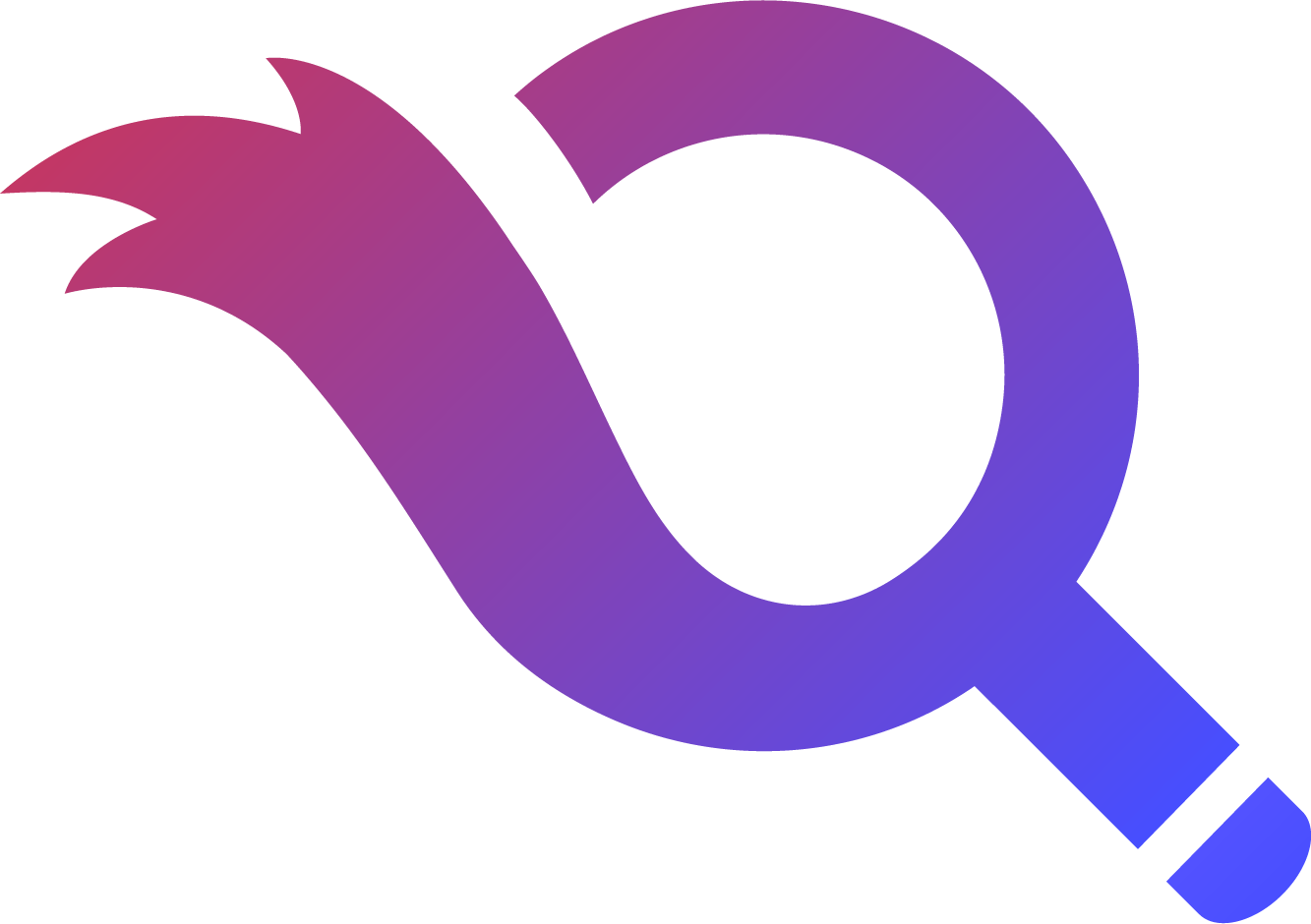
This post is brought to you by Tailscan,
the best devtool for Tailwind CSS.
To use Swift enums effectively, you can follow these guidelines based on the content presented at WWDC:
-
Basic Usage:
- Enums in Swift can represent a choice out of a set of possible values. For example, you can use an enum to represent the state of a light (on or off) or the level of brightness.
- Enums can also have associated values, which allow you to store additional information along with each case. For example, a color control case might have associated values for hue and saturation.
-
Pattern Matching:
- Swift's pattern matching capabilities allow you to match enum cases together with their associated values in a single switch statement, making your code more concise and readable.
