How can I unit test a SwiftUI View?
Generated on 8/2/2024
1 search
To unit test a SwiftUI View, you can leverage the new Swift testing framework introduced by Apple. Here are the steps and concepts you need to know:
-
Create a Test Target: If you don't already have a test target in your Xcode project, you can create one by choosing a name for your new target and clicking finish.
-
Import the Testing Module: Start by importing the testing module in your test file.
-
Write a Test Function: Write a global function and add the
@Test
attribute to it. This attribute indicates that the function is a test. Xcode will recognize it and show a run button alongside it. -
Initialize the View: In your test function, initialize the SwiftUI view you want to test.
-
Use Expectations and Assertions: Use the
#expect
macro to verify that the view behaves as expected. You can write simple or complex Swift expressions to check the state of your view. -
Run the Test: You can run the test directly from Xcode by clicking the run button next to the test function.
Here is an example of how you might write a test for a SwiftUI view:
import Testing
import SwiftUI
@testable import YourApp
@Test
func testMySwiftUIView() {
// Initialize the view
let view = MySwiftUIView()
// Use expectations to verify the view's state
#expect(view.someProperty).toEqual(expectedValue)
}
For more detailed information on Swift testing, you can refer to the session Meet Swift Testing.
Relevant Sessions
These sessions cover the new Swift testing framework and how to integrate it with Xcode, providing a comprehensive guide to writing and running tests for your SwiftUI views.
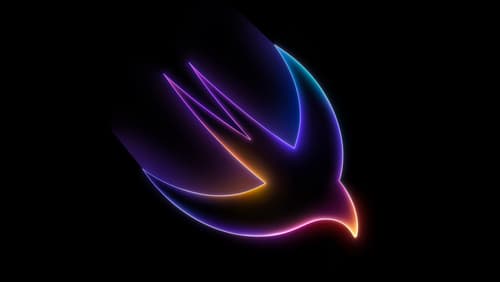
Platforms State of the Union
Discover the newest advancements on Apple platforms.
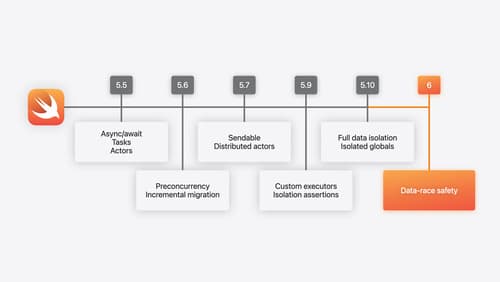
What’s new in Swift
Join us for an update on Swift. We’ll briefly go through a history of Swift over the past decade, and show you how the community has grown through workgroups, expanded the package ecosystem, and increased platform support. We’ll introduce you to a new language mode that achieves data-race safety by default, and a language subset that lets you run Swift on highly constrained systems. We’ll also explore some language updates including noncopyable types, typed throws, and improved C++ interoperability.
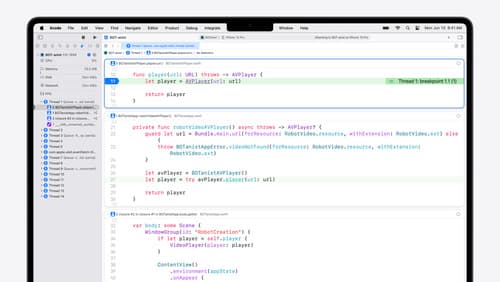
What’s new in Xcode 16
Discover the latest productivity and performance improvements in Xcode 16. Learn about enhancements to code completion, diagnostics, and Xcode Previews. Find out more about updates in builds and explore improvements in debugging and Instruments.
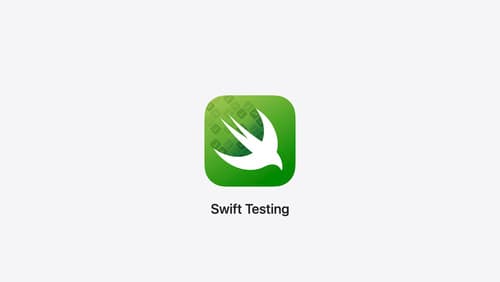
Meet Swift Testing
Introducing Swift Testing: a new package for testing your code using Swift. Explore the building blocks of its powerful new API, discover how it can be applied in common testing workflows, and learn how it relates to XCTest and open source Swift.