- Example:
enum ColorControl { case hueSaturation(hue: Int, saturation: Int) case temperature(Int) } let color = ColorControl.hueSaturation(hue: 120, saturation: 100) switch color { case .hueSaturation(let hue, let saturation): print("Hue: \(hue), Saturation: \(saturation)") case .temperature(let temp): print("Temperature: \(temp)") }
-
Error Handling:
- Enums are also useful for error handling. You can define an enum that conforms to the
Error
protocol to represent different error cases. - Example:
enum NetworkError: Error { case badURL case requestFailed case unknown } func fetchData(from url: String) throws { guard url == "validURL" else { throw NetworkError.badURL } // Fetch data logic } do { try fetchData(from: "invalidURL") } catch NetworkError.badURL { print("Bad URL") } catch { print("Unknown error") }
- Enums are also useful for error handling. You can define an enum that conforms to the
-
Using Enums in Event Handlers:
- Enums can be particularly useful in event handlers where you need to react to different attributes being set. Auto-completion in Swift will help you handle all possible cases.
- Example:
enum LightAttribute { case onOff(Bool) case brightness(Int) case color(ColorControl) } func handleEvent(attribute: LightAttribute) { switch attribute { case .onOff(let isOn): // Handle on/off case .brightness(let level): // Handle brightness case .color(let colorControl): // Handle color control } }
For more detailed examples and explanations, you can refer to the following sessions from WWDC:
- Go small with Embedded Swift
- A Swift Tour: Explore Swift’s features and design
- What’s new in App Intents
Relevant Sessions
- Go small with Embedded Swift
- A Swift Tour: Explore Swift’s features and design
- What’s new in App Intents
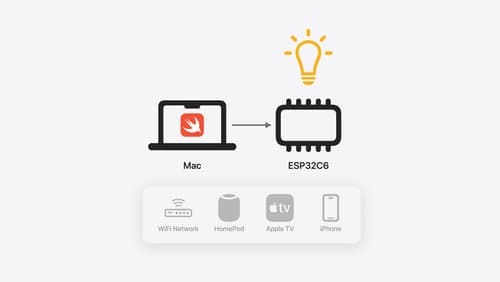
Go small with Embedded Swift
Embedded Swift brings the safety and expressivity of Swift to constrained environments. Explore how Embedded Swift runs on a variety of microcontrollers through a demonstration using an off-the-shelf Matter device. Learn how the Embedded Swift subset packs the benefits of Swift into a tiny footprint with no runtime, and discover plenty of resources to start your own Embedded Swift adventure.
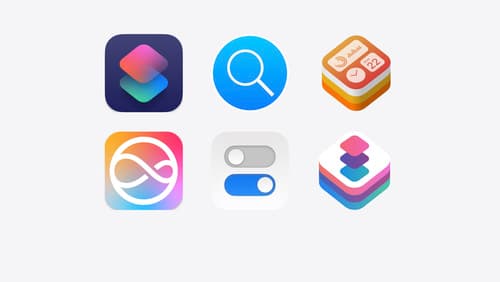
What’s new in App Intents
Learn about improvements and all-new features with App Intents, and discover how this framework can help you expose your app’s functionality to Siri, Spotlight, Shortcuts, and more. We’ll show you how to make your entities more meaningful to the platform with the Transferable API, File Representations, new IntentFile APIs, and Spotlight Indexing, opening up powerful functionality in Siri and the Shortcuts app. Empower your intents to take people deep into your app with URL Representable Entities. Explore new techniques to model your entities and intents with new APIs for error handling and union values.
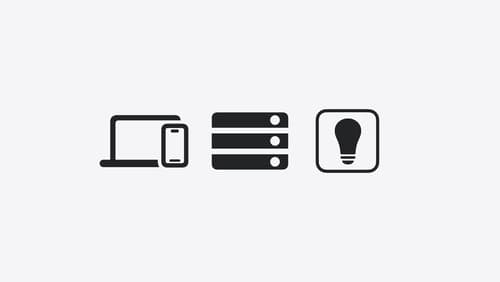
A Swift Tour: Explore Swift’s features and design
Learn the essential features and design philosophy of the Swift programming language. We’ll explore how to model data, handle errors, use protocols, write concurrent code, and more while building up a Swift package that has a library, an HTTP server, and a command line client. Whether you’re just beginning your Swift journey or have been with us from the start, this talk will help you get the most out of the language.
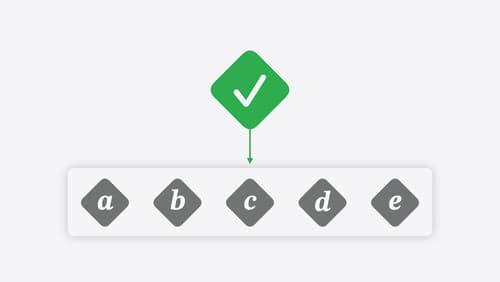
Go further with Swift Testing
Learn how to write a sweet set of (test) suites using Swift Testing’s baked-in features. Discover how to take the building blocks further and use them to help expand tests to cover more scenarios, organize your tests across different suites, and optimize your tests to run in parallel.